Hi, at times you may want to merge multiple json objects into a single entity when working with different APIs. Recently I also found myself in such situation and came across a very simple and effective solution with jQuery. In this tutorial, I'll show you how to merge two json objects into one using jQuery library. jQuery provides several utility functions and the extend() method is one among them. It merges two or more objects together into the first object (i.e., first parameter passed to the function).
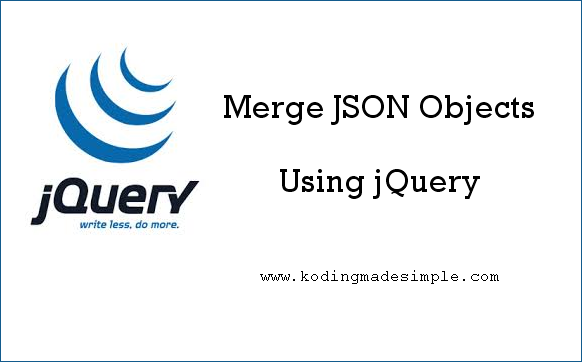
Merging Two JSON Objects using jQuery
In order to merge the json objects we have to use jQuery's extend() function which takes up multiple parameters. Here is the syntax for the method.
jQuery.extend([deep], target, object1 [, objectN])
- Where 'deep' when set as TRUE, makes the merging process recursive (optional).
- 'target' is the object which consists of the merged contents.
- 'object1' is the first object to be merged.
- 'objectN' is the additional objects to be merged.
Both 'deep' & 'target' parameters are optional and if the target object is not provided, then the subsequent object(s) contents will be merged and added to the first given object.
Here is the simple example of merging two json objects in jquery and displaying the result in a <div>
block.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <title>Merge Two JSON Objects in jQuery Example</title> <script src="js/jquery-1.10.2.js"></script> </head> <body> <div id="output"></div> <script> $(document).ready(function() { var obj1 = {"name": "Garrett Davidson", "gender": "male", "dob": "1990/04/20"}; var obj2 = {"position": "System Administrator", "location": "New York"}; $.extend(obj1, obj2); $('#output').text(JSON.stringify(obj1)); }); </script> </body> </html> // produces output: // obj1 // {"name":"Garrett Davidson","gender":"male","dob":"1990/04/20","position":"System Administrator","location":"New York"} // obj2 // {"position": "System Administrator", "location": "New York"}
In the above script, we have two objects, 'obj1' and 'obj2' that contains personal and professional details of an employee.
$.extend(obj1, obj2);
statement merges the contents of obj2
with obj1
as we haven't provided target object. In order to display the merged contents in a readable format, we have used JSON.stringify(obj1);
statement to convert the object into json string.
Alternatively you can also preserve the original objects by passing an empty object as 'target' parameter like this,
<script> $(document).ready(function() { var obj1 = {"name": "Garrett Davidson", "gender": "male", "dob": "1990/04/20"}; var obj2 = {"position": "System Administrator", "location": "New York"}; var obj = $.extend({}, obj1, obj2); $('#output').text(JSON.stringify(obj)); }); </script> // produces output: // obj1 // {"name": "Garrett Davidson", "gender": "male", "dob": "1990/04/20"} // obj2 // {"position": "System Administrator", "location": "New York"} // obj // {"name":"Garrett Davidson","gender":"male","dob":"1990/04/20","position":"System Administrator","location":"New York"}
If you want to just only verify if the object contents are properly merged, then you can log and check it in the browser console like this,
var obj = $.extend({}, obj1, obj2); console.log(obj);
And that was all about merging two json objects into one using jquery.
Recommended Read: How to Read and Parse JSON String in jQuery
Don't Miss: Display JSON in HTML Table using jQuery DataTables Plug-in
No comments:
Post a Comment