Today we'll see a codeigniter tutorial demonstrating user login and registration system. As you know Login and Signup Module (User Management System) forms the base for membership driven websites and applications. I have written about codeingiter login and registration process separately earlier, but got requests from blog readers to provide a complete user management module. So in today's post, I have tried to cover as much as possible in user module comprising home, login, signup and user profile/dashboard page.
So let us see how to create complete codeigniter login and registration system.
Create Database for CI Login & Signup Module:
First create the mysql database required for the codeigniter user management system.
Read Also:- CodeIgniter Two-Step Registration Form with Email Confirmation
- CodeIgniter Pagination Script with Search Filter using Bootstrap
- How to Retrieve Data From Database using CodeIgniter & AJAX
CREATE DATABASE `testdb`; USE `testdb`; CREATE TABLE IF NOT EXISTS `user` ( `id` int(12) NOT NULL AUTO_INCREMENT, `fname` varchar(30) NOT NULL, `lname` varchar(30) NOT NULL, `email` varchar(60) NOT NULL, `password` varchar(40) NOT NULL, PRIMARY KEY (`id`), UNIQUE KEY `email` (`email`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
Run the above the sql commands in mysql db and create the necessary database and table.
Build CodeIgniter Login and Registration System:
To build our codeigniter login and registration system we need a bunch of controller, view and model files. Here are the files we are going to create.
- home.php - This is the Home page controller of the user management application.
- login.php - It's the user login form controller file.
- signup.php - This one is the user signup page(registration) controller.
- profile.php - This is the controller for my profile/dashboard page.
- home_view.php - It is the Home page view file.
- login_view.php - View file for login controller.
- signup_view.php - View file for signup/registration controller.
- profile_view.php – It is the user profile view file.
- user_model.php - This is the model file for the user management module. Here we place all the db transactions of the module.
Codeignitor User Login and Registration System:
In case you don't know, below are the steps breakdown for what we are going to build in this user login/signup system in code igniter.
- The user enters the Home page which contains links for Login and Signup on top navigation menu.
- As for New Users » user click on Signup link » user redirected to signup/registration form » user fills up the form details and submit » process user details and store it into database » notify user.
- As for Registered Users » user click on the Login link on Home page » user redirected to login form » user enter login credentials » authenticate user and redirect to User Profile page.
- For Singed-in users » Show User's name and Logout link on top menu bar.
- When user clicks on Logout » destroy session and redirect to Home page.
Note: As for designing user interface, I have used the readily available Twitter Bootstrap CSS as it's easier this way and mobile responsive in nature. Check here to know about using bootstrap with codeigniter framework.
Home.php
This is controller for the Home/Main page of the user management module and it shows Login and Signup link at the top menu for guests and User's Name and Logout link for signed-in users.
<?php class home extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('url', 'html')); $this->load->library('session'); } function index() { $this->load->view('home_view'); } function logout() { // destroy session $data = array('login' => '', 'uname' => '', 'uid' => ''); $this->session->unset_userdata($data); $this->session->sess_destroy(); redirect('home/index'); } } ?>
Signup.php
This is the controller file for the codeigniter user registration form. It collects first name, last name, email id and password from the user and validates the input and stores it into database.
<?php class signup extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form','url')); $this->load->library(array('session', 'form_validation')); $this->load->database(); $this->load->model('user_model'); } function index() { // set form validation rules $this->form_validation->set_rules('fname', 'First Name', 'trim|required|alpha|min_length[3]|max_length[30]|xss_clean'); $this->form_validation->set_rules('lname', 'Last Name', 'trim|required|alpha|min_length[3]|max_length[30]|xss_clean'); $this->form_validation->set_rules('email', 'Email ID', 'trim|required|valid_email|is_unique[user.email]'); $this->form_validation->set_rules('password', 'Password', 'trim|required|matches[cpassword]|md5'); $this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required'); // submit if ($this->form_validation->run() == FALSE) { // fails $this->load->view('signup_view'); } else { //insert user details into db $data = array( 'fname' => $this->input->post('fname'), 'lname' => $this->input->post('lname'), 'email' => $this->input->post('email'), 'password' => $this->input->post('password') ); if ($this->user_model->insert_user($data)) { $this->session->set_flashdata('msg','<div class="alert alert-success text-center">You are Successfully Registered! Please login to access your Profile!</div>'); redirect('signup/index'); } else { // error $this->session->set_flashdata('msg','<div class="alert alert-danger text-center">Oops! Error. Please try again later!!!</div>'); redirect('signup/index'); } } } } ?>
This is how the signup form looks like,
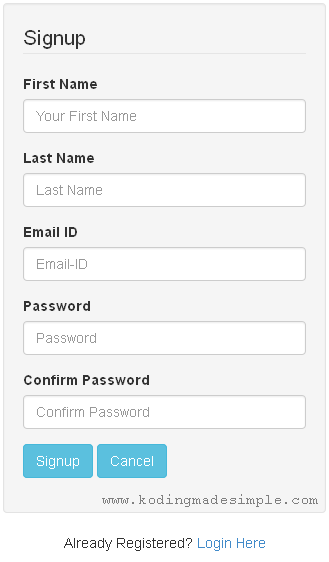
All fields are mandatory and once the user submits the form the input will be validated against a set of form validation rules. If the validation fails, user will be prompted to enter right data by showing the error message like this.
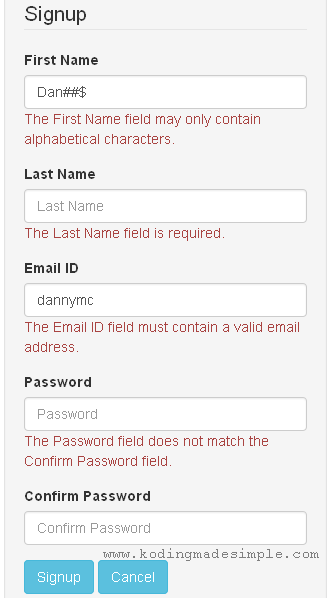
If validation succeeds, registration process will be completed and notified to the user.
Login.php
This is the controller file for user login process. It asks for user's email address and password and check if the provided login credentials are correct or not.
<?php class login extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form','url','html')); $this->load->library(array('session', 'form_validation')); $this->load->database(); $this->load->model('user_model'); } function index() { // get form input $email = $this->input->post("email"); $password = $this->input->post("password"); // form validation $this->form_validation->set_rules("email", "Email-ID", "trim|required|xss_clean"); $this->form_validation->set_rules("password", "Password", "trim|required|xss_clean"); if ($this->form_validation->run() == FALSE) { // validation fail $this->load->view('login_view'); } else { // check for user credentials $uresult = $this->user_model->get_user($email, $password); if (count($uresult) > 0) { // set session $sess_data = array('login' => TRUE, 'uname' => $uresult[0]->fname, 'uid' => $uresult[0]->id); $this->session->set_userdata($sess_data); redirect("profile/index"); } else { $this->session->set_flashdata('msg', '<div class="alert alert-danger text-center">Wrong Email-ID or Password!</div>'); redirect('login/index'); } } } } ?>
It produces a login form like this,
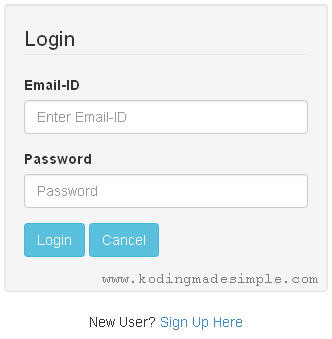
If login failed, the user will be shown an error message like this,
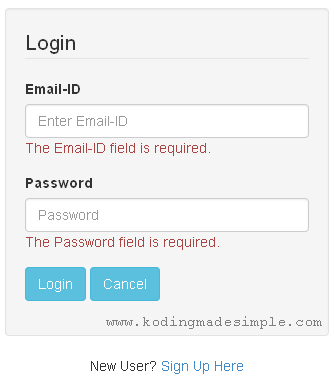
If user credentials are valid, then it will be stored in session and user will be redirected to profile/dashboard page.
Profile.php
This is the controller for the User Profile page. It lists user profile summary and other details.
<?php class profile extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('url','html')); $this->load->library('session'); $this->load->database(); $this->load->model('user_model'); } function index() { $details = $this->user_model->get_user_by_id($this->session->userdata('uid')); $data['uname'] = $details[0]->fname . " " . $details[0]->lname; $data['uemail'] = $details[0]->email; $this->load->view('profile_view', $data); } } ?>
That's it. These are all the important controller files required for the code igniter user registration and login system. Now run the app and go to Home page.
![]() |
CodeIgniter Login and Registration System - Home Page |
Click on Signup link and it will take you to the user signup form.
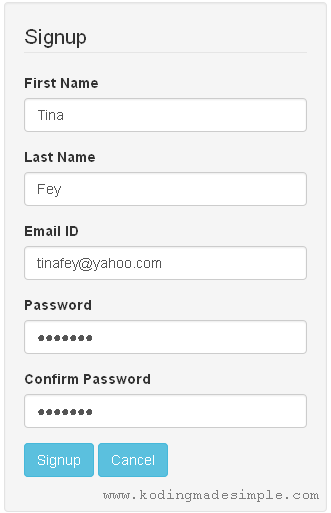
Now enter the details and submit the form. If things gone right, you will be shown with a success message like this,
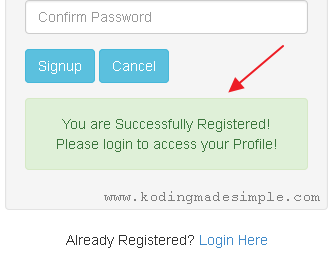
Now click on the Login link to go to the login page.
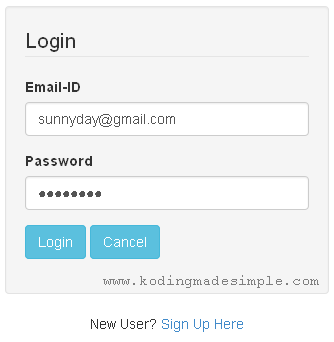
Provide the email and password which you have registered earlier. Now you will be redirected to your Profile page.
![]() |
CodeIgniter Login and Registration Module - User Profile Page |
To sign-out the session, click on Logout link at the top navigation menu which will take you back to Home page.
CodeIgniter Login and Registration Source Code:
Read Also:- CodeIgniter Basic Database CRUD Operations for Beginners
- Dynamic Dependent Select Box using CodeIgniter, AJAX & jQuery
- CodeIgniter Modal Contact Form using AJAX and jQuery
- CodeIgniter Tutorials for Beginners - The Ultimate Guide for CI
Well! Now we have created user login and registration system in codeignator. I hope you have enjoyed this codeigniter login and registration tutorial. Please let me know your queries through comments :)
thanks a lot for this tutorial
ReplyDeleteWelcome!
Deletemine doesnt work help plzzz
DeleteWhen I logged in, it shows "Wrong Email-ID or Password"
ReplyDeletehelp!
same problem im facing
Deletebut sessions not destroyed here after logout.when i back after logout page is redirect to home page
ReplyDeletehttp://127.0.0.1/ci%20login%20and%20registration/home/
ReplyDelete403 error show. pls help me. i don't understand which path gave in url
May I ask why the use of "ENGINE=MyISAM" and not "ENGINE=InnoDB"? I was under the impression that MyISAM is for version before mySQL 5.5.
ReplyDeleteEmail-ID
ReplyDeleteUnable to access an error message corresponding to your field name Email-ID.(xss_clean)
Password
Unable to access an error message corresponding to your field name Password.(xss_clean)
help sir
ReplyDeleteEmail-ID
Unable to access an error message corresponding to your field name Email-ID.(xss_clean)
Password
Unable to access an error message corresponding to your field name Password.(xss_clean)
load the helper('security') to fix your problem
Deleteload the helper('security') to fix your problem
DeleteHi Rodel, here is the solution that worked for me. Just remove xss_clean from your validation.. reason being, xss_clean is no longer part of form validation in codeigniter 3
Deletei place asset folder application inside but css and js not working, how can i do ?help me beacuse i am new in codeigniter
ReplyDeleteThank you
and if had different sessions
ReplyDeletelogin and signup links doesnt work. I keep getting 404. I notice we you use multiple controller so I write the routes.php like this:
ReplyDelete$route['default_controller'] = 'home';
$route['signup'] = 'signup';
$route['login'] = 'login';
$route['profile'] = 'profile';
But it still not working. Can you tell me what should I do to fix this?
yeah me too i think new ci cant work well this source ?
ReplyDeleteas I make a validation for multi users
ReplyDeletehow can i navigate the home page?
ReplyDeletePlease I'm having problem here, home page does not display as yours, can we communicate through eamil please? well my email is munirmdee@gmail.com
ReplyDeletegetting error during form validation as "The Password field does not match the Confirm Password field." Any help is much appreciated. Thanks.
ReplyDeleteThe below has solved my problem by update in singup.php in controllers.
ReplyDelete$this->form_validation->set_rules('password', 'Password', 'trim|required|md5');
$this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required|md5|matches[password]');
This sorted it for me. Many thank Pavan.
Deletedoesnt work, can you help plz???
ReplyDeletedoesnt work, can you help plzz???
ReplyDeleteWhat's not working? Showing error?
Deleteits too easy
Delete(xss_clean) ==> load the helper('security') to fix your problem
--------------------
"The Password field does not match the Confirm Password field."
replace
---------
$this->form_validation->set_rules('password', 'Password', 'trim|required|matches[cpassword]|md5');
$this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required');
----------
par
----------
$this->form_validation->set_rules('password', 'Password', 'trim|required|md5');
$this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required|md5|matches[password]');
hello i need help
ReplyDeleteneed help
ReplyDeletedoesnt work
ReplyDeleteCan you please be more precise? do you get error? on which part?
Deletecan you please send on my email would e great
ReplyDeleteHow to make multi user login with this?
ReplyDeleteUnable to load the requested class: Session
ReplyDeletehow to slove this
login is not working | showing login form but after clicking on login buton showing message "Wrong Email-ID or Password!" even email and pwd are correct in database.. Help admin
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteunable to login showing message "Wrong Email-ID or Password!" event coreeect in database
ReplyDeletethe code dont work :(
ReplyDeletehome page and profile was not working properly
ReplyDeleteFatal error: Class 'CI_Controller' not found in C:\xampp\htdocs\CI\controller\home.php on line 2
ReplyDeletewhat solution for me?
Hi. Firstly thanks for the article - just what I was looking for as an introduction to CI and to help me develop a simple registration process.
ReplyDeleteI have the home page wired up and working, but the login page is returning an error.
Fatal error: Call to undefined function mysqli_init() in /home/vpsadmin/public_html/igniter/system/database/drivers/mysqli/mysqli_driver.php on line 135
I have the default group database set up and I'm using the mysqli db driver in my database config.
Anyone seen this issue? I would be very grateful for some advice on this.
Many thanks.
I just noticed that you always load you helpers in php. Why not use the cofig? what the difference by then?
ReplyDeleteJust for tutorial purpose and better understanding. In real time, you must take advantage of autoloading helpers and libraries at start. It is efficient & minimizes server load.
DeleteThat's what I thought. I almost read all the tutorials just like this one and I'm comparing each codes. I found yours more precise. I also want to include avatar on each users and also their level can you upgrade your snippet?Sorry for asking to much I'm just starting to code using codeigniter. Best regards.
Deletekindly help me i got wrong email id or wrong password after login
ReplyDeletehi, i got wrong email and wrong password error kindly help me
ReplyDeleteHey this code works! Please make sure you have the login details in mysql db.
Deleteyes its working now
DeleteValli Pandy kindly can u tell me how can i create add user,group and permission on dashboard??
DeleteValli Pandy, BTW your validation "xss_clean" I read at the stackoverflow is not necessary anymore. I think you must separate that kind of validation.
ReplyDeleteValli Pandy, BTW your validation "xss_clean" is no longer necessary I think you should separate that additional validation.
ReplyDeleteThanks for tutorial, but how do I create random numeric to Id instead of Auto_Increment?
ReplyDeleteI move the code to a Github repo to help improve the system and provide better documentation going forward.
ReplyDeletehttps://github.com/gegere/Codeigniter-Login-and-Registration
Welcome:)
Deletenothing to say bro,just awesome
ReplyDeleteparticulary your discussion is awesome,clear logic,thanks again
ReplyDeleteNice tutorial its working for me .
ReplyDelete