Hi! In this tutorial let me show you about upload, view and download file in php and mysql. The file uploading process is similar to what we have discussed here, but this php script not only uploads file to the server but also stores the file path and its created date in mysql database. Apart from uploading file, it also gives you the option to view file on browser and download it from server.
With PHP you can practically upload any type of files and the file uploading script I have shared below will work for all file types like PDF, Document, Images, MP3, Videos, Zip archives etc. Just include those file extensions in the filtering process ($allowed
array) and you will be able to upload them.
How to Upload, View & Download File in PHP & MySQL?
Let's move on to the coding part. First you should create mysql database to store file details.
Create MySQL Database:
CREATE DATABASE `demo` ; Use `demo`; CREATE TABLE IF NOT EXISTS `tbl_files` ( `id` int(9) NOT NULL AUTO_INCREMENT, `filename` varchar(255) NOT NULL, `created` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1;
Next is the database connectivity script that establishes connection to mysql database from php.
dbconnect.php
<?php //connect mysql database $host = "localhost"; $user = "username"; $pass = "password"; $db = "demo"; $con = mysqli_connect($host, $user, $pass, $db) or die("Error " . mysqli_error($con)); ?>
Then create index.php - this is the main file containing user interface. It has an upload form and a html table to display the list of uploaded files from database along with 'View' & 'Download' links for them.
index.php
<?php include_once 'dbconnect.php'; // fetch files $sql = "select filename from tbl_files"; $result = mysqli_query($con, $sql); ?> <!DOCTYPE html> <html> <head> <title>Upload View & Download file in PHP and MySQL | Demo</title> <meta content="width=device-width, initial-scale=1.0" name="viewport" > <link rel="stylesheet" href="css/bootstrap.css" type="text/css" /> </head> <body> <br/> <div class="container"> <div class="row"> <div class="col-xs-8 col-xs-offset-2 well"> <form action="upload.php" method="post" enctype="multipart/form-data"> <legend>Select File to Upload:</legend> <div class="form-group"> <input type="file" name="file1" /> </div> <div class="form-group"> <input type="submit" name="submit" value="Upload" class="btn btn-info"/> </div> <?php if(isset($_GET['st'])) { ?> <div class="alert alert-danger text-center"> <?php if ($_GET['st'] == 'success') { echo "File Uploaded Successfully!"; } else { echo 'Invalid File Extension!'; } ?> </div> <?php } ?> </form> </div> </div> <div class="row"> <div class="col-xs-8 col-xs-offset-2"> <table class="table table-striped table-hover"> <thead> <tr> <th>#</th> <th>File Name</th> <th>View</th> <th>Download</th> </tr> </thead> <tbody> <?php $i = 1; while($row = mysqli_fetch_array($result)) { ?> <tr> <td><?php echo $i++; ?></td> <td><?php echo $row['filename']; ?></td> <td><a href="uploads/<?php echo $row['filename']; ?>" target="_blank">View</a></td> <td><a href="uploads/<?php echo $row['filename']; ?>" download>Download</td> </tr> <?php } ?> </tbody> </table> </div> </div> </div> </body> </html>
Note: This demo uses twitter bootstrap for css stylesheet.
Running index.php will generate a page with upload form and table with files details similar to this. Users can either click on 'View' link to view the files on browser or on 'Download' to download the files from server.
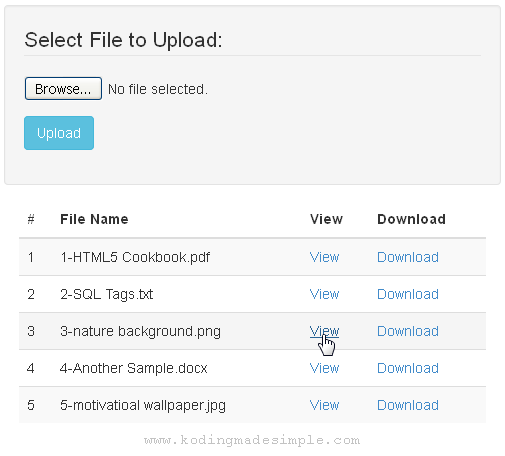
Finally there is 'uploads.php' file which will be executed when the form is submitted to upload the selected file. Here is where we actually upload the file to the server from the client machine and save its name and uploaded date into the database.
uploads.php
<?php //check if form is submitted if (isset($_POST['submit'])) { $filename = $_FILES['file1']['name']; //upload file if($filename != '') { $ext = pathinfo($filename, PATHINFO_EXTENSION); $allowed = ['pdf', 'txt', 'doc', 'docx', 'png', 'jpg', 'jpeg', 'gif']; //check if file type is valid if (in_array($ext, $allowed)) { // get last record id $sql = 'select max(id) as id from tbl_files'; $result = mysqli_query($con, $sql); if (count($result) > 0) { $row = mysqli_fetch_array($result); $filename = ($row['id']+1) . '-' . $filename; } else $filename = '1' . '-' . $filename; //set target directory $path = 'uploads/'; $created = @date('Y-m-d H:i:s'); move_uploaded_file($_FILES['file1']['tmp_name'],($path . $filename)); // insert file details into database $sql = "INSERT INTO tbl_files(filename, created) VALUES('$filename', '$created')"; mysqli_query($con, $sql); header("Location: index.php?st=success"); } else { header("Location: index.php?st=error"); } } else header("Location: index.php"); } ?>
This script upload file from local machine to server and stores its details into database and redirects to index.php. If everything goes right you will be able to see success message on completion.
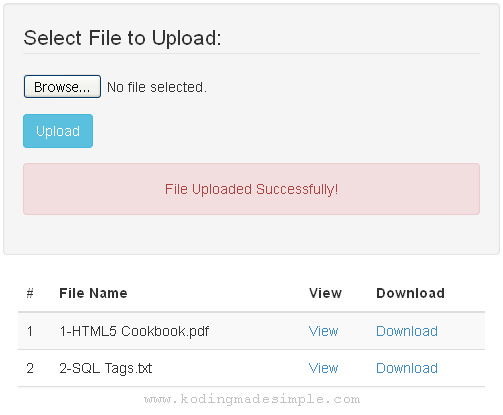
If there's any error you will be notified about it.
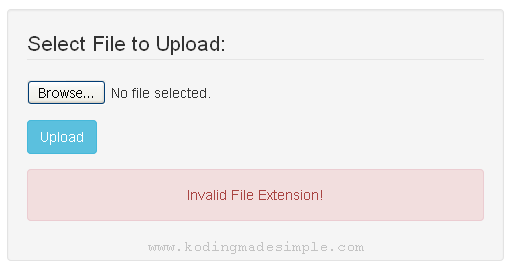
So we have seen about file upload and to view and download them using php and mysql database. If you want you can set the file size limit or restrict users to upload only pdf or images etc.
Parse error: syntax error, unexpected '[' in C:\xampp\htdocs\upload a data\upload.php on line 11
ReplyDeletehelp me to solve this
Very helpfull, thanks.
ReplyDeletecode doesn't work!
ReplyDeleteNo file are inserted into the database.
just able to show success, or invalid file extension notice
It won't store file in db. Only reference to the file path and file creation time. File will be uploaded to 'uploads' directory on the server. You need to create 'uploads' folder in your application root before running the script.
DeleteHope this helps.
Cheers.
you need to include connection file in uploads.php
Deletethank you so much this code very helpful for me
ReplyDeleteGlad you liked it!
DeleteHOW can i use this code in codeigniter?
ReplyDeleteHOW can i use this code in codeigniter?
ReplyDeleteWell! Here are the two ci tutorials you can try with. First one deals with displaying db data in a html table and the other one for uploading files in ci. You have to mix the logic.
Deletehttp://www.kodingmadesimple.com/2016/08/codeigniter-display-query-result-in-view.html
http://www.kodingmadesimple.com/2015/02/image-file-upload-in-codeigniter-validations-examples.html
Saves well to the folder but doesn't show the files on index page
ReplyDeletePlease make sure the file name is stored in db. The data is pulled off from the database and displayed.
Deletewhy downloaded files r empty?
ReplyDeleteit show me " Warning: mysqli_fetch_array() expects parameter 1 to be mysqli_result, boolean given in C:\wamp64\www\FTP\index.php on line 61 ". How can i solve it?
ReplyDeletefetch() method or read here https://www.w3schools.com/php/func_mysqli_fetch_array.asp
Deletehello. How can i modify the code to upload and download mp3 and mp4 files?
ReplyDeleteHi. This code was really great and helpful. But I have a problem. I cant see my uploaded files. I can only upload files. Thanks
ReplyDeletehow to solve that please help me
ReplyDeleteWarning: mysqli_fetch_array() expects parameter 1 to be mysqli_result, boolean given in C:\xampp\htdocs\System\admin\index.php on line 58
file not move to folder only getting file uploaded file success showing when i view and download file not showing
ReplyDeletewhen i upload the file successfully uploaded but when i view and download file are not view and download particular file
ReplyDeletechange uploads.php to upload.php
ReplyDeleteAdd include 'dbconnect.php'; to upload.php
change uploads.php to upload.php
ReplyDeleteadd include 'dbconnect.php'; to upload.php
im too facing the same problem , can anyone help
ReplyDeleteDear Pandey, The upload.php actually does not inserts any data in database. As a result files are being uploaded into UPLOADS folder, but does not show in files list as it pulls data from the database. You have to fix the upload.php
ReplyDeletehye valley
ReplyDeleteI have a problem which it said
Warning: mysqli_connect(): (HY000/1045): Access denied for user 'username'@'localhost' (using password: YES) in C:\xampp\htdocs\naster\dbconnect.php on line 7
Warning: mysqli_error() expects parameter 1 to be mysqli, boolean given in C:\xampp\htdocs\naster\dbconnect.php on line 7
Error
you need to change username into 'root'
DeleteWhat about the view and download??
ReplyDeleteVery helpful! ty..But how to delete those upload files using button? Any helpful code I can use?
ReplyDeletecreate a button and input a sql codes
DeleteI can't view the download?
ReplyDeletethankyou code work fine
ReplyDeletenice project thanks
ReplyDeletewebsite development patna
Files are successfully uploaded on directory path given but how i want to view and download it?
ReplyDelete