PHP Login and Registration Script: User Login/registration system is one of the key components of any membership driven websites and the ability to build them is a must have skill for any web developer. In this post, let us see how to create a complete login and registration system using php and mysql database.
This tutorial is fairly simple and easy to understand, but it covers as much as required to build advanced login/registration system - right from data validation to maintaining php sessions for authenticated access.
MySQL Database for Login/Registration Module
Before entering into the coding part, first let us build the database required for this example.
Read Also:- Country State City Dropdown List using jQuery, Ajax & PHP
- Simple AJAX Pagination Script in jQuery, PHP PDO & MySQL
- File Upload, View and Download using PHP and MySQL
CREATE DATABASE `testdb`; USE `testdb`; CREATE TABLE IF NOT EXISTS `users` ( `id` int(8) NOT NULL AUTO_INCREMENT, `name` varchar(30) NOT NULL, `email` varchar(60) NOT NULL, `password` varchar(40) NOT NULL, PRIMARY KEY (`id`), UNIQUE KEY `email` (`email`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
Run this sql file in phpmyadmin interface and create the database and table.
PHP Script for Login and Registration System
To build the login/registration system we need to create the following files.
- index.php - This is the home page of our application
- register.php - It contains the user sign up form
- login.php - It contains the login form
- logout.php - This contains the user logout script
- dbconnect.php - MySQL database connection script
System Flow for User Login Registration Module
These are the steps breakdown of the user registration/login module we are going to build.
- The Home page (index.php) contains links for login and sign up process in top navigation menu.
- For new users » click on sigup link » user redirected to registration form » fill up the form and submit » validate the form input » registration succeed » notify user and redirect to login page.
- For registered users » click on login link on index.php » user redirected to login page » user provide login credentials » authenticate user and redirect to index page.
- For signed in users » show user's name and logout link on navigation bar.
- User clicks on logout » delete user details from session variable and destroy session » redirect to Home page.
Note: This tutorial uses twitter bootstrap framework for css. I prefer to use this as it's readily available and you can easily integrate bootstrap with HTML. But if you prefer custom style sheet then you can simply attach one and replace all the class selectors used in the html tags with your own. However the program functionality remains the same and will not be disturbed.
Dbconnect.php
This is where we connect to the mysql database. Keeping database connectivity in a separate file is very handy. This way you can get access to database across multiple files by simply including the file once and access it anywhere.
<?php //connect to mysql database $con = mysqli_connect("localhost", "myusername", "mypassword", "testdb") or die("Error " . mysqli_error($con)); ?>
Register.php
This php file contains the code for the user registration process. The access to this page will be restricted for signed in user. We do this by checking out the session variable.
<?php session_start(); if(isset($_SESSION['usr_id'])) { header("Location: index.php"); } include_once 'dbconnect.php'; //set validation error flag as false $error = false; //check if form is submitted if (isset($_POST['signup'])) { $name = mysqli_real_escape_string($con, $_POST['name']); $email = mysqli_real_escape_string($con, $_POST['email']); $password = mysqli_real_escape_string($con, $_POST['password']); $cpassword = mysqli_real_escape_string($con, $_POST['cpassword']); //name can contain only alpha characters and space if (!preg_match("/^[a-zA-Z ]+$/",$name)) { $error = true; $name_error = "Name must contain only alphabets and space"; } if(!filter_var($email,FILTER_VALIDATE_EMAIL)) { $error = true; $email_error = "Please Enter Valid Email ID"; } if(strlen($password) < 6) { $error = true; $password_error = "Password must be minimum of 6 characters"; } if($password != $cpassword) { $error = true; $cpassword_error = "Password and Confirm Password doesn't match"; } if (!$error) { if(mysqli_query($con, "INSERT INTO users(name,email,password) VALUES('" . $name . "', '" . $email . "', '" . md5($password) . "')")) { $successmsg = "Successfully Registered! <a href='login.php'>Click here to Login</a>"; } else { $errormsg = "Error in registering...Please try again later!"; } } } ?> <!DOCTYPE html> <html> <head> <title>User Registration Script</title> <meta content="width=device-width, initial-scale=1.0" name="viewport" > <link rel="stylesheet" href="css/bootstrap.min.css" type="text/css" /> </head> <body> <nav class="navbar navbar-default" role="navigation"> <div class="container-fluid"> <!-- add header --> <div class="navbar-header"> <button type="button" class="navbar-toggle" data-toggle="collapse" data-target="#navbar1"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand" href="index.php">Koding Made Simple</a> </div> <!-- menu items --> <div class="collapse navbar-collapse" id="navbar1"> <ul class="nav navbar-nav navbar-right"> <li><a href="login.php">Login</a></li> <li class="active"><a href="register.php">Sign Up</a></li> </ul> </div> </div> </nav> <div class="container"> <div class="row"> <div class="col-md-4 col-md-offset-4 well"> <form role="form" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" name="signupform"> <fieldset> <legend>Sign Up</legend> <div class="form-group"> <label for="name">Name</label> <input type="text" name="name" placeholder="Enter Full Name" required value="<?php if($error) echo $name; ?>" class="form-control" /> <span class="text-danger"><?php if (isset($name_error)) echo $name_error; ?></span> </div> <div class="form-group"> <label for="name">Email</label> <input type="text" name="email" placeholder="Email" required value="<?php if($error) echo $email; ?>" class="form-control" /> <span class="text-danger"><?php if (isset($email_error)) echo $email_error; ?></span> </div> <div class="form-group"> <label for="name">Password</label> <input type="password" name="password" placeholder="Password" required class="form-control" /> <span class="text-danger"><?php if (isset($password_error)) echo $password_error; ?></span> </div> <div class="form-group"> <label for="name">Confirm Password</label> <input type="password" name="cpassword" placeholder="Confirm Password" required class="form-control" /> <span class="text-danger"><?php if (isset($cpassword_error)) echo $cpassword_error; ?></span> </div> <div class="form-group"> <input type="submit" name="signup" value="Sign Up" class="btn btn-primary" /> </div> </fieldset> </form> <span class="text-success"><?php if (isset($successmsg)) { echo $successmsg; } ?></span> <span class="text-danger"><?php if (isset($errormsg)) { echo $errormsg; } ?></span> </div> </div> <div class="row"> <div class="col-md-4 col-md-offset-4 text-center"> Already Registered? <a href="login.php">Login Here</a> </div> </div> </div> <script src="js/jquery-1.10.2.js"></script> <script src="js/bootstrap.min.js"></script> </body> </html>
Read: How to Use Google reCAPTCHA in PHP Form
This is how the registration form looks like.

Registration form requires user to fill up name, email id and password and confirm password fields. All the fields are mandatory and user will be asked to provide the data if it is left blank like this.

Once the user fills out the details and submits the form for sign up, we sanitize the received data to avoid sql injection and validate the form input. If validation fails the user will be shown with the appropriate error message below each form input like this.

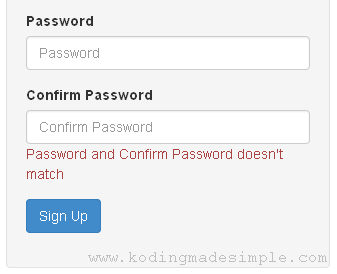
If the validation succeeds the data will be stored in the database and user will be shown with the login link.
Login.php
This file contains the php code for user login process. The access to login form will be restricted for signed in users by checking if the php session is set or not.
<?php session_start(); if(isset($_SESSION['usr_id'])!="") { header("Location: index.php"); } include_once 'dbconnect.php'; //check if form is submitted if (isset($_POST['login'])) { $email = mysqli_real_escape_string($con, $_POST['email']); $password = mysqli_real_escape_string($con, $_POST['password']); $result = mysqli_query($con, "SELECT * FROM users WHERE email = '" . $email. "' and password = '" . md5($password) . "'"); if ($row = mysqli_fetch_array($result)) { $_SESSION['usr_id'] = $row['id']; $_SESSION['usr_name'] = $row['name']; header("Location: index.php"); } else { $errormsg = "Incorrect Email or Password!!!"; } } ?> <!DOCTYPE html> <html> <head> <title>PHP Login Script</title> <meta content="width=device-width, initial-scale=1.0" name="viewport" > <link rel="stylesheet" href="css/bootstrap.min.css" type="text/css" /> </head> <body> <nav class="navbar navbar-default" role="navigation"> <div class="container-fluid"> <!-- add header --> <div class="navbar-header"> <button type="button" class="navbar-toggle" data-toggle="collapse" data-target="#navbar1"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand" href="index.php">Koding Made Simple</a> </div> <!-- menu items --> <div class="collapse navbar-collapse" id="navbar1"> <ul class="nav navbar-nav navbar-right"> <li class="active"><a href="login.php">Login</a></li> <li><a href="register.php">Sign Up</a></li> </ul> </div> </div> </nav> <div class="container"> <div class="row"> <div class="col-md-4 col-md-offset-4 well"> <form role="form" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" name="loginform"> <fieldset> <legend>Login</legend> <div class="form-group"> <label for="name">Email</label> <input type="text" name="email" placeholder="Your Email" required class="form-control" /> </div> <div class="form-group"> <label for="name">Password</label> <input type="password" name="password" placeholder="Your Password" required class="form-control" /> </div> <div class="form-group"> <input type="submit" name="login" value="Login" class="btn btn-primary" /> </div> </fieldset> </form> <span class="text-danger"><?php if (isset($errormsg)) { echo $errormsg; } ?></span> </div> </div> <div class="row"> <div class="col-md-4 col-md-offset-4 text-center"> New User? <a href="register.php">Sign Up Here</a> </div> </div> </div> <script src="js/jquery-1.10.2.js"></script> <script src="js/bootstrap.min.js"></script> </body> </html>
This file produces a login form similar to this.

Login form requires users to provide login credentials (email & password). Both the input fields are mandatory and notify user to fill out if left blank.
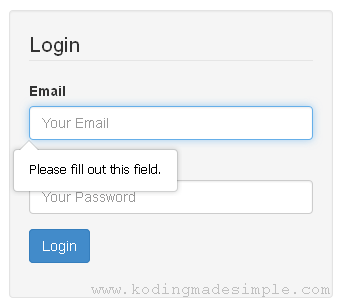
Here too we sanitize the data and check for user authentication against database records. If it fails, we notify the user with error message like this,

If the user credentials are legitimate then we create session and store user's id and name in the session variable and redirect to index.php.
Read: How to Upload & Watermark Images with PHP
Index.php
This is the home page of our application and shows login and sign up menu links for visitors and for signed in members will show their name and logout link respectively.
<?php session_start(); include_once 'dbconnect.php'; ?> <!DOCTYPE html> <html> <head> <title>Home | Koding Made Simple</title> <meta content="width=device-width, initial-scale=1.0" name="viewport" > <link rel="stylesheet" href="css/bootstrap.min.css" type="text/css" /> </head> <body> <nav class="navbar navbar-default" role="navigation"> <div class="container-fluid"> <div class="navbar-header"> <button type="button" class="navbar-toggle" data-toggle="collapse" data-target="#navbar1"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand" href="index.php">Koding Made Simple</a> </div> <div class="collapse navbar-collapse" id="navbar1"> <ul class="nav navbar-nav navbar-right"> <?php if (isset($_SESSION['usr_id'])) { ?> <li><p class="navbar-text">Signed in as <?php echo $_SESSION['usr_name']; ?></p></li> <li><a href="logout.php">Log Out</a></li> <?php } else { ?> <li><a href="login.php">Login</a></li> <li><a href="register.php">Sign Up</a></li> <?php } ?> </ul> </div> </div> </nav> <script src="js/jquery-1.10.2.js"></script> <script src="js/bootstrap.min.js"></script> </body> </html>
Logout.php
This file will be fired up when the user clicks on the logout link. The user logout process is fairly simple. It destroy session and unset the user data from the session.
<?php session_start(); if(isset($_SESSION['usr_id'])) { session_destroy(); unset($_SESSION['usr_id']); unset($_SESSION['usr_name']); header("Location: index.php"); } else { header("Location: index.php"); } ?>
Once you created all the above files, run the app and you will see the home page like this,
![]() |
index.php - Home Page |
Now click on the sign up link and you will be redirected to the user registration form,

Now enter the form details and submit the sign up button. If everything goes right then you will be registered and notified with a message like this,
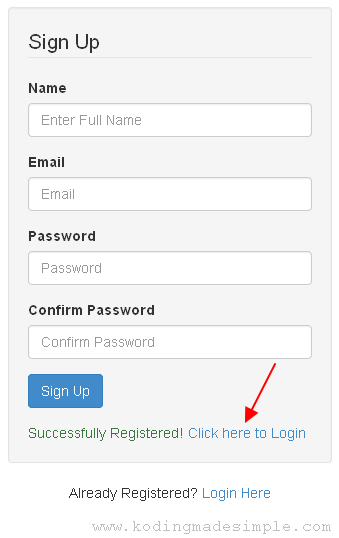
Now go to the login form through the login link.

Provide the login credentials and you will be signed in and redirected to the index page.
![]() |
index.php as signed-in user |
Click on logout to sign out the session and you will be taken back to the index page again.
![]() |
After Log out |
That's it. You have successfully created user login and registration system in php and mysql.
Read Also:- AJAX Modal Login Page using PHP OOP, MySQL and jQuery
- CRUD [Create, Read, Update, Delete] in PHP, MySQL & jQuery
- Dynamic Image Gallery from Folder in PHP, JQuery & Bootstrap
I hope you find this php login/registration script useful. Meet you in another interesting tutorial!
Last Modified: 27-Nov-2017
Great article. How would I modify this to restrict access to a page if user is not signed in?
ReplyDeleteIt is provided in the above code...
DeleteYou have to check if session is set or not,
if(isset($_SESSION['usr_id'])) {
//code goes here
} else {
//redirect to somewhere else
}
Where to put
Deleteif(isset($_SESSION['usr_id'])) {
//code goes here
} else {
//redirect to somewhere else
}
? and what code do u mena by codoe goes here?
Thank you so much for this wonderful tutorial:-)
ReplyDeleteGlad you liked it Michael! Cheers.
DeleteI have question sir, how can I add social login here? thanks..MORE POWER TO YOU.
DeleteHi! I have planned to write some detailed articles on social logins in the forthcoming days. Meantime you can try some good php libraries like HybridAuth. It makes social login process much easier.
DeleteThank you so much
ReplyDeleteidk why but for some reason i can't use the Login i get a dbconnect.php but i have check everything and it should work
ReplyDeleteHey nice job! Ty but would you create an additionally post with an editing-form-function for example: changeable username/email/password or delete-account func :)
ReplyDeleteIt's just amazing sir
ReplyDeleteI also tried so much to make a login and register system
http://17chapters.blogspot.in/2016/08/how-to-make-login-and-register-system.html
I hope you will like it sir please send me feedback and comment
Thank you so much and your one is just awesome
Any other post with session?
ReplyDeletehi sir, i am a computer science student , please help me my university project
ReplyDeletemy project name is complaint management system so that , if do you have any sample coding source code please send me my email address sivajasi2016@gmail.com
thank you...
ReplyDeleteHi
ReplyDeleteThis is a great guide. I am having trouble logging in once a user has registered, I get this error Warning: mysqli_fetch_array() expects parameter 1 to be mysqli_result, boolean given in on line 17. anyone else had this?
Thanks
Shane
I am getting this error login.php on line 17 Warning: mysqli_fetch_array() expects parameter 1 to be mysqli_result, boolean given in
ReplyDeleteAny ideas why?
Thanks
Shane
Hi! You get error because there's no registered user in the db. Try registering a user and then use those credentials to login.
DeleteI have complete filled each required fields without error but it says "Error in registering...Please try again later!" what is the problem? please help me :(
ReplyDeleteI have filled completely the register form without error on each fields but I always get this error "Error in registering...Please try again later!" what is the problem? .. I have checked my database .. but still it's not working , please help me :(
ReplyDeleteWhen I want to add username in fields I get this error "Error in registering...Please try again later!" :( how could I fix this?
ReplyDeletePerfecto
ReplyDeleteWant a material design login and register page, then check out here
ReplyDeletehttps://17chapters.blogspot.in/2016/08/how-to-make-login-and-register-system.html
It's just awesome
pls help me now i got this after i have finishing putting the script
ReplyDeleteParse error: syntax error, unexpected T_STRING in /home/a3496574/public_html/Open_An_Account.php on line 66
Why does it display ERROR on my browser when I read these files into my MAMP htdoc folder
ReplyDeleteHi, I just download the file and I put them into my MAMP htdocs folder, but when I load any of those files, the browser said "error", can you help me ?
ReplyDeleteI have some other .php pages but it works well
how from sign in to another page
ReplyDeleteHi,i am having problems with the login script. It gives the following error messages.
ReplyDeleteWarning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in /home/a1673109/public_html/wp-content/themes/twentyfourteen/login.php on line 13
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in /home/a1673109/public_html/wp-content/themes/twentyfourteen/login.php on line 14
Warning: mysqli_query() expects parameter 1 to be mysqli, null given in /home/a1673109/public_html/wp-content/themes/twentyfourteen/login.php on line 15
Warning: mysqli_fetch_array() expects parameter 1 to be mysqli_result, null given in /home/a1673109/public_html/wp-content/themes/twentyfourteen/login.php on line 17
Hello, anyway you can guide me through the process to convert this to PDO instead?
ReplyDeletegetting this error
ReplyDeleteWarning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\wamp\www\register.php on line 15
Thank yo very much. how i can create a private page for the user? like facebook wall ? where the user can put status or upload a picture ? thank you
ReplyDeleteyour explanation is so clear
ReplyDeletecan you help me please, im a student in web design and i need to do a project for my diploma.. its a donation website
....
ReplyDeleteThanks for that, took me a while to adapt it to my existing users database and to figure out how to incorporate the bootstrap element. It now looks like a professional base for my site on both tablet and pc, but on my phone it is unusable. The login and register buttons on the menu are not there and are replaced by a single button with 3 lines, I do not recognize this and it doesn't seem to correspond to the code.
ReplyDeletethere is no validation against identical input from user... otherwise a good tutorial
ReplyDeleteexactly.maybe you have idea how to maximaly simple solve such problem?
DeleteProbably by comparing name with all names in table and same with email...
Password can stay same ...;) or if not please correct me
First thanks for the great tutorial. I've been trying to figure this out for days!! and you are the first resource that I've had success with. Registration is working for me but my login has a bug in it. The system logs me in when I enter a valid user email address and password but the redirect doe not get me to the index.php page, which is at /testdb/index.php/, and I can access it via the browser.
ReplyDeleteWhen I login as a valid registered user the code is inserting "login.php" in the path statement and the browser takes me to /testdb/login.php/index.php, which generates the following error in the browser "ERR_TOO_MANY_REDIRECTS"
After logging in if I access the index page directly, the page shows the user is signed in.
Any idea what may be wrong with my code?
Thanks in advance for whatever assistance you may be able to provide.
Frank
First thanks for the great tutorial. I've been trying to figure this out for days!! and you are the first resource that I've had success with. Registration is working for me but my login has a bug in it. The system logs me in when I enter a valid user email address and password but the redirect doe not get me to the index.php page, which is at /testdb/index.php/, and I can access it via the browser.
ReplyDeleteWhen I login as a valid registered user the code is inserting "login.php" in the path statement and the browser takes me to /testdb/login.php/index.php, which generates the following error in the browser "ERR_TOO_MANY_REDIRECTS"
After logging in if I access the index page directly, the page shows the user is signed in.
Any idea what may be wrong with my code?
Thanks in advance for whatever assistance you may be able to provide.
Frank
Please how can this be implemented in production mode. That how do I connect the database to production mode, when the site goes live. Thanks
ReplyDeleteHi! You have to just change the connection details to that of the live server.
Deletemysqli_connect("localhost", "myusername", "mypassword", "testdb")
Like 'localhost' should be your live server name/ip and username and password also should be changed. Just get those details from your hosting service if you have trouble.
Hope this helps.
Cheers.
So that the login details of the users can be saved in production mode after building the authentication system
ReplyDeletehi, the script seems to work fine, but when I link from the index.php to my actual webpage with the
ReplyDeletein the beginning of the page it does not make any difference, I still can login to my webpage without longing in through the login page, what do I miss here?
still have not been able to prevent the link to my webpage even if not logged in! Please reply as soon as you can, thank you
ReplyDeleteHi! To provide access to only logged-in user, add the below code at the top of the page.
Deletesession_start();
if(isset($_SESSION['usr_id'])) {
header("Location: index.php");
}
Hei can you please add checking for existing username or existing email not to alow doubling the records in database for same user?
DeleteThanks!
Hi, I wonder if downloaded files bundle differs from files shown on this page?
ReplyDeleteI used files shown here on this page and it works differently... it doesnt show logged in user as on printscreens attached on this page? Thanks for your help!
How can I restrict signing in for users that are already in a database? Thanks!
ReplyDeleteNotice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 15
ReplyDeleteWarning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 15
Notice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 16
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 16
Notice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 17
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 17
Notice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 18
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 18
this is the promplem in register.php
Notice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 15
ReplyDeleteWarning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 15
Notice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 16
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 16
Notice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 17
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 17
Notice: Undefined variable: con in C:\xamp\htdocs\web\Register.php on line 18
Warning: mysqli_real_escape_string() expects parameter 1 to be mysqli, null given in C:\xamp\htdocs\web\Register.php on line 18
how can i fix this..need help
everything works great, thank you. is there an easy way to prevent the user from being registered until i approve there account? i suppose i would need to integrate a php mailer?
ReplyDeleteThe code works PERFECT with me. However, I need to know what I should change in the codes to do 2 things:
ReplyDelete1- Make the Password field requires a complex password with minimum 8 characters.
2- Make the Name field accepts other languages like Arabic.
Thanks for your help.
Thanks for these great tutorial, but assuming i want to add more field, like occupation, address e.t.c please how can i do that, example below
ReplyDelete//check if form is submitted
if (isset($_POST['signup'])) {
$name = mysqli_real_escape_string($con, $_POST['name']);
$email = mysqli_real_escape_string($con, $_POST['email']);
$password = mysqli_real_escape_string($con, $_POST['password']);
$cpassword = mysqli_real_escape_string($con, $_POST['cpassword']);
$job = mysqli_real_escape_string($con, $_POST['job']);
//name can contain only alpha characters and space
if (!preg_match("/^[a-zA-Z ]+$/",$name)) {
$error = true;
$name_error = "Name must contain only alphabets and space";
}
if(!filter_var($email,FILTER_VALIDATE_EMAIL)) {
$error = true;
$email_error = "Please Enter Valid Email ID";
}
if(strlen($password) < 6) {
$error = true;
$password_error = "Password must be minimum of 6 characters";
}
if($password != $cpassword) {
$error = true;
$cpassword_error = "Password and Confirm Password doesn't match";
}
if (!$error) {
if(mysqli_query($con, "INSERT INTO users(name,email,password,job) VALUES('" . $name . "', '" . $email . "', '" . md5($password) . "', '" . $job . "')")) {
$successmsg = "Successfully Registered! Click here to Login";
} else {
$errormsg = "Error in registering...Please try again later!";
}
}
}
?>
hi... sir very nice article..
ReplyDeleteplease provide css code of this index.php , register.php and login.php
on localhost css link does not work please provide full css code manually
my email is sameerahmadsaifi@gmail.com
Hey sorry for late reply. I haven't used custom css for any of my tutorials. Only Bootstrap css. Just download it from their official site and make use of it or try loading from cdn.
DeleteThis remains one of the best introductions to getting a user registration system online - FANTASTIC WORK! Can I ask, is there ANY chance you could also do a password reset for lost/forgotten passwords? I'm keen to stay within the great work you've done, but struggling a bit on how to do this. Please! :) THANK YOU for your great work.
ReplyDeleteHi thanks for your support! Yes I've planned to do it... perhaps in forthcoming tutorials. Stay tuned:)
DeleteDo you do customisations? I would like to add an email verification process when a new user registers, and the Password Reset feature in case a user forgot the password.
ReplyDeleteThis was really helpful tutorial. Thanks. :)
ReplyDeletethanks! saved me time and brain power, mucho gracias
ReplyDeleteWelcome :)
DeleteThis is how my login page comes out as
ReplyDeletehttps://gyazo.com/1fdd36874ec518bede063ee8fbaa7bac
I have done everything but my login page comes out like this
ReplyDeletehttps://gyazo.com/1fdd36874ec518bede063ee8fbaa7bac
It seems like you haven't included the bootstrap css file. Please download and include it. Mentioned about it in the article.
DeleteCheers.
Awsome! Thank you.
ReplyDelete