Hi! In today's post let's see how to upload and watermark image using php and bootstrap. Watermarking will protect your digital photos from unauthorized usage over internet. It's like embossing a small image or text (watermark) over other images to protect them from being copied. The process is done manually with any image editing tool like Photoshop. And the same can be done with PHP if you want to automate the process and do it in bulk. For this you need to provide a semi-transparent image to be used as watermark and it will be added to the original images programmatically.
Let's say you have a gallery page on a company website and often have to upload photos from various events to it. Wouldn't it be handy if all the images you upload are watermarked on fly and added to the gallery itself? Yep that's what we are going to do here. Upload image, watermark it with a stamp and display on the page.
I'm not going into details about creating image gallery. You can check it here. I'll keep this demo simple and mainly focused on watermarking the image.
Read:How to Upload Image and Watermark using PHP?
First make sure you have a transparent png image to use as watermark template. This can be anything like your business logo or text. And also create a folder 'uploads' in the application root to store all your watermarked images.
Then create index.php file and add a simple upload form to it.
<!DOCTYPE html> <html> <head> <title>Upload & Watermark Image with PHP</title> <meta name="viewport" content="width=device-width, initial-scale=1.0" > <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" type="text/css" rel="stylesheet" /> </head> <body> <br/> <div class="container"> <div class="row"> <div class="col-xs-8 col-xs-offset-2 well" style="background: none;"> <form action="index.php" method="post" enctype="multipart/form-data"> <legend>Select File to Upload:</legend> <div class="form-group"> <input type="file" name="file1" /> </div> <div class="form-group"> <input type="submit" name="submit" value="Upload" class="btn btn-danger"/> </div> </form> </div> </div> </div> </body> </html>
Create Image Source from Uploaded Photo:
Next receive the uploaded image, check for its file extension and create corresponding image source from the uploaded picture.
<?php if (isset($_POST['submit'])) { $img_name = $_FILES['file1']['name']; $img_source = $_FILES['file1']['tmp_name']; $img_type = strtolower($_FILES['file1']['type']); if($img_name!='') { // check for image type switch($img_type) { //Create new image from file case 'image/png': $image_src = imagecreatefrompng($img_source); break; case 'image/jpeg': $image_src = imagecreatefromjpeg($img_source); break; case 'image/gif': $image_src = imagecreatefromgif($img_source); break; default: $image_src = false; } } else { $msg = 'Please select an image for watermarking!'; } } ?>
Merge Watermark Image with Uploaded Image:
Then use the PHP imagecopy()
function to copy the watermark image to the original uploaded image and move it to the 'uploads' folder. Here you have to determine the position where the watermark should be placed. It can be right at the center or anywhere you want.
I want to play subtle here and positioned a small watermark at the bottom-right corner of the image. But you can go as bold as you want and add a bigger watermark and at the center. It's just up to you.
<?php $watermark_src = imagecreatefrompng($watermark_image); $destination_image = $folder_path . $img_name; // position watermark $right_margin = 20; $bottom_margin = 20; $watermark_width = imagesx($watermark_src); $watermark_height = imagesy($watermark_src); // merge watermark image with uploaded image imagecopy($image_src, $watermark_src, imagesx($image_src) - $watermark_width - $right_margin, imagesy($image_src) - $watermark_height - $bottom_margin, 0, 0, $watermark_width, $watermark_height); // save image to folder imagejpeg($image_src, $destination_image, 80); // release memory imagedestroy($image_src); imagedestroy($watermark_src); ?>
Display Watermarked Image on Page:
Now you have the watermarked image in the 'uploads' folder. Let's display it on the page.
<?php if(isset($destination_image)) { ?> <div class="col-xs-8 col-xs-offset-2 text-center well" style="background: none;"> <img src="<?php echo $destination_image; ?>" width=100% /> </div> <?php } ?>
Done! Here's the complete script for index.php demo we have created.
index.php
<?php // uploads folder $folder_path = 'uploads/'; $watermark_image = 'stamp.png'; if (isset($_POST['submit'])) { $img_name = $_FILES['file1']['name']; $img_source = $_FILES['file1']['tmp_name']; $img_type = strtolower($_FILES['file1']['type']); if($img_name!='') { // check for image type switch($img_type) { //Create new image from file case 'image/png': $image_src = imagecreatefrompng($img_source); break; case 'image/jpeg': $image_src = imagecreatefromjpeg($img_source); break; case 'image/gif': $image_src = imagecreatefromgif($img_source); break; default: $image_src = false; } if($image_src) { $watermark_src = imagecreatefrompng($watermark_image); $destination_image = $folder_path . $img_name; // position watermark $right_margin = 20; $bottom_margin = 20; $watermark_width = imagesx($watermark_src); $watermark_height = imagesy($watermark_src); // merge watermark image with uploaded image imagecopy($image_src, $watermark_src, imagesx($image_src) - $watermark_width - $right_margin, imagesy($image_src) - $watermark_height - $bottom_margin, 0, 0, $watermark_width, $watermark_height); // save image to folder imagejpeg($image_src, $destination_image, 80); // release memory imagedestroy($image_src); imagedestroy($watermark_src); } else { $msg = 'Invalid image type! Only png, jpg or gif allowed!!!'; } } else { $msg = 'Please select an image for watermarking!'; } } ?> <!DOCTYPE html> <html> <head> <title>Upload & Watermark Image with PHP</title> <meta name="viewport" content="width=device-width, initial-scale=1.0" > <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" type="text/css" rel="stylesheet" /> </head> <body> <br/> <div class="container"> <div class="row"> <div class="col-xs-8 col-xs-offset-2 well" style="background: none;"> <form action="index.php" method="post" enctype="multipart/form-data"> <legend>Select File to Upload:</legend> <div class="form-group"> <input type="file" name="file1" /> </div> <div class="form-group"> <input type="submit" name="submit" value="Upload" class="btn btn-danger"/> </div> <?php if(isset($msg)) { ?> <div class="alert alert-danger text-center"><?php echo $msg; ?></div> <?php } ?> </form> </div> <?php if(isset($destination_image)) { ?> <div class="col-xs-8 col-xs-offset-2 text-center well" style="background: none;"> <img src="<?php echo $destination_image; ?>" width=100% /> </div> <?php } ?> </div> </div> </body> </html>
Run the file and you'll see the upload page like this,
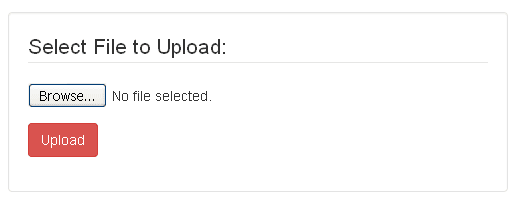
Select an image and click upload button. If everything goes right, the php script will upload, watermark and display the image right at the bottom of the upload form like this,

Also it does proper error handling. If the chosen image type is not a valid one, the watermarking script will show appropriate warning message.

That's it. You can use the same logic to watermark images in bulk. You have everything in place so tt wouldn't be hard to tweak the code to fit your logic.
Also Read:I hope after reading this, you got a good idea about watermarking images with php. If you think this tutorial helped you please share it in social media for others reference.
No comments:
Post a Comment