Hi, I have been writing codeigniter tutorials series for quite some time and already discussed about creating login form and contact form in codeigniter and bootstrap. One of the primitive features of any membership website is user registration system. Obviously the common trait of a signup form is to ask for too many details which will scare away users from your site. But keeping the form as minimal as possible will encourage them to sign up easily. Having said that today I’m going to show you, how to create a simple and elegant registration form in codeigniter along with email verification procedure.
Build Two-Step User Registration System with Email Verification
The codeigniter registration system we are going to build is a two step registration process namely 1. user registration and 2. user authentication process. It’s common now-a-days to send a confirmation link to user’s email-id during signup and activating the user’s account only when he/she confirms the email id they have provided at the time of registration. By this way we can keep away the spammers and attract only the serious users interested in the value provided by your site.
Must Read: Codeigniter Login, Logout & Sigup System using MySQL & Bootstrap
Steps for User Registration System
Here goes the flow for our user registration module.
- Ask user to fill in the basic details with email id in the registration form.
- Once validating the user submitted details, insert user data into database.
- Create a unique hash key and send a confirmation link to the user’s email id.
- User clicks on the confirmation link and return back to site.
- Verify the hash key within the link and activate the user’s account.
Creating Registration Form in CodeIgniter with Email Verification
Now we’ll move on to implement the above registration procedure in codeigniter. Beforehand, we’ll create mysql database required to store the user’s details.
Create MySQL Database
First create a database named ‘mysite’ and run the below sql command to create the ‘user’ table to save data.
SET SQL_MODE="NO_AUTO_VALUE_ON_ZERO"; CREATE TABLE IF NOT EXISTS `user` ( `id` int(12) NOT NULL AUTO_INCREMENT, `fname` varchar(30) NOT NULL, `lname` varchar(30) NOT NULL, `email` varchar(60) NOT NULL, `password` varchar(40) NOT NULL, `status` bit(1) NOT NULL DEFAULT b'0', PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
The status
field is set by default to ‘0’ indicating the deactivate state of the user, and it will be set to ‘1’ for activating the user account once we confirm the email id.
Next we’ll move on to creating required codeigniter files for this registration module.
The Model (‘user_model.php’)
Create a model file named ‘user_model.php’ inside ‘application/models’ folder. Then copy paste the below code to the file.
<?php class user_model extends CI_Model { function __construct() { // Call the Model constructor parent::__construct(); } //insert into user table function insertUser($data) { return $this->db->insert('user', $data); } //send verification email to user's email id function sendEmail($to_email) { $from_email = 'team@mydomain.com'; //change this to yours $subject = 'Verify Your Email Address'; $message = 'Dear User,<br /><br />Please click on the below activation link to verify your email address.<br /><br /> http://www.mydomain.com/user/verify/' . md5($to_email) . '<br /><br /><br />Thanks<br />Mydomain Team'; //configure email settings $config['protocol'] = 'smtp'; $config['smtp_host'] = 'ssl://smtp.mydomain.com'; //smtp host name $config['smtp_port'] = '465'; //smtp port number $config['smtp_user'] = $from_email; $config['smtp_pass'] = '********'; //$from_email password $config['mailtype'] = 'html'; $config['charset'] = 'iso-8859-1'; $config['wordwrap'] = TRUE; $config['newline'] = "\r\n"; //use double quotes $this->email->initialize($config); //send mail $this->email->from($from_email, 'Mydomain'); $this->email->to($to_email); $this->email->subject($subject); $this->email->message($message); return $this->email->send(); } //activate user account function verifyEmailID($key) { $data = array('status' => 1); $this->db->where('md5(email)', $key); return $this->db->update('user', $data); } } ?>
This model file takes care of all the background communication and we have created three functions,
1. insertUser()
to save the form data into database provided by user.
2. sendEmail()
to send verification email to the user’s email id provided at the time of registration. Here we generate a unique hash key with the user’s email-id and attach it with a link and mail it to the user. Read this tutorial on sending email in codeigniter using smtp to learn more about configuring email settings.
3. verifyEmailID()
to activate the user account after confirming the hash key from the earlier generated link. Here we update the status field from ‘0’ to ‘1’ representing the active state of the account.
Note: The above mentioned active state of the user will be used during user login process. Check this codeigniter login tutorial to see it in action.
The Controller (‘user.php’)
Next create a controller file named ‘user.php’ inside ‘application/controllers’ folder. And copy paste the below code to it.
<?php class user extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form','url')); $this->load->library(array('session', 'form_validation', 'email')); $this->load->database(); $this->load->model('user_model'); } function index() { $this->register(); } function register() { //set validation rules $this->form_validation->set_rules('fname', 'First Name', 'trim|required|alpha|min_length[3]|max_length[30]|xss_clean'); $this->form_validation->set_rules('lname', 'Last Name', 'trim|required|alpha|min_length[3]|max_length[30]|xss_clean'); $this->form_validation->set_rules('email', 'Email ID', 'trim|required|valid_email|is_unique[user.email]'); $this->form_validation->set_rules('password', 'Password', 'trim|required|matches[cpassword]|md5'); $this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required'); //validate form input if ($this->form_validation->run() == FALSE) { // fails $this->load->view('user_registration_view'); } else { //insert the user registration details into database $data = array( 'fname' => $this->input->post('fname'), 'lname' => $this->input->post('lname'), 'email' => $this->input->post('email'), 'password' => $this->input->post('password') ); // insert form data into database if ($this->user_model->insertUser($data)) { // send email if ($this->user_model->sendEmail($this->input->post('email'))) { // successfully sent mail $this->session->set_flashdata('msg','<div class="alert alert-success text-center">You are Successfully Registered! Please confirm the mail sent to your Email-ID!!!</div>'); redirect('user/register'); } else { // error $this->session->set_flashdata('msg','<div class="alert alert-danger text-center">Oops! Error. Please try again later!!!</div>'); redirect('user/register'); } } else { // error $this->session->set_flashdata('msg','<div class="alert alert-danger text-center">Oops! Error. Please try again later!!!</div>'); redirect('user/register'); } } } function verify($hash=NULL) { if ($this->user_model->verifyEmailID($hash)) { $this->session->set_flashdata('verify_msg','<div class="alert alert-success text-center">Your Email Address is successfully verified! Please login to access your account!</div>'); redirect('user/register'); } else { $this->session->set_flashdata('verify_msg','<div class="alert alert-danger text-center">Sorry! There is error verifying your Email Address!</div>'); redirect('user/register'); } } } ?>
In the controller file we have created two functions namely, register()
for the first step of the registration process and verify()
for authenticating the user.
Also Read: How to Submit Form using AJAX in CodeIgniter
CodeIgniter Registration Form Validation
In the register() method, we asks the user to fill in the details, submit it and validate the form data. As I have said earlier, we have a signup form with minimal fields for first name
, last name
, email-id
and password
. It’s a good practice to ask the user to re-enter the password once again and we confirm if both the passwords match or not. If there is any mismatch we notify the user with an error message like this.

We also confirm if the email-id provided by user is unique and not used already for another account. Codeigniter provides a simple way to confirm this with the built-in validation function is_unique[user.email]
where ‘user’ is the database table name and ‘email’ is the database column name to check out.
Once we validate the user details and confirm everything is ok, we insert the data into database with the line $this->user_model->insertUser($data)
. And send the mail to user’s email-id using $this->user_model->sendEmail($this->input->post('email'))
.
For generating the hash key, I have md5()
‘ed the user’s email-id and sent it with the confirmation link. By this way, we don’t have to store a separate hash key in the database for each user. However you can generate a random hash and store in the database for later verification. It’s up to you.
After successfully sending the email, we notify the user to confirm their email-id to complete the registration process like this.

Later when the user clicks on the email link, they will be taken back to the site and to the verify() function in the controller. The hash code will be tested against the email id stored in the database and that particular user account will be activated and will be promptly notified like this.

Related Read: Beginners Guide to Database CRUD using CodeIgniter and Bootstrap
The View (‘user_registration_view.php’)
We have created model and controller file for user registration. Now it’s time to build user interface i.e., registration form with all the required form fields. For that create a view file named ‘user_registration_view.php’ inside ‘application/views’ folder and copy paste the below code to it.
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>CodeIgniter User Registration Form Demo</title> <link href="<?php echo base_url("bootstrap/css/bootstrap.css"); ?>" rel="stylesheet" type="text/css" /> </head> <body> <div class="container"> <div class="row"> <div class="col-md-6 col-md-offset-3"> <?php echo $this->session->flashdata('verify_msg'); ?> </div> </div> <div class="row"> <div class="col-md-6 col-md-offset-3"> <div class="panel panel-default"> <div class="panel-heading"> <h4>User Registration Form</h4> </div> <div class="panel-body"> <?php $attributes = array("name" => "registrationform"); echo form_open("user/register", $attributes);?> <div class="form-group"> <label for="name">First Name</label> <input class="form-control" name="fname" placeholder="Your First Name" type="text" value="<?php echo set_value('fname'); ?>" /> <span class="text-danger"><?php echo form_error('fname'); ?></span> </div> <div class="form-group"> <label for="name">Last Name</label> <input class="form-control" name="lname" placeholder="Last Name" type="text" value="<?php echo set_value('lname'); ?>" /> <span class="text-danger"><?php echo form_error('lname'); ?></span> </div> <div class="form-group"> <label for="email">Email ID</label> <input class="form-control" name="email" placeholder="Email-ID" type="text" value="<?php echo set_value('email'); ?>" /> <span class="text-danger"><?php echo form_error('email'); ?></span> </div> <div class="form-group"> <label for="subject">Password</label> <input class="form-control" name="password" placeholder="Password" type="password" /> <span class="text-danger"><?php echo form_error('password'); ?></span> </div> <div class="form-group"> <label for="subject">Confirm Password</label> <input class="form-control" name="cpassword" placeholder="Confirm Password" type="password" /> <span class="text-danger"><?php echo form_error('cpassword'); ?></span> </div> <div class="form-group"> <button name="submit" type="submit" class="btn btn-default">Signup</button> <button name="cancel" type="reset" class="btn btn-default">Cancel</button> </div> <?php echo form_close(); ?> <?php echo $this->session->flashdata('msg'); ?> </div> </div> </div> </div> </div> </body> </html>
Note: As for the style sheet I used twitter bootstrap css, but feel free to use your own if you prefer.
Recommended Read: Quick Guide to Integrate Twitter Bootstrap CSS with CodeIgniter
The above view file produces a minimal and elegant registration form like this.
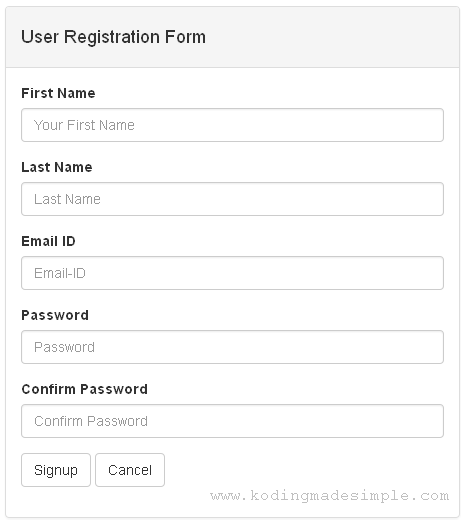
As you can see in the view file, I have used several css styles (‘class’) to quickly design this form and those are Bootstrap’s build-in form classes. To display the form validation errors, I have used codeigniter’s form_error()
function below the corresponding form input fields.
Also the line $this->session->flashdata();
is used to notify success/failure message after registration process. Unlike simple echoed messages, the flashdata message will be displayed only once after the redirection takes place.
And that was all about creating user registration form in codeigniter with email confirmation. I hope this two step registration system will be helpful to you. If you have any trouble implementing it, please voice it through your comments.
Recommended Read:- How to Create Login Form in CodeIgniter and MySQL Database
- Create Contact Form in CodeIgniter with Database Storage Option
P.S.: For those who faced password matching issues, I have tweaked the validation rules a little to fix it. You can check it now.
Last Modified: 14-Nov-2017
why am i getting database error? it says no database selected after i clicked sign up
ReplyDeleteHi, you have to select the database first. For that config the database settings in 'application/config/database.php' file like this,
Delete$db['default']['hostname'] = 'localhost';
$db['default']['username'] = 'mysql_username';
$db['default']['password'] = 'mysql_password';
$db['default']['database'] = 'mysite';
$db['default']['dbdriver'] = 'mysql';
Then it will work.
Cheers.
Verification code not updating data..
ReplyDeleteI am getting "The Confirm Password field does not match the Password field." error even if typing same passwords!
ReplyDeletedo i need to do anything on user_model.php file???
user_model.php is here
Deleteplease try this code
Delete$this->form_validation->set_rules('password', 'password', 'trim|required|md5');
$this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required|md5|matches[password]');
It works. Thanks.
DeleteI am getting The Confirm Password field does not match the Password field. error
ReplyDeleteHi! It works properly here. Please check if you have any typos with the password field name.
DeleteCheers.
hi, same prople with The Confirm Password field does not match the Password field.
ReplyDelete$this->form_validation->set_rules('password', 'Password', 'trim|required|matches[cpassword]|md5');
Delete$this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required|md5');
Just change your code like above. matches[] need to be put in first element of password, not in last one.
I am sorry for asking this nonsense question but I really dont know how to open the page once I set up all above files.
DeleteIf you have the demo application say 'cidemo' in the root folder (htdocs in xampp, www in wamp), then access the page like this,
Deletelocalhost/cidemo/index.php/user
Cheers.
it displays error
Deletepage not found
404 Page Not Found
The page you requested was not found.
Delete404 Page Not Found
The page you requested was not found.
Hi! I got the problem when I click Sign Up. It says "Oops! Error. Please try again later!!!"
ReplyDeleteCan you help me please?
Hi! That error pops up if mail is not sent. Please verify if you have proper smtp settings. Check this below link for right smtp settings.
Deletehttp://www.kodingmadesimple.com/2015/03/how-to-send-email-in-codeigniter-smtp.html
Cheers.
Hi! You need to turn on your gmail setting
Deletehttps://www.google.com/settings/security/lesssecureapps
Hi! You need to turn on your gmail setting first.
Deletehttps://www.google.com/settings/security/lesssecureapps
Thank you all guys. It works now. :D
DeleteStill not working... what seems to be the problem?
Deletei get erroe in sql statement
ReplyDeleteWhat error you get?
Deletei cant demo this simple project, i dont understand, can you give me a tutorial?
Deletei cant demo this simple code, can you give me some tutorial?
Deletepassword and cpassword doesnot match.
ReplyDeleteHi, when I clicked on Sign Up, nothing happened. Can you help me please?
ReplyDeleteMySQL ha dicho: Documentación
ReplyDelete#1064 - You have an error in your SQL syntax; check the manual that corresponds to your MariaDB server version for the right syntax to use near 'b’0’,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_IN' at line 7
Hi! There's some quotation error with the given sql statement. I got it fixed. Please recheck the post now.
DeleteCheers.
Getting this error when I am going to create the table -
ReplyDelete#1064 - You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'b’0’,
PRIMARY KEY (`id`)' at line 7
#1064 - You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'b’0’,
ReplyDeletePRIMARY KEY (`id`)' at line 7
#1064 - You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'b’0’,
ReplyDeletePRIMARY KEY (`id`)' at line 7
Hey! There seems to be some error with quotation marks in the provided sql. I got it fixed. Please recheck the post.
DeleteCheers.
Wow this worked perfectly
ReplyDeletebut im having an issue like the email sent from my site goes into the spam folder in receiver mail.
Any workaround?
Hi! There is no specific workaround to this. Every email provider have their own spam filtering rules.
DeleteYou can check this thread to know more in detail,
http://stackoverflow.com/questions/29697841/phpmailer-sends-email-to-junk-or-spam-folder-using-gmail-smtp
Cheers.
i got stuck
DeleteUnable to access an error message corresponding to your field name First Name.(xss_clean)
$this->load->helper(array('form','url','security'));
Deleteadd 'security' in user.php file
and to everyone having issue with the password and confirm password error message that always said both field doesn't match, try changing this line from user.php (controller)
ReplyDeletefrom : $this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required|matches[password]md5');
to $this->form_validation->set_rules('cpassword', 'Confirm Password', 'trim|required|md5|matches[password]');
move the "md5" before matches, as it will convert to md5 first then compare to the password field
ok, this is so strange
ReplyDeletei succesfully sent a mail with verified link from localhost, but failed when tried it from online hosting
the data went to database, but the email not being send
Make sure the mail sending option is enabled else contact your service provider.
DeleteCheers.
Muh. Adi Fahmi, how can i send mails from localhost?
DeleteVery good and simple tutorial but people are making jokes
ReplyDeleteThanks for your support :)
DeleteVery nice article.if the article explain for lot of new information.
ReplyDeletehadoop training in chennai | informatica training in chennai
Welcome Vignesh! Thanks for your support.
Deletenothing happens when i click sign up
ReplyDeletePlease check this article. This will show you how to configure and run CI files.
Deletehttp://www.kodingmadesimple.com/2015/05/install-setup-php-codeigniter-framework-xampp-localhost.html
Cheers.
hi when i am verify the email set flashdata not working
ReplyDeletewhen i have verify the emial set flashdta not working
ReplyDeleteUnable to access an error message corresponding to your field name First Name.(xss_clean)
ReplyDeleteUnable to access an error message corresponding to your field name Last Name.(xss_clean)
ReplyDeletedelete xss_clean :)
Delete$this->form_validation->set_rules('lname', 'Last Name', 'trim|required|alpha|min_length[3]|max_length[30]');
Delete "xss_clean"
DeleteNice post..keep sharing your information regularly for my future reference. QTP Training in Chennai | Software Testing Training in Chennai
ReplyDeleteFatal error: Call to undefined function form_open() in C:\wamp64\www\CodeIgniter-3.0.6\application\views\user_registration_view.php on line 24
ReplyDeletewhile run the file it will sow an error like this
ReplyDelete$db['test'] = array( 'dsn' => '', 'hostname' => 'localhost', 'username' => 'root', 'password' => '', 'database' => 'mysite', 'dbdriver' => 'mysql', 'dbprefix' => '', 'pconnect' => TRUE, 'db_debug' => TRUE, 'cache_on' => FALSE, 'cachedir' => '', 'char_set' => 'utf8', 'dbcollat' => 'utf8_general_ci', 'swap_pre' => '', 'encrypt' => FALSE, 'compress' => FALSE, 'stricton' => FALSE, 'failover' => array() );
An Error Was Encountered
No database connection settings were found in the database config file.
i got an error!!
ReplyDelete$db['test'] = array( 'dsn' => '', 'hostname' => 'localhost', 'username' => 'root', 'password' => '', 'database' => 'mysite', 'dbdriver' => 'mysql', 'dbprefix' => '', 'pconnect' => TRUE, 'db_debug' => TRUE, 'cache_on' => FALSE, 'cachedir' => '', 'char_set' => 'utf8', 'dbcollat' => 'utf8_general_ci', 'swap_pre' => '', 'encrypt' => FALSE, 'compress' => FALSE, 'stricton' => FALSE, 'failover' => array() );
An Error Was Encountered
No database connection settings were found in the database config file.
got an error
ReplyDelete$db['test'] = array( 'dsn' => '', 'hostname' => 'localhost', 'username' => 'root', 'password' => '', 'database' => 'mysite', 'dbdriver' => 'mysql', 'dbprefix' => '', 'pconnect' => TRUE, 'db_debug' => TRUE, 'cache_on' => FALSE, 'cachedir' => '', 'char_set' => 'utf8', 'dbcollat' => 'utf8_general_ci', 'swap_pre' => '', 'encrypt' => FALSE, 'compress' => FALSE, 'stricton' => FALSE, 'failover' => array() );
An Error Was Encountered
No database connection settings were found in the database config file.
got error!
ReplyDeleteYou have not specified a database connection group via $active_group in your config/database.php file.
Hi! Refer this link for proper database configuration in CI.
Deletehttp://www.kodingmadesimple.com/2015/05/install-setup-php-codeigniter-framework-xampp-localhost.html
Hello,
ReplyDeletei get this error when I click register. Bu tth data is in my db table.
A PHP Error was encountered
Severity: Notice
Message: Undefined property: Register::$email
Filename: core/Model.php
Line Number: 77
Backtrace:
File: /var/www/clients/client0/web3/web/application/models/Register_model.php
Line: 49
Function: __get
File: /var/www/clients/client0/web3/web/application/controllers/Register.php
Line: 78
Function: sendEmail
File: /var/www/clients/client0/web3/web/application/controllers/Register.php
Line: 21
Function: register
File: /var/www/clients/client0/web3/web/index.php
Line: 315
Function: require_once
Fatal error: Call to a member function initialize() on null in /var/www/clients/client0/web3/web/application/models/Register_model.php on line 49
A PHP Error was encountered
Severity: Error
Message: Call to a member function initialize() on null
Filename: models/Register_model.php
Line Number: 49
Backtrace:
ReplyDeleteHello, I get the following error but when I check my data base the data is in there. No email sent also.
A PHP Error was encountered
Severity: Notice
Message: Undefined property: Register::$email
Filename: core/Model.php
Line Number: 77
Backtrace:
File: /var/www/clients/client0/web3/web/application/models/Register_model.php
Line: 49
Function: __get
File: /var/www/clients/client0/web3/web/application/controllers/Register.php
Line: 78
Function: sendEmail
File: /var/www/clients/client0/web3/web/application/controllers/Register.php
Line: 21
Function: register
File: /var/www/clients/client0/web3/web/index.php
Line: 315
Function: require_once
Fatal error: Call to a member function initialize() on null in /var/www/clients/client0/web3/web/application/models/Register_model.php on line 49
A PHP Error was encountered
Severity: Error
Message: Call to a member function initialize() on null
Filename: models/Register_model.php
Line Number: 49
Backtrace:
change this code:
Deleteif ($this->user_model->insertUser($data)) {
$to_email= $this->input->post('email', TRUE);
if ($this->user_model->sendEmail($to_email)) {
when i click on sogn up i got this error
ReplyDeleteUnable to access an error message corresponding to your field name First Name.(xss_clean)
The Password field does not match the Confirm Password field.
when i clcik on signup i got this error
ReplyDeleteUnable to access an error message corresponding to your field name First Name.(xss_clean)
Hi, i have created and uploaded this files to an external hosting. The first page is working fine, When I click on sign up, the page is going to http://127.0.0.6/index.php/user/register intead of my hosting domain address! how could I fix this issue
ReplyDeleteYou have done really great job. Thanks for sharing the informative blog. Its really useful for us. Core Java Training | Mainframe Training | J2EE Training | SQL Server DBA Training
ReplyDeleteHi, I have read your blog. Really very informative and useful for us. Also your blog content is very unique. Keep posting. Dot Net Training | Oracle DBA Training | Core Java Training
ReplyDeleteMessage: Undefined property: CI_Loader::$session
ReplyDeleteFilename: views/user_registration_view.php
That's the error i'm getting
When i click on signup, it shows Error 404 on this address
ReplyDeletehttp://127.0.0.1/CI/index.php/user/register
What is wrong?
Thank you for this code. I am completely new to php. I am trying to this code for my site. I have only changed the database related information all rest is kept as it is. The user registration data is correctly saved in the database and all the other functions are working perfectly however the login is not working ... after registering when I try to login it stays on the login screen does not go to the home page. Please help!! Thanks in advance. R Programming Training | DataStage Training | SQL Training | SAS Training | Android Training| SharePoint Training
ReplyDeleteThis is dency from chennai. I have read your blog. Its very useful for me and gathered some interesting informations. I have applied to this. Keep going on. Thank for a good post.
ReplyDelete[url="http://www.traininginsholinganallur.in/web-designing-training-in-chennai.html"]Web design Training in chennai [/url]
404 Page not found.
ReplyDeleteGet this error, how to fix it ?
Please help me.
Hi, This is really nice blog. You have done really great job. Keep posting. Big Data Training | Hadoop Admin Training | Devops Training | Oracle DBA Online Training
ReplyDeletethe best cognos training in chennai.the best cognos training in chennai»the best cognos training in chennai.
ReplyDeleteAfter i click signup it says Object not found!Error 404
ReplyDeleteAfter i click signup it says Object not found!Error 404
ReplyDelete100% Job Oriented R Programming Training In Chennai for more Information click to
ReplyDeletethe best data -warehousing training in chennai
Local form that I send email from my server not
ReplyDeleteLocal form that I send email from my server not
ReplyDeletefunction sendEmail($to_email)
ReplyDelete{
$from_email = 'xxxxxx@gmail.com'; //Cambiar esto a la suya
$subject = 'Verifique su dirección de correo electrónico';
$message = 'Querido usuario,
Por favor, haga clic en el enlace de activación a continuación para verificar su dirección de correo electrónico.
http://xxxxxx.co/xxxxx/xxxxxx/verify/' . md5($to_email) . '
Gracias
';
// configurar los ajustes de correo electrónico
$config['useragent'] = 'CodeIgniter';
$config['protocol'] = 'smtp';
$config['smtp_crypto'] = 'ssl';
$config['smtp_host'] = 'smtp.gmail.com'; //smtp host name
$config['smtp_port'] = '465'; // número de puerto SMTP
$config['smtp_user'] = $from_email;
$config['smtp_pass'] = 'xxxxxx'; // $ FROM_EMAIL contraseña
$config['mailtype'] = 'html';
$config['charset'] = 'utf-8';
$config['wordwrap'] = TRUE;
$config['newline'] = "\r\n"; // utilizar comillas dobles
$this->email->initialize($config);
// enviar correo
$this->email->from($from_email, 'xxxxxxx');
$this->email->to($to_email);
$this->email->subject($subject);
$this->email->message($message);
return $this->email->send();
}
send email locally but not in my hosting
its workinf very fine in my system but, when i will to confirm by link in the email its show error system not found. how will i solve this error? thanks in advance
ReplyDeleteAfter copying all this code i enter mywebsitename/index.php/user and it shows me 404 page not found.What should I have to do?
ReplyDeleteThanks for sharing this wonderful post.
ReplyDeleteBest Informatica Training in Chennai
Thanks for sharing this useful post.
ReplyDeleteSelenium Training in Chennai
Hii,
ReplyDeletefound very informative articles to learn, added few more information.
Thanks for sharing this wonderful articles.
keep updating more :)
Amazon Web Services Training in Chennai
Thanks for sharing the valuable information.
ReplyDeletedot net training in chennai
Thanks for sharing this helping post. keep updating more :)
ReplyDeleteOracle Forms & Reports Training in chennai
A PHP Error was encountered
ReplyDeleteSeverity: Warning
Message: mysqli::real_connect(): (HY000/1044): Access denied for user ''@'localhost' to database 'mysite'
Filename: mysqli/mysqli_driver.php
Line Number: 202
Backtrace:
File: D:\xampp\htdocs\CI\form\application\controllers\User.php
Line: 7
Function: database
File: D:\xampp\htdocs\CI\form\index.php
Line: 315
Function: require_once
A Database Error Occurred
Unable to connect to your database server using the provided settings.
Filename: D:/xampp/htdocs/CI/form/system/database/DB_driver.php
Line Number: 436
I found this error please give the solution.in my mail twinkalkushwaha@gmail.com
Hi, thanks for the tutorial. Question: in the code that you provided there is md5 hashing, is it possible a stronger encryption like bcrypt?
ReplyDeleteThanks in advance
after completing this how call it into localhost?
ReplyDeleteafter completing how i used it into localhost?
ReplyDeleteHi I am getting below error message . I have check all file but not able to understand exact issue
ReplyDelete404 Page Not Found
The page you requested was not found.
Hi i am getting below error message i check all file but not get exact issue
ReplyDelete404 Page Not Found
The page you requested was not found.
Thanks a lot very sharing this idea install information is really good.I'll be very like this blog web page.
ReplyDeleteSelenium Online Training
why i got this "The Email ID field must contain a unique value."
ReplyDeletethis source doesn't work. Why?
ReplyDeletemail functionality is not working
ReplyDeletemail functionality is not working
ReplyDeleteUnable to access an error message corresponding to your field name First Name.(xss_clean) this error occure in refistraion form
ReplyDeleteUnable to access an error message corresponding to your field name First Name.(xss_clean) this error occure in regestration form
ReplyDeleteWe have to develop blog information for our creativity mind.This blog is very helping for us. Thanks a lot. It's very interesting and useful blog.
ReplyDeleteSEO Training in Chennai
SEO Training Course in Chennai
Hi.
ReplyDeletei have Got This error
" Oops! Error. Please try again later!!! "
Even though i have already save a File "email.php" in config Folder and also trun on "Allow less secure apps: ON" by going on that Link
https://myaccount.google.com/lesssecureapps?pli=1
please help.!!!
same problem in my code
Deletestatus not changed when i click on verification link
ReplyDeletenothing happened when i click on verification link
ReplyDeleteWhy i get this error?
ReplyDeleteA Database Error Occurred
Error Number: 1146
Table 'edu-center.user' doesn't exist
SELECT * FROM `user` WHERE `email` = 'adil@sdasd.ru' LIMIT 1
Filename: C:/xampp/htdocs/edu-center/system/database/DB_driver.php
Line Number: 691
got an error message..! what is this? Unable to access an error message corresponding to your field name First Name.(xss_clean)
ReplyDeleteA PHP Error was encountered
ReplyDeleteSeverity: Notice
Message: Undefined property: Kontributor::$email
Filename: core/Model.php
Line Number: 77
Backtrace:
File: C:\xampp\htdocs\TOHO_CI\application\models\Kontributor_m.php
Line: 29
Function: __get
File: C:\xampp\htdocs\TOHO_CI\application\controllers\kontributor.php
Line: 73
Function: sendEmail
File: C:\xampp\htdocs\TOHO_CI\index.php
Line: 315
Function: require_once
Fatal error: Call to a member function initialize() on null in C:\xampp\htdocs\TOHO_CI\application\models\Kontributor_m.php on line 29
A PHP Error was encountered
Severity: Error
Message: Call to a member function initialize() on null
Filename: models/Kontributor_m.php
Line Number: 29
Backtrace:
error when send email :(
I get below error when i run
ReplyDeleteAn uncaught Exception was encountered
Type: Error
Message: Call to a member function num_rows() on boolean
Filename: C:\Apache24\htdocs\mossis\system\libraries\Form_validation.php
Line Number: 1122
Backtrace:
File: C:\Apache24\htdocs\mossis\application\controllers\User.php
Line: 28
Function: run
File: C:\Apache24\htdocs\mossis\index.php
Line: 315
Function: require_once
Why is the sendEmail($to_email) function inside the model and not the controller?
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteIs there an online demo?
ReplyDelete