Codeigniter provides a wide number of helper class and libraries for rapid application development. Upload library is one among them that makes file uploading process in Codeigniter a breeze. We'll see how to do file and image upload in codeigniter in this tutorial. File and Image uploading are similar since images are themselves files. But there are options for you to restrict the uploading process specific to images files alone and we'll see it below.
File Upload in CodeIgniter
First of all, we need a destination folder to store all the uploaded files. So create a folder named 'uploads' in the root folder. Make sure it is writable for the uploading process to work.
Read Also:- How to Create Login Page in CodeIgniter & MySQL
- How to Send Email in CodeIgniter using Gmail
- CodeIgniter Pagination System using Bootstrap
Create Controller
Create a controller file 'uploadfile.php' inside 'application\controllers' folder in codeigniter.
<?php class uploadfile extends CI_Controller { function __construct() { parent::__construct(); $this->load->helper(array('form', 'url')); } //index function function index() { //load file upload form $this->load->view('upload_file_view'); } //file upload function function upload() { //set preferences $config['upload_path'] = './uploads/'; $config['allowed_types'] = 'txt|pdf'; $config['max_size'] = '100'; //load upload class library $this->load->library('upload', $config); if (!$this->upload->do_upload('filename')) { // case - failure $upload_error = array('error' => $this->upload->display_errors()); $this->load->view('upload_file_view', $upload_error); } else { // case - success $upload_data = $this->upload->data(); $data['success_msg'] = '<div class="alert alert-success text-center">Your file <strong>' . $upload_data['file_name'] . '</strong> was successfully uploaded!</div>'; $this->load->view('upload_file_view', $data); } } } ?>
The upload()
method in the codeigniter controller is where the actual file upload process takes place. Here we load the 'upload' library with the statement $this->load->library('upload', $config);
. The $config is the array where we set preferences to control the uploading process. This is where we define the file uploading path, allowed file extensions, maximum file size etc.
This controller upload() method will be invoked on form submission, and the file will be validated and shows the validation error in case it fails. Else it will upload the file to the destination folder.
We have used some of the important methods of the 'upload' class library in our controller.
- The callback,
$this->upload->do_upload()
will upload the file selected in the given field name to the destination folder. Note that the parameter to this method is optional and if it is not mentioned, then it expects the file from the input field with name 'userfile'. So if you set the file input with name other than 'userfile' make sure you pass the field name as parameter to thedo_upload()
function. - The callback
$this->upload->display_errors()
returns the error message if the do_upload() method returns false. - And the callback
$this->upload->data()
returns an array of data related to the uploaded file like the file name, path, size etc.
Create View
Create the view file 'upload_file_view.php' inside 'application\views' folder. This view file contains a simple upload form with file input and a submit button.
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>CodeIgniter File Upload Form</title> <!-- load bootstrap css file --> <link href="<?php echo base_url("assets/bootstrap/css/bootstrap.css"); ?>" rel="stylesheet" type="text/css" /> </head> <body> <div class="container"> <div class="row"> <div class="col-md-6 col-md-offset-3 well"> <legend>CodeIgniter File Upload Demo</legend> <?php echo form_open_multipart('uploadfile/upload');?> <fieldset> <div class="form-group"> <div class="row"> <div class="col-md-12"> <label for="filename" class="control-label">Select File to Upload</label> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-12"> <input type="file" name="filename" size="20" /> <span class="text-danger"><?php if (isset($error)) { echo $error; } ?></span> </div> </div> </div> <div class="form-group"> <div class="row"> <div class="col-md-12"> <input type="submit" value="Upload File" class="btn btn-primary"/> </div> </div> </div> </fieldset> <?php echo form_close(); ?> <?php if (isset($success_msg)) { echo $success_msg; } ?> </div> </div> </div> </body> </html>
The above view will produce an upload form like this.
![]() |
CodeIgniter File Upload Demo Form |
Generally I use bootstrap css framework to design the html forms for all my tutorials. This tutorial is no exception and I have used bootstrap components to give a nice look and feel to our upload form. Also I have set the form type to 'multipart' for the file uploading to work. (If you are a newbie to bootstrap then take a look at this tutorial on using bootstrap with codeigniter php framework to learn more).
When the user submits the form, it will be validated against the preferences set by the $config[] array. If the file is not selected or any other form validation error occurs, it is displayed below the file input like this.
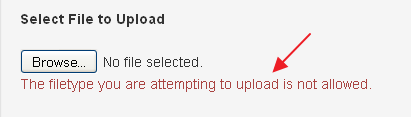
If the validation succeeds and the file is uploaded successfully to the destination folder, a success message will be displayed at the bottom of the form like this.
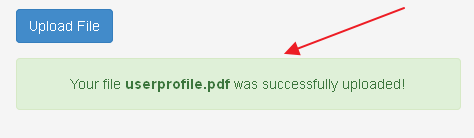
Uploading Images in CodeIgniter
If you want to restrict the user to upload only image files in codeigniter, then you can set the preferences to something like this in the controller file,
<?php $config['upload_path'] = './uploads/'; $config['allowed_types'] = 'png|jpg|gif'; $config['max_size'] = '150'; $config['max_width'] = '1024'; /* max width of the image file */ $config['max_height'] = '768'; /* max height of the image file */ ?>
Hence in this way you can restrict the users to upload only images. Moreover all those image files should fall under the maximum file size, width and height set by you :).
Read Also:- How to Resize Uploaded Image and Create Thumbnail in CodeIgniter
- How to Upload Multiple Files & Images in CodeIgniter (At Once)
- Fetch Data From Database using AJAX & CodeIgniter
And that explains the file and image uploading process in codeigniter. I hope you like this turorial. Meet you in another interesting post. Please don't forget to share this on your social circle. Good Day!!!
I have some doubt?? above code works perfect for me but what to do when i have form with some fields like name email etc and image upload form with it.Do i have different controller for image and form or can be done in same controller.
ReplyDeleteHi, you can use the same controller function to upload and insert form fields. First validate the post data, upload image and insert the data into DB.
DeleteUse this post for reference,
www.kodingmadesimple.com/2015/01/codeigniter-bootstrap-insert-form-data-into-database.html
Hope that helps.
Cheers.
nice thread gaann..
ReplyDeleteGlad you liked it...Cheers.
DeleteHow if we have 2 images or 2 files to upload? How to modify that code?
ReplyDeleteHi, you have to create file input field as an array (filename[]) and should loop through the filename[] array to upload images/files one by one.
DeleteThis stackoverflow thread explains the concept better.
http://stackoverflow.com/questions/20113832/multiple-files-upload-in-codeigniter
Cheers.
Hai Valli Pandy, what is the different between public function and function only in controller and model?
ReplyDeleteBoth ways it refers as public visibility. The functions can be declared as public, private or protected but if they are not defined then it is considered as public. Though it's a good practice to use explicit visibility keywords.
DeleteHope that explains.
Cheers.
CI set_value not working with jquery datepicker .
ReplyDelete$data = array(
'type' => 'text',
'name' => 'c_dob',
'id' => 'c_dob',
'value' =>set_value('c_dob'),
'class' => 'form-control',
'placeholder'=>'DATE OF BIRTH'
);
echo form_input($data);
any help ???
Please refer this below CI tutorial to know how to set_value for datepicker control!
Deletehttp://www.kodingmadesimple.com/2015/01/codeigniter-bootstrap-insert-form-data-into-database.html
Cheers.
can you more explain about the 'upload_path' ?
ReplyDeletei not understand how to make upload path where i want to save the upload file.
Thanks