Hi! In this tutorial we'll see how to populate dropdown from database in codeigniter and validate the said drop down list with custom form validation. In codeigniter, you have to use the form helper's function form_dropdown() to add dropdown list (combo box) to html forms. The function takes up associative array as argument which will then be added as field options.
To populate the drop down from database in a form, you have to fetch the data from DB, convert it into an array of (key, value) pairs and pass it as an argument to the function. Let's see in detail how to do this in codeigniter.
Populate Dropdown from Database in CodeIgniter:
Consider this MySQL database with tables 'Category' & 'Books'.

From the above db diagram it's evident that the table 'Books' contains a foreign key CategoryID
which is dependent on the parent table 'Category'. So in any case if we create a form to insert book details to database, the 'Category' field should be kept as a dropdown list in the form which in turn should be populated from the parent table.
Hence we have to fetch all category id & names from tbl 'Category' and add it to the dropdown field making the user to choose any of the category name from the available list. Category id won't be visible to the user, but during db insertion the id will be inserted into database instead of name.
In codeigniter, to populate drop down list from database first create a function getCategory()
in model file to fetch category id & name from the database and returns them as an associative array.
Model:
<?php class demo_model extends CI_Model { function __construct() { parent::__construct(); } function getCategory() { $query = $this->db->get('category'); $result = $query->result(); $cat_id = array('-CHOOSE-'); $cat_name = array('-CHOOSE-'); for ($i = 0; $i < count($result); $i++) { array_push($cat_id, $result[$i]->CategoryID); array_push($cat_name, $result[$i]->CategoryName); } return array_combine($cat_id, $cat_name); } } ?>
Next in a controller file make a call back to the model function created and pass category details as an array to the view file. This array will then be used in the view to populate the dropdown 'category'.
Plus if you want to validate the drop down input, then you must also need to add a custom validation function to the controller which ensures if user selects valid category name or not.
Controller:
<?php class demo extends CI_Controller { function __construct() { parent::__construct(); $this->load->helper(array('form', 'url')); $this->load->library('form_validation'); $this->load->database(); $this->load->model('demo_model'); } function index() { $data['category'] = $this->demo_model->getCategory(); $this->form_validation->set_rules('bookname', 'Book Name', 'trim|required'); $this->form_validation->set_rules('category', 'Category', 'callback_validate_dropdown'); $this->form_validation->set_rules('author', 'Author', 'trim|required'); $this->form_validation->set_rules('isbn', 'ISBN', 'trim|required'); if ($this->form_validation->run() == FALSE) { // failed validation $this->load->view('demo_view', $data); } else { // here goes your code to insert into db echo "Hooray! Now write down your code to insert book details into database..."; } } function validate_dropdown($str) { if ($str == '-CHOOSE-') { $this->form_validation->set_message('validate_dropdown', 'Please choose a valid %s'); return FALSE; } else { return TRUE; } } } ?>
Finally in a view file you have to pass the array containing the category details to the function form_dropdown()
and populate the combo list with category id, name pairs.
And to display the validation error you have to add form_error()
function below the input fields.
View:
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>CodeIgniter Dropdown from Database Example</title> <link href="<?php echo base_url("assets/bootstrap/css/bootstrap.css"); ?>" rel="stylesheet" type="text/css" /> <style> .well { background: none; } </style> </head> <body> <div class="container"> <div class="row"> <div class="col-xs-6 col-xs-offset-3 well"> <?php $attributes = array("name" => "booksform"); echo form_open("demo/index", $attributes);?> <legend>Books Form</legend> <div class="form-group"> <label for="bookname">Book Name</label> <?php $attributes = 'id="bookname" placeholder="Enter Book Name" class="form-control"'; echo form_input('bookname', set_value('bookname'), $attributes); ?> <span class="text-danger"><?php echo form_error('bookname'); ?></span> </div> <div class="form-group"> <label for="category">Category</label> <?php $attributes = 'class="form-control" id="category"'; echo form_dropdown('category', $category, set_value('category'), $attributes); ?> <span class="text-danger"><?php echo form_error('category'); ?></span> </div> <div class="form-group"> <label for="author">Author</label> <?php $attributes = 'id="author" placeholder="Author" class="form-control"'; echo form_input('author', set_value('author'), $attributes); ?> <span class="text-danger"><?php echo form_error('author'); ?></span> </div> <div class="form-group"> <label for="author">ISBN</label> <?php $attributes = 'id="isbn" placeholder="ISBN" class="form-control"'; echo form_input('isbn', set_value('isbn'), $attributes); ?> <span class="text-danger"><?php echo form_error('isbn'); ?></span> </div> <div class="form-group"> <button name="submit" type="submit" class="btn btn-info">Submit</button> <button name="cancel" type="reset" class="btn btn-info">Cancel</button> </div> <?php echo form_close(); ?> </div> </div> </div> </body> </html>
As already said, form_dropdown('category', $category, set_value('category'), $attributes);
function creates a standard html dropdown field.
- And for the function parameters we have used, the first one 'category' is the name of the dropdown field.
- The second parameter $category is the associative array to be populated in the dropdown box.
- The third one is the default value to be selected. Here we used set_value('category') to keep the previous value chosen by the user in case of failed validation.
- The final parameter $attributes includes any of the additional attributes we want to add to the dropdown.
Once everything is set, run the controller file and you can see a form with dropdown list populated with category names like this,

If the user fails to select valid category and submits the form, it will show up error like this,
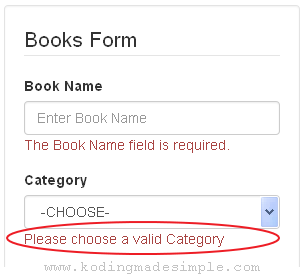
If validation runs successful, you can insert the form details into database which is beyond the scope of this tutorial. But never worry, here this tutorial will teach you how to insert form data into database in codeigniter.
Now that was all about populating dropdown list from database in PHP Codeigniter. I hope you have enjoyed this tutorial. See you in the next post :)
Severity: Notice
ReplyDeleteMessage: Undefined variable: city
Filename: views/insert_view.php
Line Number: 44
Thank you very much. This tutorial is very helpful!
ReplyDelete