Hi! Today I'm going to share a code snippet to create fullscreen html5 video background on websites. Full screen background has become a popular trend of web design and nothing grabs user attention than a good video background. It will make website visitors to stop and take notice thus increasing the time spent on a website. It is a great marketing tactic to get business leads as well. Here we will see how to add full screen background video using HTML5 and CSS. You don't need any plug-ins which is a huge plus of this method.
HTML5 Video:
HTML5 supports so many media objects and Video is one among them. The compatibility of HTML5 Video is very high and most modern browsers support it.
HTML5 has this <video>
element to add videos to a webpage with several configuration attributes like 'autoplay', 'loop' etc. It acts as a placeholder for a video object and supports the 'poster' attribute which will be replaced by the first frame of the video on loading. Therefore it is a good practice to set the poster image as the first frame of a video.
Read: Quick PHP Form Validation using Parsley.js
Create Placeholder for HTML5 Video:
First create a html5 placeholder for the video and add the video source to it.
HTML Markup:
<video id="bgvideo" poster="images/malachite-kingfisher.jpg" playsinline autoplay loop muted> <source src="malachite-kingfisher.webm" type="video/webm"> <source src="malachite-kingfisher.mp4" type="video/mp4"> </video>
Note that without the 'loop' attribute, the video will play only once.
Next add some css to set the video to full screen, center on page and place it behind all the html elements of the web page. Also add a fallback image to the background and this could be the first frame of the video.
CSS:
#bgvideo { background: url('images/malachite-kingfisher.jpg') no-repeat; position: fixed; top: 50%; left: 50%; width: auto; height: auto; min-width: 100%; min-height: 100%; transform: translateX(-50%) translateY(-50%); -moz-transform: translateX(-50%) translateY(-50%); -webkit-transform: translateX(-50%) translateY(-50%); -ms-transform: translateX(-50%) translateY(-50%); transition: 1s opacity; background-size: cover; z-index: -100; }
Read: Detect and Redirect to Mobile Site using PHP and JavaScript
Add Text to the Webpage:
Now let's add some text in the foreground. This could be an excellent place to promote products or services.
HTML Markup:
<div id="bgtext"> <h1>Malachite Kingfisher</h1> <p>One among the rarest birds in the World!!!</p> <p>Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Aenean commodo ligula eget dolor. Aenean massa. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Donec quam felis, ultricies nec, pellentesque eu, pretium quis, sem.</p> <p>Nulla consequat massa quis enim. Donec pede justo, fringilla vel, aliquet nec, vulputate eget, arcu. In enim justo, rhoncus ut, imperdiet a, venenatis vitae, justo. Nullam dictum felis eu pede mollis pretium. Integer tincidunt. Cras dapibus. Vivamus elementum semper nisi. Aenean vulputate eleifend tellus. Aenean leo ligula, porttitor eu, consequat vitae, eleifend ac.</p> <p><a href="#">More Details Here »</a></p> </div>
Simply adding the text won't do. We have to layer the text in front and position it in such a way that it does not hide the background video, but enough to catch user's attention. We can use css to do it.
CSS:
#bgtext { color: white; width: 33%; background: rgba(0,0,0,0.3); font-family: Arial Narrow, sans-serif; font-weight: 100; padding: 28px; margin: 28px; font-size: 19px; float: right; text-align: justify; } h1 { margin-top: 0; font-size: 42px; text-transform: uppercase; letter-spacing: 4px; } a { background:rgba(0,0,0,0.5); color: #FFFFFF; text-decoration: none; padding: 7px; transition: .6s background; display: inline-block; } a:hover { background: rgba(0,0,0,0.9); } #bgtext button { width: 80%; background: rgba(255,255,255,0.23); color: #FFFFFF; margin: 14px auto; font-size: 18px; padding: 6px; display: block; border: none; border-radius: 3px; cursor: pointer; transition: .3s background; } #bgtext button:hover { background: rgba(0,0,0,0.5); }
That's it! We now have a nice full background video layered with text on the front.
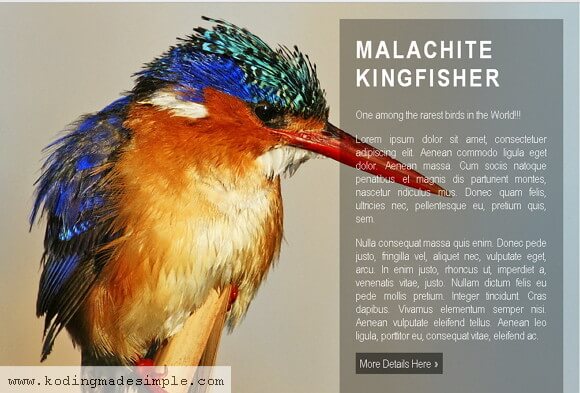
The following is the complete script of our example.
index.html
<!DOCTYPE html> <html> <head> <title>Fullscreen HTML5 Video Background</title> <link href="css/mystyles.css" type="text/css" rel="stylesheet" /> </head> <body> <video id="bgvideo" poster="images/malachite-kingfisher.jpg" playsinline autoplay loop muted> <source src="malachite-kingfisher.webm" type="video/webm"> <source src="malachite-kingfisher.mp4" type="video/mp4"> </video> <div id="bgtext"> <h1>Malachite Kingfisher</h1> <p>One among the rarest birds in the World!!!</p> <p>Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Aenean commodo ligula eget dolor. Aenean massa. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Donec quam felis, ultricies nec, pellentesque eu, pretium quis, sem.</p> <p>Nulla consequat massa quis enim. Donec pede justo, fringilla vel, aliquet nec, vulputate eget, arcu. In enim justo, rhoncus ut, imperdiet a, venenatis vitae, justo. Nullam dictum felis eu pede mollis pretium. Integer tincidunt. Cras dapibus. Vivamus elementum semper nisi. Aenean vulputate eleifend tellus. Aenean leo ligula, porttitor eu, consequat vitae, eleifend ac.</p> <p><a href="#">More Details Here »</a></p> </div> </body> </html>
mystyles.css
body { margin: 0; background: #000000; } #bgvideo { background: url('images/malachite-kingfisher.jpg') no-repeat; position: fixed; top: 50%; left: 50%; width: auto; height: auto; min-width: 100%; min-height: 100%; transform: translateX(-50%) translateY(-50%); transition: 1s opacity; background-size: cover; z-index: -100; } #bgtext { color: white; width: 33%; background: rgba(0,0,0,0.3); font-family: Arial Narrow, sans-serif; font-weight: 100; padding: 28px; margin: 28px; font-size: 19px; float: right; text-align: justify; } #bgtext button { width: 80%; background: rgba(255,255,255,0.23); color: #FFFFFF; margin: 14px auto; font-size: 18px; padding: 6px; display: block; border: none; border-radius: 3px; cursor: pointer; transition: .3s background; } #bgtext button:hover { background: rgba(0,0,0,0.5); } a { background:rgba(0,0,0,0.5); color: #FFFFFF; text-decoration: none; padding: 7px; transition: .6s background; display: inline-block; } a:hover { background: rgba(0,0,0,0.9); } h1 { margin-top: 0; font-size: 42px; text-transform: uppercase; letter-spacing: 4px; }
Read: Currency Conversion in PHP using Google Finance API
With native HTML5 Video and little CSS code we can implement full screen video background on your website. Background video is a powerful feature, but it must be handled effectively. Too long or too short video wouldn't cut it. Also make sure to compress video and keep its size no more than 5 MB so that it takes less bandwidth. I hope you like this tutorial. Please don't forget to share it in social media.
No comments:
Post a Comment