Hey! Today I'm going to show you how to create a simple 5 star rating system using jquery and pure css. Star Rating System is very popular online and is used in most of the websites these days. It allows users to rate contents, comments, reviews etc. and let others know if it is good or bad. There is a wide range of star rating plug-ins available in the market. But I'm going to show you a dead simple way to implement this 5-star rating system without using any plug-ins. All we need is jquery library and css.
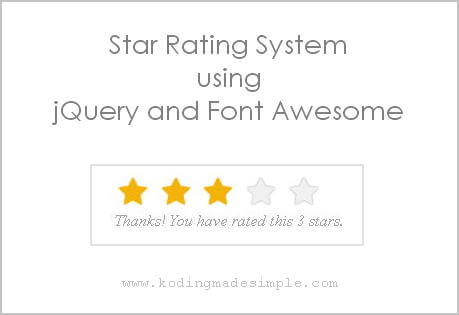
Building jQuery 5-Star Rating System:
To implement the star rating system, we must create a list of stars that looks empty or gray by default. The stars should be highlighted when the mouse hover over them and turn to gray when it exit to a different area of the webpage. And the rating should be counted when the user clicks on the stars and the value should be preserved in a hidden text field.
Read: Create Fullscreen Video Background using HTML5 & CSS
For the stars I'm not going to use images, but the vector icon library, 'Font Awesome'. I have already used it in a tutorial about creating social sharing buttons. The library is easy to use and can create scalable vector icons which you can style with css.
Step 1) Load Required Libraries
We'll need two libraries for building the star rating system, one is jquery and the other is font awesome. Hence we'll first load them in our web page.
<head> <link href="path/to/font-awesome.css" rel="stylesheet" type="text/css" /> <script src="path/to/jquery-1.10.2.js"></script> </head>
Step 2) Creating HTML List of Stars
Next we have to add a set of stars to the page. For that we will use the html list and add the 'star' icon from font-awesome lib.
<input id="star_rating" name="star_rating" type="hidden" /> <ul> <li><i class="fa fa-star fa-fw"></i></li> <li><i class="fa fa-star fa-fw"></i></li> <li><i class="fa fa-star fa-fw"></i></li> <li><i class="fa fa-star fa-fw"></i></li> <li><i class="fa fa-star fa-fw"></i></li> </ul> <div id="msg" name="msg"></div>
In the above markup we have also included a hidden text box to store the user rating and a placeholder to display the notification to users.
Step 3) Add Some CSS Styling
It's time to add some css styles to prettify the plain looking stars.
<style type="text/css"> ul { margin: 0; padding: 0; } li { font-size: 30px; color: #F0F0F0; display: inline-block; text-shadow: 0 0 1px #666666; } #msg { color: #A6A6A6; font-style: italic; } </style>
Okay! The above styles will produce a page with 5 stars like this one,

As I have said before, stars should be highlighted when the user clicks or hover mouse over it. So we need to add css style for highlighting stars in yellow.
.highlight-stars { color: #F4B30A; text-shadow: 0 0 1px #F4B30A; }
Read: How to Store Image into Database with PHP & MySQL
Step 4) jQuery Script
Finally we have to add some jquery functions for the rating system to work. Even though we have stars and everything, nothing will happen unless we have some UI interactive functions. Here we have to write mouse handlers to highlight / un-highlight the stars when the user hover over the stars or leave them. And when they click on the stars the rating should be stored in the hidden field and notified to the user with a message.
<script type="text/javascript"> $(document).ready(function(){ $('li').mouseover(function(){ obj = $(this); $('li').removeClass('highlight-stars'); $('li').each(function(index){ $(this).addClass('highlight-stars'); if(index == $('li').index(obj)){ return false; } }); }); $('li').mouseleave(function(){ $('li').removeClass('highlight-stars'); }); $('li').click(function(){ obj = $(this); $('li').each(function(index){ $(this).addClass('highlight-stars'); $('#star_rating').val((index+1)); $('#msg').html('Thanks! You have rated this ' + (index+1) + ' stars.'); if(index == $('li').index(obj)){ return false; } }); }); $('ul').mouseleave(function(){ if($('#star_rating').val()){ $('li').each(function(index){ $(this).addClass('highlight-stars'); if((index+1) == $('#star_rating').val()){ return false; } }); } }); }); </script>
Done! Now we have everything in place. Run the page in the browser and you'll see 5-star rating system. Hover over the stars and click on them to rate it and you can see a message on the rating you have provided.

Store User Rating into Database:
In case you want to store user rating in the database, you need to do it with the help of some server scripts like php.
Remember we have retained the rating in a hidden text box? You can simply submit the form using ajax and access the rating field value with php.
Here is a rough php script to get the user rating and store it in mysql database.
<?php // store user rating into mysql db $rating = $_POST["star_rating"]; $con = mysqli_connect("localhost", "root", "", "db_rating") or die("Error " . mysqli_error($con)); $sql = "INSERT INTO tbl_rating(rating, timestamp) VALUES('" . $rating . "', '" . @date('Y-m-d H:i:s') . "')"; mysqli_query($con, $sql) or die("Error " . mysqli_error($con)); ?>
Read: How to Lazy Load Images using PHP and JavaScript
That's how you can implement 5 star rating system using jquery, css and font awesome. There's no need to use third party plug-ins to add user rating system to your website. I hope you find this tutorial useful. Please don't forget to share it in social media.
No comments:
Post a Comment