Hi! In today's post, let's see how to filter multidimensional array by key value in php. PHP offers extensive functions to manipulate arrays of which array_filter makes the unimaginable possible. The function allows you to filter elements of an array with custom callbacks.
Multi-dimensional arrays are complicated enough but filtering them by key value is a headache because you have to iterate over each element and look for the specific key values to filter it. But PHP's array_filter() function provides a short and simple way to filter multidimensional array by key and value. You have to use the appropriate callback filter and the rest is cake walk.
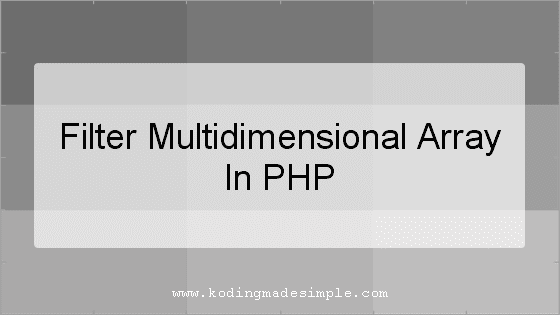
PHP - Filtering Multidimensional Array by Key Value:
Before we start the process, a little intro about the function array_filter()
.
It let you to filter array by value using custom callback. It takes up three parameters, 1. the source, 2. callback function that acts as the conditional filter and 3. flag to define whether a key, value or both should be used for filtering.
Consider the following multi-dimensional array,
Array ( [0] => Array ( [name] => John [email] => john@mydomain.com [dept] => Finance ) [1] => Array ( [name] => Lilly [email] => lilly@mydomain.com [dept] => Sales ) [2] => Array ( [name] => Austin [email] => austin@mydomain.com [dept] => HR ) [3] => Array ( [name] => Whites [email] => whites@mydomain.com [dept] => Finance ) [4] => Array ( [name] => Milan [email] => milan@mydomain.com [dept] => Sales ) )
Now let's see how to filter this array so that the result only contains the elements that have their 'dept => Sales'. Here 'dept' is the key and 'Sales' is the value.
<?php $myarray = array( array("name"=>"John", "email"=>"john@mydomain.com", "dept"=>"Finance"), array("name"=>"Lilly", "email"=>"lilly@mydomain.com", "dept"=>"Sales"), array("name"=>"Austin", "email"=>"austin@mydomain.com", "dept"=>"HR"), array("name"=>"Whites", "email"=>"whites@mydomain.com", "dept"=>"Finance"), array("name"=>"Milan", "email"=>"milan@mydomain.com", "dept"=>"Sales") ); $filter = "Sales"; $new_array = array_filter($myarray, function($var) use ($filter){ return ($var['dept'] == $filter); }); echo "<pre>"; print_r($new_array); ?>
Above code will produce the following output,
Output:
Array ( [1] => Array ( [name] => Lilly [email] => lilly@mydomain.com [dept] => Sales ) [4] => Array ( [name] => Milan [email] => milan@mydomain.com [dept] => Sales ) )
Please note that we are filtering multi-dimensional array which is an array or arrays. So, here each element is itself another array. What the function did is, check each array against the given condition, and keep it in the result if it passes through the condition else remove it.
Filtering Array by Mutiple Key Values:
In the previous example, we have used a single conditional filter, but you can also filter it by multiple values.
Consider the following example,
<?php $myarray = array( array("name"=>"John", "email"=>"john@mydomain.com", "dept"=>"Finance"), array("name"=>"Lilly", "email"=>"lilly@mydomain.com", "dept"=>"Sales"), array("name"=>"Austin", "email"=>"austin@mydomain.com", "dept"=>"HR"), array("name"=>"Whites", "email"=>"whites@mydomain.com", "dept"=>"Finance"), array("name"=>"Milan", "email"=>"milan@mydomain.com", "dept"=>"Sales") ); $filter = array("Lilly", "Whites"); $new_array = array_filter($myarray, function($var) use ($filter){ return in_array($var['name'], $filter); }); echo "<pre>"; print_r($new_array); ?>
Output:
Array ( [1] => Array ( [name] => Lilly [email] => lilly@mydomain.com [dept] => Sales ) [3] => Array ( [name] => Whites [email] => whites@mydomain.com [dept] => Finance ) )
In the above code, we have filtered the sourcearray only to contain those elements (arrays) which have their name in the list we provided. When you provide a separate filtering condition in the callback, you must include 'use' before it.
Read Also:- AJAX Inline Editing Table with PHP, MySQL and AJAX
- Submit Form without Page Refresh with AJAX and PHP
Likewise you can filter the multi dimensional array by key value with php. Filtering one dimensional or simply the array is much simpler that doing it with two or more dimensional. I hope this helps. Please share the post if you find it useful :)
No comments:
Post a Comment