Hi, we'll see how to build contact form in codeigniter application. Contact forms are an essential component of today's websites as they are a means of communication between the end user and the website owner. Earlier I have written a tutorial on creating bootstrap contact form using php and this time I'll walk you step by step to build a contact form in codeigniter. For the form ui design, I'll use bootstrap css but you can use your own stylesheets instead of bootstrap. Learn more about using bootstrap in codeigniter here.
Don't Miss: Basic Database CRUD Operations in CodeIgniter and MySQL for Beginners
Related Read: CodeIgniter Contact Form with MySQL Database Storage Option
Building CodeIgniter Contact Form
Our goal is to create a simple yet effective contact form with proper validation and email functionality. Codeigniter provides a powerful email library to send emails and we are going to use it here.
The codeigniter contact form needs a controller and view file. No need for a model file as there is no database activity. The contact form we are going to build will collect name, email id, subject and message from the user, check for validation errors and send mail if everything is ok else notify the user about error.
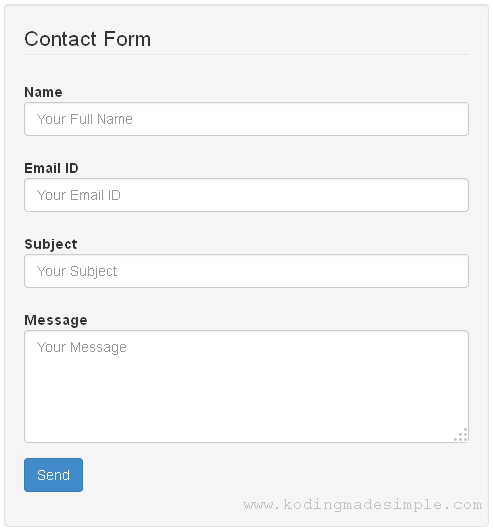
The Controller File
Create a file named "contactform.php" inside application >> controllers folder. Codeigniter provides email library which makes the mail sending task very easier for us.
First let's load the required libraries and helpers in the controller.
<?php class contactform extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form','url')); $this->load->library(array('session', 'form_validation', 'email')); } } ?>
Next create a custom validation function to allow only alphabets and space for the name field.
<?php class contactform extends CI_Controller { ... //custom validation function to accept only alphabets and space input function alpha_space_only($str) { if (!preg_match("/^[a-zA-Z ]+$/",$str)) { $this->form_validation->set_message('alpha_space_only', 'The %s field must contain only alphabets and space'); return FALSE; } else { return TRUE; } } } ?>
Then create the index() function to be called on page load. Here we'll define the form validation rules and run the validation check on form input data.
<?php class contactform extends CI_Controller { ... function index() { //set validation rules $this->form_validation->set_rules('name', 'Name', 'trim|required|xss_clean|callback_alpha_space_only'); $this->form_validation->set_rules('email', 'Emaid ID', 'trim|required|valid_email'); $this->form_validation->set_rules('subject', 'Subject', 'trim|required|xss_clean'); $this->form_validation->set_rules('message', 'Message', 'trim|required|xss_clean'); //run validation on form input if ($this->form_validation->run() == FALSE) { //validation fails $this->load->view('contact_form_view'); } else { // code to send mail } } } ?>
If there is any validation error we re-populate the input data and display the error message below the input fields.
If the validation is successful then we'll move on to send mail.
Add Email Sending Functionality to Contact Form
To send email, first we have to set the email preferences and initialize the 'email' library.
<?php //configure email settings $config['protocol'] = 'smtp'; $config['smtp_host'] = 'ssl://smtp.gmail.com'; $config['smtp_port'] = '465'; $config['smtp_user'] = 'user@gmail.com'; // email id $config['smtp_pass'] = 'password'; // email password $config['mailtype'] = 'html'; $config['wordwrap'] = TRUE; $config['charset'] = 'iso-8859-1'; $config['newline'] = "\r\n"; //use double quotes here $this->email->initialize($config); ?>
The above is the codeigniter email settings for gmail smtp server. Likely you can use any other third party smtp servers other than gmail. Learn more about sending email in codeigniter using smtp protocol.
Next use the form data to send email.
<?php //get the form data $name = $this->input->post('name'); $from_email = $this->input->post('email'); $subject = $this->input->post('subject'); $message = $this->input->post('message'); //set to_email id to which you want to receive mails $to_email = 'user@gmail.com'; //send mail $this->email->from($from_email, $name); $this->email->to($to_email); $this->email->subject($subject); $this->email->message($message); if ($this->email->send()) { // mail sent $this->session->set_flashdata('msg','<div class="alert alert-success text-center">Your mail has been sent successfully!</div>'); redirect('contactform/index'); } else { //error $this->session->set_flashdata('msg','<div class="alert alert-danger text-center">There is error in sending mail! Please try again later</div>'); redirect('contactform/index'); } ?>
The variable $to_mail is the email-address you want to receive the mails.
The method $this->email->send()
will return a boolean value TRUE/FALSE depending on if mail is sent or not. Using it inside if statement, we set success or failure message to notify the user. Here we use $this->session->set_flashdata()
to store the message and display it when the page is redirected.
Complete Code for the Contact Form Controller:
<?php class contactform extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form','url')); $this->load->library(array('session', 'form_validation', 'email')); } function index() { //set validation rules $this->form_validation->set_rules('name', 'Name', 'trim|required|xss_clean|callback_alpha_space_only'); $this->form_validation->set_rules('email', 'Emaid ID', 'trim|required|valid_email'); $this->form_validation->set_rules('subject', 'Subject', 'trim|required|xss_clean'); $this->form_validation->set_rules('message', 'Message', 'trim|required|xss_clean'); //run validation on form input if ($this->form_validation->run() == FALSE) { //validation fails $this->load->view('contact_form_view'); } else { //get the form data $name = $this->input->post('name'); $from_email = $this->input->post('email'); $subject = $this->input->post('subject'); $message = $this->input->post('message'); //set to_email id to which you want to receive mails $to_email = 'user@gmail.com'; //configure email settings $config['protocol'] = 'smtp'; $config['smtp_host'] = 'ssl://smtp.gmail.com'; $config['smtp_port'] = '465'; $config['smtp_user'] = 'user@gmail.com'; $config['smtp_pass'] = 'password'; $config['mailtype'] = 'html'; $config['charset'] = 'iso-8859-1'; $config['wordwrap'] = TRUE; $config['newline'] = "\r\n"; //use double quotes //$this->load->library('email', $config); $this->email->initialize($config); //send mail $this->email->from($from_email, $name); $this->email->to($to_email); $this->email->subject($subject); $this->email->message($message); if ($this->email->send()) { // mail sent $this->session->set_flashdata('msg','<div class="alert alert-success text-center">Your mail has been sent successfully!</div>'); redirect('contactform/index'); } else { //error $this->session->set_flashdata('msg','<div class="alert alert-danger text-center">There is error in sending mail! Please try again later</div>'); redirect('contactform/index'); } } } //custom validation function to accept only alphabets and space input function alpha_space_only($str) { if (!preg_match("/^[a-zA-Z ]+$/",$str)) { $this->form_validation->set_message('alpha_space_only', 'The %s field must contain only alphabets and space'); return FALSE; } else { return TRUE; } } } ?>
The View File
Next we have to create view file for codeigniter contact form. Create a file named "contact_form_view.php" inside application >> views folder. This file will holds up the html markup mixed with bootstrap css classes to render the form. Using the bootstrap form classes will properly align the form elements with required padding. Here is the complete markup for our contact form.
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>CodeIgniter Contact Form Example</title> <!--load bootstrap css--> <link href="<?php echo base_url("path/to/bootstrap/bootstrap.css"); ?>" rel="stylesheet" type="text/css" /> </head> <body> <div class="container"> <div class="row"> <div class="col-md-6 col-md-offset-3 well"> <?php $attributes = array("class" => "form-horizontal", "name" => "contactform"); echo form_open("contactform/index", $attributes);?> <fieldset> <legend>Contact Form</legend> <div class="form-group"> <div class="col-md-12"> <label for="name" class="control-label">Name</label> </div> <div class="col-md-12"> <input class="form-control" name="name" placeholder="Your Full Name" type="text" value="<?php echo set_value('name'); ?>" /> <span class="text-danger"><?php echo form_error('name'); ?></span> </div> </div> <div class="form-group"> <div class="col-md-12"> <label for="email" class="control-label">Email ID</label> </div> <div class="col-md-12"> <input class="form-control" name="email" placeholder="Your Email ID" type="text" value="<?php echo set_value('email'); ?>" /> <span class="text-danger"><?php echo form_error('email'); ?></span> </div> </div> <div class="form-group"> <div class="col-md-12"> <label for="subject" class="control-label">Subject</label> </div> <div class="col-md-12"> <input class="form-control" name="subject" placeholder="Your Subject" type="text" value="<?php echo set_value('subject'); ?>" /> <span class="text-danger"><?php echo form_error('subject'); ?></span> </div> </div> <div class="form-group"> <div class="col-md-12"> <label for="message" class="control-label">Message</label> </div> <div class="col-md-12"> <textarea class="form-control" name="message" rows="4" placeholder="Your Message"><?php echo set_value('message'); ?></textarea> <span class="text-danger"><?php echo form_error('message'); ?></span> </div> </div> <div class="form-group"> <div class="col-md-12"> <input name="submit" type="submit" class="btn btn-primary" value="Send" /> </div> </div> </fieldset> <?php echo form_close(); ?> <?php echo $this->session->flashdata('msg'); ?> </div> </div> </div> </body> </html>
We have used codeigniter's form_error()
method below each input field to display the validation errors. Simultaneously we use set_value()
method to repopulate the form input in case of validation errors. We display these errors in red color by using bootstrap's "text-danger" class element.
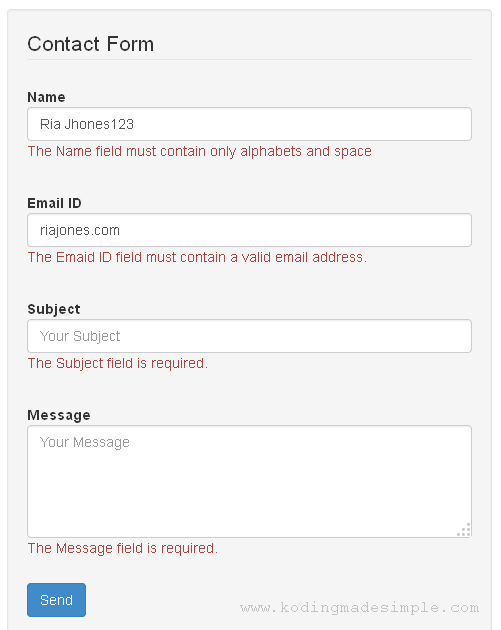
Towards the bottom of the form, we echoed flashdata()
message to display the success or failure message to the user. The success message will be displayed inside a green color box and failure message in a red color box.
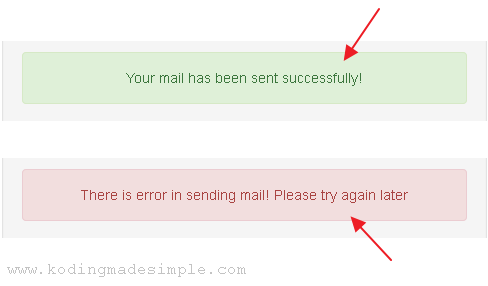
I hope now you have a good understanding of building contact form in codeigniter.
Read Also: How to Create Registration Form in CodeIgniter with Email Confirmation
Don't Miss: How to Create Login Form with Sessions in CodeIgniter and MySQL
I was lost how to implement contact form with smtp, this would hep me for sure
ReplyDeleteGlad you find it useful :)
DeleteSaved me a lot of time to figure out how it works with this framework (normally I am using Symfony2), so thanks for this very well written tutorial!
ReplyDeleteManuel
Glad you liked it Manuel...Cheers!!!
DeleteWhy you didn't provide download file ?
ReplyDeletewhat is it, i'm still don't get it
$config['smtp_user'] = 'user@gmail.com'; // email id
$config['smtp_pass'] = 'password'; // email password
Hi,
Delete'smtp_user' should be the email id from the which you want to send the mail.
'smtp_pass' is the password of the email id you use for 'smtp_user'.
Example: if you want to use gmail server for sending mail, then 'smtp_user' should be one of your gmail accound id and 'smtp_pass' is the password of the gmail account.
Hope this helps.
Hello ,
ReplyDeleteThank you for this well written tutorial.
It helped me a lot to understand and implement email functionality.
It didn't work for me. Do you have the complete downloadable files?
ReplyDeleteI'm having trouble at this point.
ReplyDeleteA PHP Error was encountered
Severity: Warning
Message: fsockopen() [function.fsockopen]: unable to connect to ssl://smtp.googlemail.com:465 (Connection timed out)
Filename: libraries/Email.php
Line Number: 1949
Backtrace:
me too , did you find a solution for this?
DeleteHi Valli,
ReplyDeleteI LOVE your CodeIgniter how-to's. They've filled alot of the voids I've had with my overall comprehension of a MVC-structured framework, all while providing valuable information in the process. Keep up the AWESOME work!!!
In reference to this particular how-to...could you detail how one could create this very same form (and associated email functionality), but display it in a bootstrap modal view and keep all form validation and status messages contained within this same view without the page reloading? Thanks!!!
dS.
Any update to this...?
DeleteHi,
DeleteSorry about my delayed response. For readers benefit, I have created a separate tutorial for modal contact form. Please check out this url.
http://www.kodingmadesimple.com/2015/09/codeigniter-modal-contact-form-jquery-ajax-validation-example.html
Hope that helps. Please let me know if you have any queries.
Cheers.
how send to email without use gmail,
ReplyDeleteHi, if you are using any other third party mail service, then get the smtp host name from your service provider and change these three email settings,
Delete$config['smtp_host'] = 'ssl://smtp.gmail.com'; // change this to your host name
$config['smtp_user'] = 'user@gmail.com'; // change to your third party email-id
$config['smtp_pass'] = 'password'; // password of the above email-id
And it should work!
Cheers.
Thank you for making such a resourceful tutorial free. It teaches a lot more on CodeIgniter
ReplyDeleteThanks for your kind words!!! Glad you liked it.
DeleteCheers :-)
Which emai and password I have to provide for
ReplyDelete" $config['smtp_user'] = 'user@gmail.com'; // email id
$config['smtp_pass'] = 'password'; // email password " ?
Hi! It should be the email and password to which you want to receive mails.
DeleteCheers.
Class names must start with an uppercase letter.
ReplyDeleteclass Contactform extends CI_Controller not-> class contactform extends CI_Controller
Hi.
ReplyDeletehow do you access the form after ?
Hi Sir,
ReplyDeleteI has problem regarding email using checkbox. I select the email in the checkbox but when submit it not sent to the email. Please help me.
Here the code in view:
Vendor to request quotation
$value) { ?>
< class="radio">
< type="checkbox" name="paramVendorId[]" class="icheck" value="id?>"> name?> (email?>)
In controller:
public function sendEmailNotificationToVendor($product_name, $selectedVendor) {
$vendors = $this->model_db->vendorListSelected($selectedVendor);
foreach ($vendors as $key => $value) {
$this->email->from('temp@rkpintas.com.my', 'eTEM System');
$this->email->to($value->email);
$this->email->subject('Request for quotation');
$this->email->message('Hi. Kindly submit your quotation on this item (' . $product_name . '). You may login using your (this) email as username and password here: http://www.rkpintas.com.my/temp/pub/login. Thank You.');
$this->email->send();
}
}
In model_db:
public function vendorListSelected($selectedVendor) {
$sql = "SELECT * FROM `vendor` WHERE `id` IN (" . $selectedVendor . ") ORDER BY `id` ASC";
$query = $this->db->query($sql);
return $query->result();
}
can i get the css folder for contact form ?
ReplyDeleteThe code cant send info to my email address...it excute else statement
ReplyDelete"There is error in sending mail! Please try again later" please can one help me