PHP Codeigniter framework provides a good number of libraries required for building a website with codeigniter and pagination library is one among them. For those who are not familiar with the term pagination, this refers to the set of numbered links that allows you to navigate from page to page to view a large set of data. For example let's say you want to fetch and display some data from database and it contains several hundreds of records. In that case pagination will be very useful to display the database resultset in chunks and let the user to skim through several page links (similar to google search results).
However the pagination links in codeigniter are plain looking in nature and a little customization to codeigniter pagination doesn't hurt. Here we'll see in this tutorial, how to style codeigniter pagination links with twitter bootstrap styles.
Implement Twitter Bootstrap Styles in CodeIgniter Pagination
We'll see an example below to fetch and display the list of department names and their department head in a neat table view along with pagination links at the bottom.
MySQL Database Example
As an example I'll use this below 'employee' database for this tutorial.
SET SQL_MODE="NO_AUTO_VALUE_ON_ZERO"; -- -- Database: `employee` -- CREATE TABLE IF NOT EXISTS `tbl_dept` ( `int_id` int(4) NOT NULL AUTO_INCREMENT, `var_dept_name` varchar(50) NOT NULL, `int_hod` int(5) NOT NULL, KEY `int_id` (`int_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ; CREATE TABLE IF NOT EXISTS `tbl_emp` ( `int_id` int(5) NOT NULL AUTO_INCREMENT, `var_emp_name` varchar(50) NOT NULL, `int_dept_id` int(4) NOT NULL, PRIMARY KEY (`int_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
The Model ('models/department_model.php')
First we create a model file with a method to fetch and return the entire department details along with the head of the department employee name.
<?php class department_model extends CI_Model{ function __construct() { // Call the Model constructor parent::__construct(); } //fetch department details from database function get_department_list($limit, $start) { $sql = 'select var_dept_name, var_emp_name from tbl_dept, tbl_emp where tbl_dept.int_hod = tbl_emp.int_id order by var_dept_name limit ' . $start . ', ' . $limit; $query = $this->db->query($sql); return $query->result(); } } ?>
The Controller ('controllers/department.php')
Next create the controller file and load the codeigniter pagination library and the department model file which we created earlier in the constructor method. Then create the index() function where we configure the behavior of the pagination library with the $config[]
array and create the pagination links to be displayed at the bottom of the department table in the view file.
<?php class department extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper('url'); $this->load->database(); $this->load->library('pagination'); //load the department_model $this->load->model('department_model'); } public function index() { //pagination settings $config['base_url'] = site_url('department/index'); $config['total_rows'] = $this->db->count_all('tbl_dept'); $config['per_page'] = "5"; $config["uri_segment"] = 3; $choice = $config["total_rows"] / $config["per_page"]; $config["num_links"] = floor($choice); //config for bootstrap pagination class integration $config['full_tag_open'] = '<ul class="pagination">'; $config['full_tag_close'] = '</ul>'; $config['first_link'] = false; $config['last_link'] = false; $config['first_tag_open'] = '<li>'; $config['first_tag_close'] = '</li>'; $config['prev_link'] = '«'; $config['prev_tag_open'] = '<li class="prev">'; $config['prev_tag_close'] = '</li>'; $config['next_link'] = '»'; $config['next_tag_open'] = '<li>'; $config['next_tag_close'] = '</li>'; $config['last_tag_open'] = '<li>'; $config['last_tag_close'] = '</li>'; $config['cur_tag_open'] = '<li class="active"><a href="#">'; $config['cur_tag_close'] = '</a></li>'; $config['num_tag_open'] = '<li>'; $config['num_tag_close'] = '</li>'; $this->pagination->initialize($config); $data['page'] = ($this->uri->segment(3)) ? $this->uri->segment(3) : 0; //call the model function to get the department data $data['deptlist'] = $this->department_model->get_department_list($config["per_page"], $data['page']); $data['pagination'] = $this->pagination->create_links(); //load the department_view $this->load->view('department_view',$data); } } ?>
As you can see in the above code, we have integrated bootstrap's '.pagination' class to codeigniter pagination links. Using the '.active' css class will highlight the active page in the links.
The View ('views/department_view.php')
Finally create view file to display the department list in a neat striped table with bootstrap pagination links at the bottom.
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>PHP CodeIgniter Pagination with Bootstrap Styles | Example</title> <!--link the bootstrap css file--> <link rel="stylesheet" href="<?php echo base_url("bootstrap/css/bootstrap.css"); ?>"> </head> <body> <div class="container"> <div class="row"> <div class="col-md-12"> <table class="table table-striped table-hover"> <thead> <tr> <th>#</th> <th>Department Name</th> <th>Head of Department</th> </tr> </thead> <tbody> <?php for ($i = 0; $i < count($deptlist); ++$i) { ?> <tr> <td><?php echo ($page+$i+1); ?></td> <td><?php echo $deptlist[$i]->var_dept_name; ?></td> <td><?php echo $deptlist[$i]->var_emp_name; ?></td> </tr> <?php } ?> </tbody> </table> </div> </div> <div class="row"> <div class="col-md-12 text-center"> <?php echo $pagination; ?> </div> </div> </div> </body> </html>
We have used bootstrap table styles such as '.table', '.table-striped', '.table-hover' to display the department list in a neat format and at the bottom with pagination links.
Open the controller in the browser and you get the department details displayed like this.
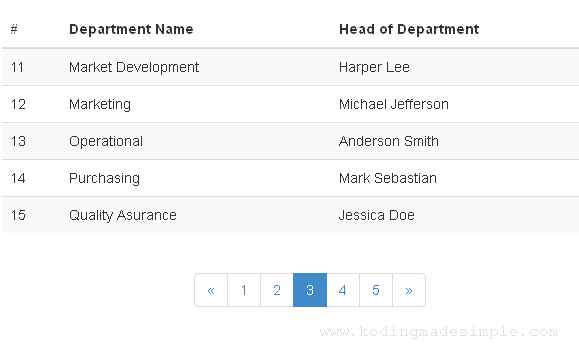
- How to integrate Twitter Bootstrap with PHP CodeIgniter
- How to Create Login Form in CodeIgniter and Bootstrap with Sessions
I hope now you get a better understanding of integrating twitter bootstrap styles with codeigniter pagination links.
Related Read: CodeIgniter-Bootstrap Pagination with Search Query Filter
Last Modified: Nov-10-2017
nicely done!
ReplyDeleteWOW. This is good one.
ReplyDeleteIt works.
Special thanks to you to share this post...........
Great you find this useful :-)
Deletegreat, its very clear
ReplyDeleteGlad you liked it...Cheers :-)
DeleteVery very nice..
ReplyDeleteI'm glad you liked it! Cheers...Valli
DeleteVery very nice and clear tutorial; thanks; keep posting plz;
ReplyDeleteI'm glad you liked it! Thanks for your support.
DeleteCheers
Valli.
Hello
ReplyDeleteI want to display a limited no. of page links, say 5 out of 10 links
so lets say user can right now see following links
prev, 1(selected), 2, 3, 4, 5... next
user clicks on, say 4, now he sees
prev... 3, 4(selected), 5, 6, 7...next
now he clicks on 7
prev... 6, 7(selected), 8, 9, 10...next
How can I do this in code igniter?
Thanks a lot...!!!
ReplyDeleteYour tutorial really help my work here...
Glad you find it useful! Cheers:)
DeleteI get a problem in redirection from 1 to 2.
ReplyDeletefor example i have 127.0.0.1/ci/users so when i wanna go to link 2 i fet 127.0.0.1/2.
could you help me?
I get a problem in redirection from 1 to 2.
ReplyDeletefor example i have 127.0.0.1/ci/users so when i wanna go to link 2 i fet 127.0.0.1/2.
could you help me?
I have a problem when i wanna navigate betweene links, for example: at first i have 127.0.0.1/CI/users and when i click into 2 i get 127.0.0.1/2. i know it's a redirect problem, but how can i solve it?
ReplyDeletei like it bro .thanks !
ReplyDeleteThank you very nicely done ;)
ReplyDeletenice tutorial
ReplyDeleteWelcome:)
Deletewhen i click first number of the pagination it trow 404 Page Not Found
ReplyDeleteThe page you requested was not found.
http://www.pixelsolutionbd.com/
ReplyDeleteWordpress
PHP
Plugin development
Thank you
ReplyDeleteVery nice :) you are awasome
ReplyDeleteIt is not necessary to create database before creating model, controller and view for pagination in Codeigniter (https://www.cloudways.com/blog/pagination-in-codeigniter/ ). It can also be done without creating any database.
ReplyDelete