PHP reCAPTCHA Tutorial: This post will show you how to use google recaptcha in php form to protect your website from bots and spammers. Old recaptcha requires users to fill out a textbox with text found in an image and to be frank this method is not so user friendly. But Google has released a new recaptcha in which user has to just tick a checkbox and be done with it. It is very effective in controlling spam without compromising user experience.
Google has named this new recaptcha as "No CAPTCHA reCAPTCHA" which is meant to be gentle on humans and hard on bots.
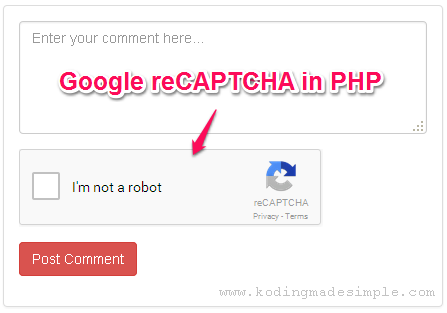
How to Use Google reCAPTCHA in PHP?
Implementing Google reCAPTCHA in php form is quite easy but before doing so you have to first register site (domain name) with Google and get site & secret keys.
Go to https://www.google.com/recaptcha/admin and register the site in which you want to use recaptcha.
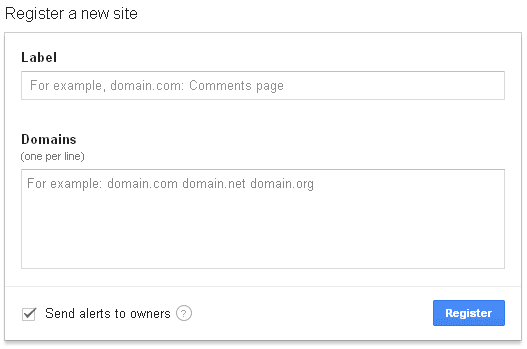
If you want to test recaptcha api from localhost, enter 'localhost' in the Domains box and click on 'Register' button.
You will be provided with two keys; one is the 'Site Key' which is used to display recaptcha widget. And the second one is the 'Secret Key' to confirm user response. You'll need these two to integrate recaptcha in your website.
Create PHP Form:
<!doctype html> <html> <head> <title>Implementing Google reCaptcha in PHP Demo</title> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" type="text/css" rel="stylesheet" /> </head> <body> <div class="container" style="margin-top: 30px;"> <div class="col-xs-6 col-xs-offset-3"> <div class="panel panel-default"> <div class="panel-body"> <form action="recaptcha_demo.php" method="post" name="comment-form" > <div class="form-group"> <textarea name="comment" placeholder="Enter your comment here..." class="form-control" rows="4" required></textarea> </div> <div class="form-group"> <input type="submit" name="submit" value="Post Comment" class="btn btn-danger"> </div> </form> </div> </div> </div> </div> </body> </html>
Above markup creates a simple comment form with a comment box and a submit button. Next load javascript library for recaptcha api in your form.
<!-- load recaptcha api --> <script src='https://www.google.com/recaptcha/api.js'></script>
Then add this below recaptcha widget before Submit button in the form.
<div class="form-group"> <div class="g-recaptcha" data-sitekey="<?php echo $site_key; ?>"></div> </div>
Note: You can rather provide the site key directly in its place without using php variable.
Then we need php script to validate recaptcha and process the comment form data.
<?php $site_key = '***************'; // change this to yours $secret_key = '***************'; // change this to yours if (isset($_POST['submit'])) { if(isset($_POST['g-recaptcha-response'])) { $api_url = 'https://www.google.com/recaptcha/api/siteverify?secret=' . $secret_key . '&response='.$_POST['g-recaptcha-response']; $response = @file_get_contents($api_url); $data = json_decode($response, true); if($data['success']) { $comment = $_POST['comment']; // process comment here $success = true; } else { $success = false; } } else { $success = false; } } ?>
Now run the php form, provide comment and tick recaptcha box and submit it.
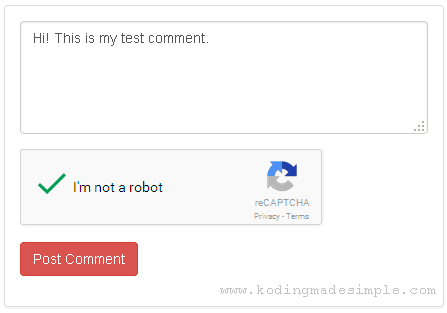
If recaptcha validation went right and rest is ok, you will be shown with a success message.
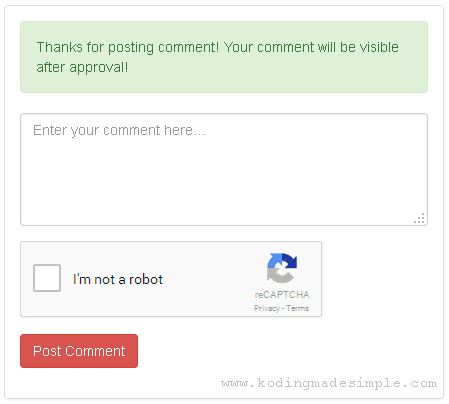
If you fail to complete captcha verification then you'd get error like this,
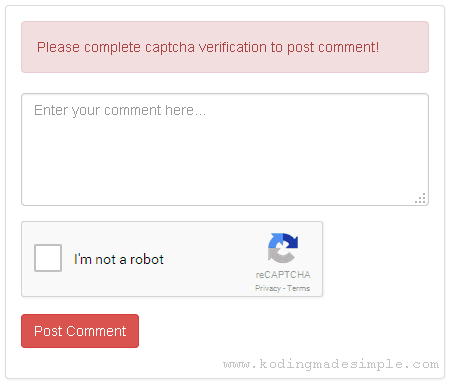
Here's the complete code for php recaptcha example.
recaptcha_demo.php
<?php $site_key = '***************'; // change this to yours $secret_key = '***************'; // change this to yours if (isset($_POST['submit'])) { if(isset($_POST['g-recaptcha-response'])) { $api_url = 'https://www.google.com/recaptcha/api/siteverify?secret=' . $secret_key . '&response='.$_POST['g-recaptcha-response']; $response = @file_get_contents($api_url); $data = json_decode($response, true); if($data['success']) { $comment = $_POST['comment']; // process comment here $success = true; } else { $success = false; } } else { $success = false; } } ?> <!doctype html> <html> <head> <title>Implementing Google reCaptcha in PHP Demo</title> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" type="text/css" rel="stylesheet" /> </head> <body> <div class="container" style="margin-top: 30px;"> <div class="col-xs-6 col-xs-offset-3"> <div class="panel panel-default"> <div class="panel-body"> <?php if (isset($success)) { if ($success==true) { ?> <div class="alert alert-success"><?php echo "Thanks for posting comment! Your comment will be visible after approval!"; ?></div> <? } else { ?> <div class="alert alert-danger"><?php echo "Please complete captcha verification to post comment!"; ?></div> <?php } } ?> <form action="recaptcha_demo.php" method="post" name="comment-form" > <div class="form-group"> <textarea name="comment" placeholder="Enter your comment here..." class="form-control" rows="4" required></textarea> </div> <div class="form-group"> <div class="g-recaptcha" data-sitekey="<?php echo $site_key; ?>"></div> </div> <div class="form-group"> <input type="submit" name="submit" value="Post Comment" class="btn btn-danger"> </div> </form> </div> </div> </div> </div> <!-- load recaptcha api --> <script src='https://www.google.com/recaptcha/api.js'></script> </body> </html>
Must Read: jQuery PHP Image Gallery from Folder using Bootstrap
Don't Miss: AJAX Username Availability Check using PHP and MySQL
That explains about integrating google recaptcha in php. I hope you find this php recaptcha tutorial useful. Please share your thoughts through comments.
thank you for such a great article with us. hope it will be much useful for us. please keep on updating..
ReplyDelete