Hi! Let's see How to integrate reCAPTCHA in Codeigniter Application. This will help protect your codeigniter websites from spam and bots. Google reCAPTCHA effectively blocks out bot access but the old recaptcha is not very user-friendly. It requires you to fill out a textbox with the characters you see on an image. Not to mention the captcha text is difficult to read even for humans. Later a new version of reCAPTCHA named 'I'm not a robot' has been released by Google. It is meant to be gentle on humans and hard on bots.
In this new recaptcha all you have to do is to tick a checkbox and be done. In fact it's much more effective in controlling spam and improves user-experience without compromise.
Also Read:How to Integrate reCAPTCHA in CodeIgniter?
In order to integrate Google reCAPTCHA in your Codeigniter application, you have to first register the specific site with Google. After registration you will be provided with two keys namely Site & Secret key.
The 'Site Key' should be used for displaying recaptcha widget on your site and 'Secret Key' for captcha verification.
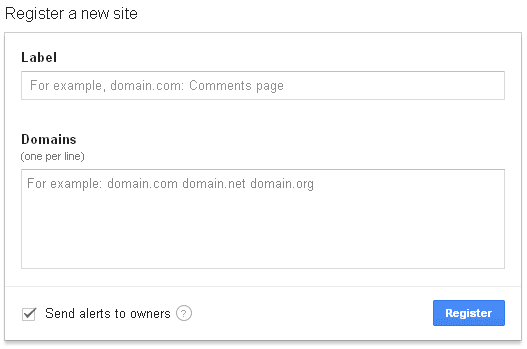
For testing purpose Google let you to run recaptcha from localhost. I'll show you how to do this.
- Visit here and register the codeigniter site you wish to integrate recaptcha.
- You have to enter 'Label' and 'Domains' details in the registration form.
- Label is just for your understanding so you can simply provide site name here.
- In 'Domains' box enter the domain name. To test recaptcha from local machine enter 'localhost'.
Click on 'Register' and you will be provided with a set of keys. Save the keys in a text file. You will need them for implementing captcha code.
Adding reCAPTCHA to Form:
For using recaptcha in a form load the api library first.
<script src='https://www.google.com/recaptcha/api.js'></script>
Then add this snippet to display recaptcha widget wherever you want.
<div class="g-recaptcha" data-sitekey="your-site-key"></div>
For better understanding let us create user feedback form in codeigniter and add recaptcha to it. You need a controller and view file for this.
The View ('recaptcha_demo_view.php'):
The view file contains a feedback form with recaptcha verification. The form will be processed only when user verifies captcha.
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>CodeIgniter Recaptcha Demo</title> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" type="text/css" rel="stylesheet" /> <script src='https://www.google.com/recaptcha/api.js'></script> </head> <body> <div class="container" style="margin-top: 20px;"> <div class="col-xs-6 col-xs-offset-3 well" style="background:none;"> <?php echo form_open("recaptcha_demo/index"); ?> <div class="form-group"> <textarea name="feedback" placeholder="Your feedback..." class="form-control" rows="4"><?php echo set_value('feedback'); ?></textarea> <span class="text-danger"><?php echo form_error('feedback'); ?></span> </div> <div class="form-group"> <div class="g-recaptcha" data-sitekey="your-site-key"></div> <span class="text-danger"><?php echo form_error('g-recaptcha-response'); ?></span> </div> <div class="form-group"> <button type="submit" name="submit" class="btn btn-lg btn-danger">Send Feedback</button> </div> <?php echo form_close(); ?> <?php echo $this->session->flashdata('msg'); ?> </div> </div> </body> </html>
The Controller ('recaptcha_demo.php')
In controller we have an index() function to which the form is submitted and a custom callback to verify captcha input.
<?php class recaptcha_demo extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form','url')); $this->load->library(array('session', 'form_validation')); } function index() { $this->form_validation->set_rules('feedback', 'Feedback', 'required'); $this->form_validation->set_rules('g-recaptcha-response', 'g-recaptcha-response', 'callback_captcha_validation'); if ($this->form_validation->run() == FALSE) { $this->load->view('recaptcha_demo_view'); } else { $this->session->set_flashdata('msg','<div class="alert alert-success text-center">Thanks for your Feedback! We got it!!!</div>'); redirect('recaptcha_demo/index'); } } function captcha_validation() { $secret_key = 'your-secret-key'; // change this to yours $url = 'https://www.google.com/recaptcha/api/siteverify?secret=' . $secret_key . '&response='.$_POST['g-recaptcha-response']; $response = @file_get_contents($url); $data = json_decode($response, true); if($data['success']) { return true; } else { $this->form_validation->set_message('captcha_validation', 'Please confirm you are human'); return false; } } }
Done! Now run the file and you will see a nice user feedback form with recaptcha at the bottom.
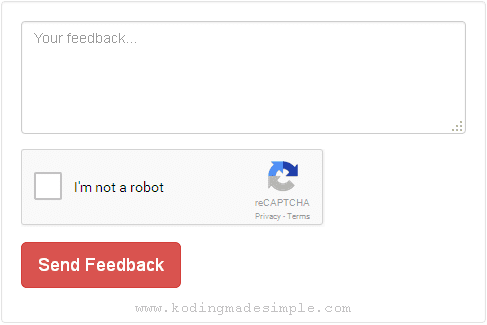
Fill-in your feedback, tick the recaptcha checkbox and click Send Feedback.

If form validation goes right you will be shown with a success message like this.

On the other hand if you don't confirm captcha and submit the form, you will be asked to confirm captcha like this.

You can also implement recaptcha in php form, just check the tutorial.
Also Read:
- CodeIgniter Login Form with MySQL and Bootstrap
- CodeIgniter Registration Form with Email Confirmation
- CodeIgniter Contact Form with Email Functionality
That's it! You have successfully integrated Google reCAPTCHA in CodeIgniter. Implement recaptcha in codeigniter applications you build and stop spammers bombarding your site. Please make sure to use site & secret key with yours for this to work. If you have any trouble using this code please let me know in comments section.
No comments:
Post a Comment