Hi! In this tutorial I'm going to show you how to get youtube video details like title, description, thumbnail image etc from a video url using php script. With the help of Google's YouTube Data API you can fetch information about youtube videos. In general each youtube video will have a specific 'ID' associated to it. To retrieve data about a video you have to pass this video id while making the api call. And the api in turn returns back the video data as json response.
YouTube API is not only helpful to fetch basic video info but also let you create playlists, channels, implement youtube search and much more.
Get YouTube Data API Key
YouTube Data API is free to use but first you must get access to it. You need a Google account to get api access and is provided in the form of an api key.
1. To get api-key, go to https://console.developers.google.com and login with your Google account.
2. Once you are in, create a new project and click on 'ENABLE API' link at the top.
3. Now scroll down to 'Youtube APIs' section and select 'Youtube Data API'.

4. Then click on the 'ENABLE' link at the top-right side of the page to enable youtube data api for your account.
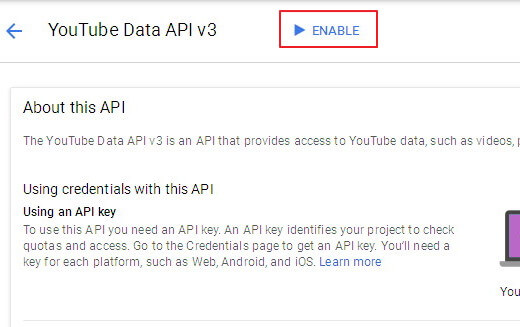
5. Once enabled, select 'Credentials' on the left-pane. Under credentials click on 'Create credentials' button and choose 'API key' in the dropdown.

7. Your api key will be generated and shown in a popup. Save the key to a text file for later use.

Done! Now we have the key to access youtube api. Let's move on to the coding part.
Fetching YouTube Video Information
In general YouTube videos share a common url structure and they look like this.
https://www.youtube.com/watch?v=VIDEO_ID
Where 'VIDEO_ID' represents the individual video id.
You have to take this video id from the youtube video url and use it to fetch details.
Like I already said, to fetch video information you have to call the youtube api. And you must also send the corresponding 'VIDEO_ID' and 'parts' of the information you need to retrieve. Following is the php script to do it.
PHP Script to Get YouTube Video Details
<?php $videoid = 'H4Jx4oefSjw'; // change this $apikey = 'API_KEY'; // change this $json = file_get_contents('https://www.googleapis.com/youtube/v3/videos?id=' . $videoid . '&key=' . $apikey . '&part=snippet'); $data = json_decode($json, true); echo "<pre>"; print_r($data); ?>
The above snippet will produce an output like this.
Array ( [kind] => youtube#videoListResponse [etag] => "m2yskBQFythfE4irbTIeOgYYfBU/YJEU8MCit4Jt-7o2NPm81zgElAQ" [pageInfo] => Array ( [totalResults] => 1 [resultsPerPage] => 1 ) [items] => Array ( [0] => Array ( [kind] => youtube#video [etag] => "m2yskBQFythfE4irbTIeOgYYfBU/Sx66nqOT7ouBnClI9ImzyEA1n90" [id] => H4Jx4oefSjw [snippet] => Array ( [publishedAt] => 2013-08-01T04:12:47.000Z [channelId] => UCj4LfrxdH7TnUZlyHOKgL5A [title] => 01. Programming in Objective-C - Introduction [description] => Ever wondered what Objective-C actually is? Historically, it was the first attempt to make C objectified. C++ came later. NextStep, and now OSX, popularized this very powerful language. So sit back, as Yari D`Areglia takes you through the first steps in getting started with this language. [thumbnails] => Array ( [default] => Array ( [url] => https://i.ytimg.com/vi/H4Jx4oefSjw/default.jpg [width] => 120 [height] => 90 ) [medium] => Array ( [url] => https://i.ytimg.com/vi/H4Jx4oefSjw/mqdefault.jpg [width] => 320 [height] => 180 ) [high] => Array ( [url] => https://i.ytimg.com/vi/H4Jx4oefSjw/hqdefault.jpg [width] => 480 [height] => 360 ) [standard] => Array ( [url] => https://i.ytimg.com/vi/H4Jx4oefSjw/sddefault.jpg [width] => 640 [height] => 480 ) [maxres] => Array ( [url] => https://i.ytimg.com/vi/H4Jx4oefSjw/maxresdefault.jpg [width] => 1280 [height] => 720 ) ) [channelTitle] => Dilan Damith Prasanga I.G. [tags] => Array ( [0] => Objective-C (Programming Language) [1] => Programming In Objective-C [2] => Programming Language (Literary Genre) ) [categoryId] => 27 [liveBroadcastContent] => none [localized] => Array ( [title] => 01. Programming in Objective-C - Introduction [description] => Ever wondered what Objective-C actually is? Historically, it was the first attempt to make C objectified. C++ came later. NextStep, and now OSX, popularized this very powerful language. So sit back, as Yari D`Areglia takes you through the first steps in getting started with this language. ) ) ) ) )
As you can see, we have decoded the json response to php array and it contains details like video title, description, thumbnail urls, view count, channel id and much more. Now you must parse this array to extract individual data like title, description etc.
To Get YouTube Video Title,
<?php echo $data['items'][0]['snippet']['title']; ?>
To Get YouTube Video Description,
<?php echo $data['items'][0]['snippet']['description']; ?>
To Get YouTube Video Thumbnail URL,
<?php $data['items'][0]['snippet']['thumbnails']['default']['url']; ?>
YouTube stores four different types of thumbnails at various resolutions for a single video. Above, I have taken the default thumbnail image url.
Once you've got the video info, use it as per your liking. Here is a simple example in which I have displayed video details in a web page.
Example
<?php echo '<h1>Title: ' . $data['items'][0]['snippet']['title'] . '</h1>'; echo '<img src="' . $data['items'][0]['snippet']['thumbnails']['default']['url'] . '" style="float:right;"/>'; echo '<p>' . $data['items'][0]['snippet']['description'] . '</p>'; ?>
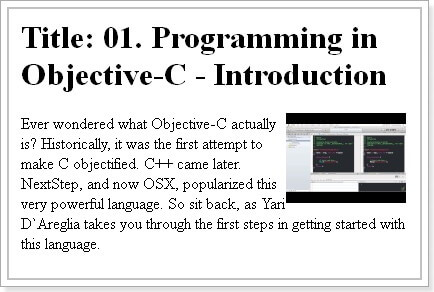
I hope now you have clear understanding of using youtube data api to fetch youtube video details using php. This is just a taste of what youtube api can do. Obviously there's more to it. I'll cover the api more in detail in future posts. If you like this tutorial, don't forget to share it in social media.
No comments:
Post a Comment