Hi, in this post we will see how to read rss feed of a blog, website and display them using php. In case you don't know, RSS stands for 'Really Simple Syndication'. It is a type of web feed used by websites, blogs and other means of online publishing to syndicate the content to its readers. The contents of the rss feed is in xml format. So, to read the feed, you must parse the xml file from the feed url and display the parts of the feed contents one by one in human readable format.
PHP comes with native XML support, so you don't need any third-party tools for doing this. There is also more than one way to read rss feed, one is to use Simple XML Parser and the other is the DOMDocument library which I will show you later in this tutorial.

How to Read RSS Feed in PHP using Simple XML Parser?
Simple XML Parser in PHP supports a native function called simplexml_load_file()
to read xml file. This function interprets the xml file into an object array. Below is the small php function to read the rss feed from the given feed url.
It gets the feed contents from an rss url, loops through it and returns the property of each feed item into an array.
<?php function read_rss_feed($rss_url) { $rss = simplexml_load_file($rss_url); $rss_feed = array(); foreach ($rss->channel->item as $item) { $node = array ( 'title' => $item->title, 'link' => $item->link, 'pubDate' => $item->pubDate, 'description' => implode(' ', array_slice(explode(' ', $item->description), 0, 40)) ); array_push($rss_feed, $node); } return $rss_feed; } ?>
Displaying RSS Feed:
Now you have to call the above read_rss_feed()
function and display the feed lists wherever you want.
<?php // read feed $feed = read_rss_feed('http://wordpress.org/news/feed/'); // set header header('Content-Type: text/html; charset=utf-8'); echo '<div style="width:60%; margin:0 auto;">'; echo '<h1>RSS Feed List</h1>'; // display rss feed foreach($feed as $item) { echo '<h4><a href="'. $item['link'] .'">' . $item['title'] . '</a></h4>'; echo '<p>' . $item['pubDate'] . '</p>'; echo '<p>' . $item['description'] . '</p>'; } echo '</div>'; ?>
Running this script will produce an output like this,
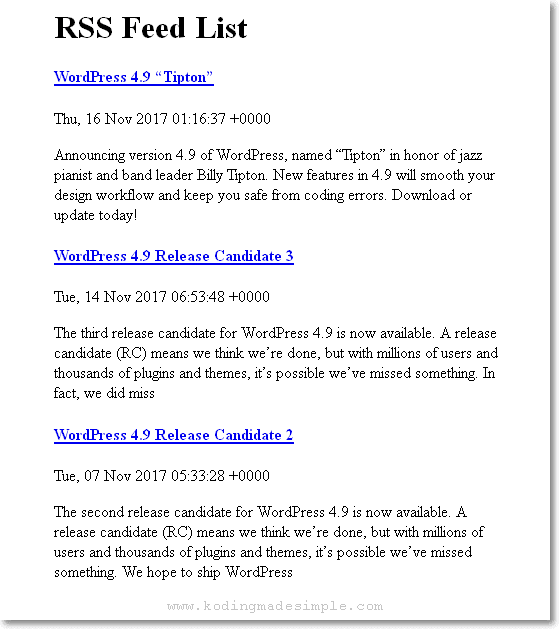
Using DOMDocument Method:
As I said earlier, there is another way to read the rss feeds and that is to use the DOM extension php library. It is a DOM parser which makes it easier for you to read the xml data. The following code shows how to use DOMDocument to work with the rss feed from a url.
<?php $rss = new DOMDocument(); $rss->load('http://wordpress.org/news/feed/'); // set header header('Content-Type: text/html; charset=utf-8'); echo '<div style="width:60%; margin:0 auto;">'; echo '<h1>RSS Feed List</h1>'; foreach ($rss->getElementsByTagName('item') as $item) { echo '<h4><a href="'. $item->getElementsByTagName('link')->item(0)->nodeValue .'">' . $item->getElementsByTagName('title')->item(0)->nodeValue . '</a></h4>'; echo '<p>' . $item->getElementsByTagName('pubDate')->item(0)->nodeValue . '</p>'; echo '<p>' . $item->getElementsByTagName('description')->item(0)->nodeValue . '</p>'; } echo '</div>'; ?>
The above code creates a DOM object and loads the rss feed from a url, then loops through the DOM node one by one to extract the pieces of the feed contents and display it in a readable format.
Also Read:That explains about reading rss feeds from websites/blogs using php. You can use the native DOM extension or the Simple XML parser to parse xml data and list it. I hope you like this tutorial. If you find it useful, please don't forget to share it in your circle.
No comments:
Post a Comment