Hi! Today let's see how to print contents of a div using javascript. Have you ever tried printing a web page or part of a page programmatically? There is an interesting utility in JavaScript called print(). It is one of the methods of the 'Window' object and is used to trigger your system's printing feature.
In case you want to print part of a page area, the simple solution is to place all the printable content inside a 'div' block and invoke the print action with some user event. Let's see how to do it.
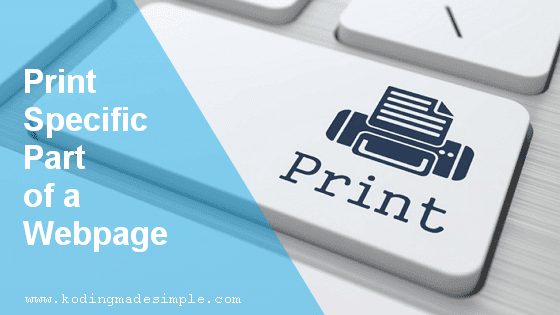
Print Contents of a Div in JavaScript:
Let's first create a div container to hold the printable part.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Print Part of a Webpage with JavaScript</title> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet" type="text/css" /> </head> <body> <div class="container"> <div id="printableDiv" class="jumbotron"> <h2>Test Printing</h2> <p>Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Aenean commodo ligula eget dolor. Aenean massa. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Donec quam felis, ultricies nec, pellentesque eu, pretium quis, sem. Nulla consequat massa quis enim.</p> </div> </div> </body> </html>
In the above markup, we have created a <div>
container with an id #printableDiv
and placed some text inside. This is the block we need to print.
Next add a button element to trigger the print action when the user clicks on it.
<button type="button" class="btn btn-warning btn-lg" onclick="printIt('printableDiv')">Print this block</button>
Include onclick
event to the button and pass the id of the div
we have created earlier.
Finally the printing part! Write a simple java script function to print the div contents.
JavaScript:
<script type="text/javascript"> function printIt(divID) { var divContent = document.getElementById(divID); var WinPrint = window.open('', '', 'width=800,height=600'); WinPrint.document.write('<link rel="stylesheet" type="text/css" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">'); WinPrint.document.write(divContent.innerHTML); WinPrint.document.close(); WinPrint.focus(); WinPrint.print(); WinPrint.close(); } </script>
The js function will open a window of defined width and height, write the contents of the div to it and invokes printing window using the print()
function.
Keep in mind that the contents will be printed without any css styles you have in the webpage. To preserve styles, you must link the css file using windowsobject. document.write()
method like I did in the JS code.
One good thing about using this method is it will not fire printing immediately, but the print window as a means of confirmation - just leaving the choice to users in case they clicked on it accidentally.
Read Also:- How to Lazy Load Images using PHP and JavaScript
- jQuery Datatables Server-side Processing in PHP & MySQL
That explains printing div contents using javascript. The method described in this post is very useful to print a portion of a webpage. You just have to keep all the printable contents in a div block and trigger the java script function. I hope you like this tutorial. Please share it in your social circle if you find it useful.
No comments:
Post a Comment