Hi! In this post let us see How to Convert XML File/String to Array in PHP. Its reverse process of converting array to xml has been already discussed and you can find that tutorial here. It’s a known fact that XML is used to store and exchange information among web services and still some of them provide their API response in xml format. In such case you have to parse those xml files and extract data to process it. PHP provides a handy library called SIMPLEXML to handle xml data. Here I’ll show you how easy it is to use simplexml library to parse and covert the xml file to php array.
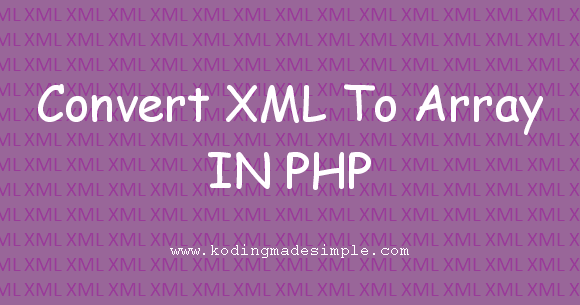
Converting XML File to PHP Array
Converting xml data into array is simpler than you think and we are going to take advantage of simplexml lib and json functions to complete the task with minimal code. Just take this below xml file as an example for our purpose.
Data.xml
<?xml version="1.0" ?> - <root> - <item0> <id>XYZ100</id> - <personal> <name>Ashton Cox</name> <gender>Male</gender> <age>32</age> - <address> <street>7 24th Street</street> <city>New York</city> <state>NY</state> <zipcode>10038</zipcode> </address> </personal> - <profile> <position>Team Lead</position> <department>Software</department> </profile> </item0> - <item1> <id>XYZ121</id> - <personal> <name>Rhona Davidson</name> <gender>Female</gender> <age>40</age> - <address> <street>S2 115th Street</street> <city>New York</city> <state>NY</state> <zipcode>10100</zipcode> </address> </personal> - <profile> <position>Integration Specialist</position> <department>Operations</department> </profile> </item1> </root>
Here is the php script to convert the above xml file to associative array.
<?php // function to convert xml to php array function xml2Array($filename) { $xml = simplexml_load_file($filename, "SimpleXMLElement", LIBXML_NOCDATA); $json = json_encode($xml); return json_decode($json,TRUE); } // function callback $arr = xml2Array("Data.xml"); print_r($arr); // produces output: // Array ( [item0] => Array ( [id] => XYZ100 [personal] => Array ( [name] => Ashton Cox [gender] => Male [age] => 32 [address] => Array ( [street] => 7 24th Street [city] => New York [state] => NY [zipcode] => 10038 ) ) [profile] => Array ( [position] => Team Lead [department] => Software ) ) [item1] => Array ( [id] => XYZ121 [personal] => Array ( [name] => Rhona Davidson [gender] => Female [age] => 40 [address] => Array ( [street] => S2 115th Street [city] => New York [state] => NY [zipcode] => 10100 ) ) [profile] => Array ( [position] => Integration Specialist [department] => Operations ) ) ) ?>
We have written a php function xml2Array()
that takes up the xml filename as argument and convert it into array.
The method simplexml_load_file($filename, "SimpleXMLElement", LIBXML_NOCDATA)
converts the given XML file into a SimpleXMLElement object. Its second param SimpleXMLElement specifies the class name of the new object created. And the third param LIBXML_NOCDATA is a constant that tells to merge CDATA as text nodes. Learn more about the method here.
And the method json_encode($xmlstring)
returns the JSON representation of the given object.
Finally json_decode($json, TRUE)
decodes the given JSON string into an array or object. Providing the second param TRUE tells it to return an associative array.
Converting XML String to PHP Array
Alternatively if you have xml data as string and wish to convert to array, then you have to use this statement instead of loading from the file.
$xml = simplexml_load_string($xmlstring, "SimpleXMLElement", LIBXML_NOCDATA);
This converts a well-formed xml string to a simplexmlelement object. You have to change only this line and the rest of the code remains the same.
Read Also:- How to Convert Multi-Dimensional Array to XML in PHP
- How to Insert Multiple JSON Data into MySQL DB in PHP
Thus with the help of this php script you can easily convert xml file or string to an array.
No comments:
Post a Comment