Hi! Welcome to Koding Made Simple. Today we'll see how to send html email in php. PHP's mail() function is simple and effective and let you send emails with text/html contents and with attachments. Though it has some shortcomings compared to other mailer libraries like PHPMailer, it does the job and comes in-built with PHP package. Plain text emails are good enough, but the ability to send html emails is more powerful in email marketing and other promotions.
HTML emails include html tags and let you add images, format texts and other eye-catching call-to-action buttons etc. But you have to add additional headers for mailing them.
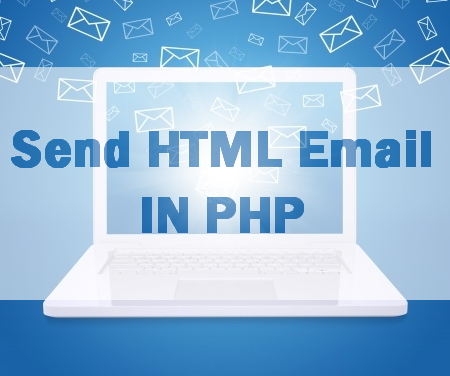
Using SMTP for Sending Email
You can send mails via third party Mail Servers but you need authentication first. That is if you want to send email via Gmail in php, then you must have a working gmail account and provide the accounts email-id and password for authentication. We'll see how to set up the php configuration for sending email via gmail smtp server.
How to Send HTML Email in PHP Using Gmail SMTP
You need to change the settings in two places i.e., php.ini
and sendmail.ini
files. You must remove the ;
at the starting of the line to enable the settings in these files. Please note the configuration is given for the xampp package. Open 'php.ini' file located at C:\xampp\php\php.ini
and edit the settings like below.
php.ini
[mail function] SMTP = smtp.gmail.com smtp_port = 587 sendmail_from = christmascontest@gmail.com sendmail_path = "\"C:\xampp\sendmail\sendmail.exe\" -t"Next open the 'sendmail.ini' file located at
C:\xampp\sendmail\sendmail.ini
and make the below changes.
sendmail.ini
smtp_server = smtp.gmail.com smtp_port = 587 auth_username = christmascontest@gmail.com auth_password = christmascontest force_sender = christmascontest@gmail.com
Save the files and restart apache server for the changes to reflect. Now you are ready to send send html email using php mail()
function.
Create a php file and write down the below php mailer code to it and save.
PHP Code for Sending HTML Email
<?php // from email $from = 'christmascontest@gmail.com'; // change this // to email $to = 'sally@somedomain.com, justin@somedomain.com, parker@somedomain.com'; // change this // subject $subject = 'Christmas Contest Announcement'; // html message $htmlmsg = '<html> <head> <title>Christmas Contest Winners</title> </head> <body> <h1>Hi! We are glad to announce the Christmas contest winners...</h1> <table> <tr style="background-color: #EEE;"> <th width="25%">#</th> <th width="35%">Ticket No.</th> <th>Name</th> </tr> <tr> <td>#1</td> <td>P646MLDO808K</td> <td>Sally</td> </tr> <tr style="background-color: #EEE;"> <td>#2</td> <td>DFJ859LV9D5U</td> <td>Parker</td> </tr> <tr> <td>#3</td> <td>AU30HI8IHL96</td> <td>Justin</td> </tr> </table> </body> </html>'; // set content type header for html email $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=UTF-8' . "\r\n"; // set additional headers $headers .= 'From: Christmas Contest <christmascontest@gmail.com>' . "\r\n"; $headers .= 'Cc: contestadmin@gmail.com' . "\r\n"; // send email if (mail($to, $subject, $htmlmsg, $headers)) echo "Email sent successfully!"; else echo "Error sending email! Please try again later..."; ?>
Change $from
to your gmail-id and $to
variable to the recipients email-id.
Plus the code includes content type header which is a must for sending html emails and additional headers should be appended with CRLF
.
Note: If you have enabled two-way authentication for Google account then please disable it for this code to work.
That's all about sending html email in php via smtp server.
Read Also:
Nice article.. thank you for sharing it.
ReplyDeleteHello and thanks for this tutorial, i will ask, what if I don't want to use localhost, but a hosting site to send html email with phpmailer, how do I go about it? Thanks
ReplyDeleteThen you get the smtp settings from your hosting provider and use it. Also make sure sending email is possible with your account.
DeleteCheers.
Thanks for your reply. Actually, i have done that, thing is, anytime i use the code below with Html as its body, messages are dropped into spam, but with plain text only, my messages are dropped into inbox.
DeleteSo, i'm hoping you can help me out. Thanks
Host='smtp.gmail.com';
$mail->SMTPAuth='true';
$mail->Username='username';
$mail->Password='temp()paswword';
$mail->SMTPSecure='tls';
$mail->Port=587;
$mail->SetFrom('from@sample.com','from');
$mail->addAddress('receiver@sample.com');
$mail->addReplyTo('replyto@sample.com','replyto');
$mail->IsHTML(true);
$mail->Subject='PHPMailer Test';
$mail->Body='PHPMailer Test Subject via Sendmail, basic';
if($mail->Send())
{
echo'Mail sent';
}
else
{
echo "Mail sending failed";
}
?>