Hi! In today's post we will see about creating URL Shortener service using PHP and Google API. Google provides tons of APIs for users to easily complete several complicated tasks. And Google url shortener service (goo.gl) is one among them. In the past I have discussed about some other APIs like youtube api and google finance api. Now I'm going to show you about using google url shortener api service and generate shorten url using php.
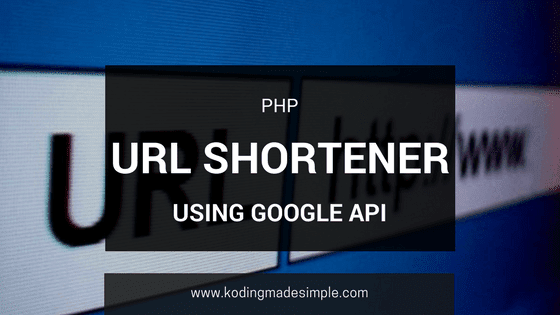
Google URL Shortener API:
The Google URL Shortener is a service that takes long URLs and makes them short with fewer characters to make the link easier to share on social networks or by email. With Google's URL Shortening API, you can create these short urls programmatically by sending the long url through the http request. Please note that the API has a daily limit of 1,000,000 requests for free - more than that goes to your billing.
In order to use google url shortener api, you must first get the api key and have to tag it with each of your REST calls.
- To obtain the key, login to Google developer console
- Create new or select an existing project
- Click 'ENABLE API' and select APIs in the APIS & auth section
- Choose 'URL Shortener API' and click 'ENABLE'
- Under Credentials, generate a new key by selecting 'API key' option.
Creating URL Shortener Service with PHP and Google API:
The following PHP script quickly generates a short URL from a long URL by using the Google's URL Shortener API. So many examples around the Net uses only cURL method. So I have tried a different way in my tutorial. Here I use the file_get_contents()
function to send the HTTP POST request to the api and retrieve the json response containing the short url.
<?php $apikey = 'YOUR_API_KEY'; // change this to yours $longurl = 'https://www.google.com/'; $data = array('longUrl' => $longurl); $context = array( 'http' => array( 'method' => 'post', 'header'=>'Content-Type:application/json', 'content' => json_encode($data) ) ); $context = stream_context_create($context); $response = file_get_contents('https://www.googleapis.com/urlshortener/v1/url?key=' . $apikey, false, $context); $json = json_decode($response, true); echo 'The Long URL: ' . $json['longUrl'] . '<br/>'; echo 'The Shortened URL: ' . $json['id']; // Output // The Long URL: https://www.google.com/ // The Shortened URL: https://goo.gl/Njku ?>
In order to make http request via file_get_contents() function, we have to pass the headers and other options through a context. For that we have used the function stream_context_create()
in the above code. It creates and returns a stream context that we need to make the http request to the web service. It takes up options as an associative array of associative arrays.
The API responds in json format containing shortened url stored in 'id'.
You can display the whole json response from the api in this way,
<?php echo "<pre>"; print_r($response); // Output // { // "kind": "urlshortener#url", // "id": "https://goo.gl/Njku", // "longUrl": "https://www.google.com/" // } ?>Read:
This is how you can generate short urls with php and google api. Feel free to modify the code and add more features to the url service. Google really simplifies the task of shortening url and the good thing is that you don't have to maintain database on your own. I hope you like this tutorial. Please do not forget to share it in social networks.
set up an account and it works online but I can not get the php sample program to work. I supplied my key and the return echoed out for both long URL and Shortened URL was blank. I echoed the long URL and APIKEY before the file_get_contents function was called and both had the correct value. No Error codes were returned.
ReplyDelete