Hi, in this PHP TUTORIAL, we'll see How to insert JSON Data into MySQL using PHP. Check out its reverse process of Converting Data from MySQL to JSON Format in PHP here. Converting json to mysql using php includes several steps and you will learn things like how to read json file, convert json to array and insert that json array into mysql database in this tutorial. For those who wonder what is JSON, let me give a brief introduction.
What is JSON File Format?
JSON file contains information stored in JSON format and has the extension of "*.json". JSON stands for JavaScript Object Notation and is a light weight data exchange format. Being less cluttered and more readable than XML, it has become an easy alternative format to store and exchange data. All modern browsers supports JSON format.
Example of a JSON File
Read Also:- How to Get JSON from Remote URL in PHP
- How to Write JSON String to File in PHP
- How to Convert JSON to CSV File in PHP
Do you want to know how a JSON file looks like? Well here is the sample.
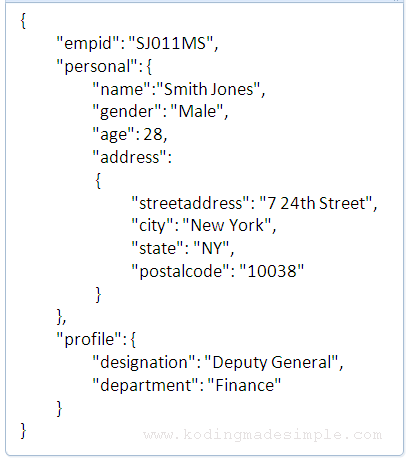
As you can see by yourself, the JSON format is very human readable and the above file contains some employee details. I'm going to use this file as an example for this tutorial and show you how to insert this JSON object into MySQL database in PHP step by step.
Step 1: Connect PHP to MySQL Database
As the first and foremost step we have to connect PHP to the MySQL database in order to insert JSON data into MySQL DB. For that we use mysql_connect()
function to connect PHP with MySQL.
<?php $con = mysql_connect("username","password","") or die('Could not connect: ' . mysql_error()); mysql_select_db("employee", $con); ?>
Here "employee" is the MySQL Database name we want to store the JSON object. Learn more about using mysqli library for php and mysql database connection here.
Step 2: Read the JSON file in PHP
Next we have to read the JSON file and store its contents to a PHP variable. But how to read json file in php? Well! PHP supports the function file_get_contents()
which will read an entire file and returns it as a string. Let’s use it to read our JSON file.
<?php //read the json file contents $jsondata = file_get_contents('empdetails.json'); ?>
Here "empdetails.json" is the JSON file name we want to read.
Step 3: Convert JSON String into PHP Array
The next step for us is to convert json to array. Which is likely we have to convert the JSON string we got from the above step to PHP associative array. Again we use the PHP json decode function which decodes JSON string into PHP array.
<?php //convert json object to php associative array $data = json_decode($jsondata, true); ?>
The first parameter $jsondata
contains the JSON file contents.
The second parameter true
will convert the string into php associative array.
Step 4: Extract the Array Values
Next we have to parse the above JSON array element one by one and store them into PHP variables.
<?php //get the employee details $id = $data['empid']; $name = $data['personal']['name']; $gender = $data['personal']['gender']; $age = $data['personal']['age']; $streetaddress = $data['personal']['address']['streetaddress']; $city = $data['personal']['address']['city']; $state = $data['personal']['address']['state']; $postalcode = $data['personal']['address']['postalcode']; $designation = $data['profile']['designation']; $department = $data['profile']['department']; ?>
Step 5: Insert JSON to MySQL Database with PHP Code
Using the above steps, we have extracted all the values from the JSON file. Finally let's insert the extracted JSON object values into the MySQL table.
<?php //insert into mysql table $sql = "INSERT INTO tbl_emp(empid, empname, gender, age, streetaddress, city, state, postalcode, designation, department) VALUES('$id', '$name', '$gender', '$age', '$streetaddress', '$city', '$state', '$postalcode', '$designation', '$department')"; if(!mysql_query($sql,$con)) { die('Error : ' . mysql_error()); } ?>
We are done!!! Now we have successfully imported JSON data into MySQL database.
Here is the complete php code snippet I have used to insert JSON to MySQL using PHP.
Complete PHP Script
<?php //connect to mysql db $con = mysql_connect("username","password","") or die('Could not connect: ' . mysql_error()); //connect to the employee database mysql_select_db("employee", $con); //read the json file contents $jsondata = file_get_contents('empdetails.json'); //convert json object to php associative array $data = json_decode($jsondata, true); //get the employee details $id = $data['empid']; $name = $data['personal']['name']; $gender = $data['personal']['gender']; $age = $data['personal']['age']; $streetaddress = $data['personal']['address']['streetaddress']; $city = $data['personal']['address']['city']; $state = $data['personal']['address']['state']; $postalcode = $data['personal']['address']['postalcode']; $designation = $data['profile']['designation']; $department = $data['profile']['department']; //insert into mysql table $sql = "INSERT INTO tbl_emp(empid, empname, gender, age, streetaddress, city, state, postalcode, designation, department) VALUES('$id', '$name', '$gender', '$age', '$streetaddress', '$city', '$state', '$postalcode', '$designation', '$department')"; if(!mysql_query($sql,$con)) { die('Error : ' . mysql_error()); } ?>
empdetails.json
{ "empid": "SJ011MS", "personal": { "name": "Smith Jones", "gender": "Male", "age": "28", "address": { "streetaddress": "7 24th Street", "city": "New York", "state": "NY", "postalcode": "10038" } }, "profile": { "designation": "Deputy General", "department": "Finance" } }
Read:
- How to Insert Multiple JSON Array into MySQL in PHP
- How to Convert MySQL to JSON Format using PHP
- How to Pretty Print JSON with PHP
Hope this tutorial helps you to understand how to insert JSON data into MySQL using PHP.
Last Modified: Feb-21-2017
How do you use this for more than one row of data? Say in the json there are two empid's, and you want to insert each of them?
ReplyDeleteHi, just loop through the json array if you have multiple rows like this.
Delete//read the json file contents
$jsondata = file_get_contents('empdetails.json');
//convert json object to php associative array
$data = json_decode($jsondata, true);
foreach($data as $row)
{
//get the employee details
$id = $row['empid'];
...
//insert into db
mysql_query($sql,$con);
}
<?php
ReplyDeleteinclude 'include/connect.php';
error_reporting(E_ALL);
$jsondata = file_get_contents('D:\xamp\htdocs\lobstersapi\monitoring_log.php');
$data = json_decode($jsondata, true);
if (is_array($data)) {
foreach ($data as $row) {
$am = $row['item']['am'];
$pm = $row['item']['pm'];
$unit = $row['unit'];
$day = $row['day'];
$userid = $row['userid'];
$sql = "INSERT INTO monitoring_log (unit, am, pm, day, userid)
VALUES ('".$am."', '".$pm."', '".$unit."', '".$day."', '".$userid."')";
mysql_query($sql);
}
}
but sir still my code is not working. Can you solve my issue
ReplyDeleteHi Umair, you haven't mentioned what error you are getting with the above code. But I could see you are trying to run a "*.php" file and get the output. Passing the exact file path to file_get_contents() won't execute the file.
DeleteInstead try using this,
file_get_contents('http://localhost/lobstersapi/monitoring_log.php')
Also make sure your web server is running for this to work.
Hope this helps you :)
still not working.
ReplyDeletemy json data that i am getting from iphone developer is {
"New item" : {
"PM" : false,
"AM" : false
},
"title" : " Unit 13"
}
and my code is :
<?php
include 'include/connect.php';
error_reporting(E_ALL);
$jsondata = file_get_contents('http://localhost/lobstersapi/monitoring_log.php');
$data = json_decode($jsondata, true);
if (is_array($data)) {
foreach ($data as $row) {
$am = $row['New item']['am'];
$pm = $row['New item']['pm'];
$unit = $row['unit'];
$sql = "INSERT INTO monitoring_log (am, pm, unit)
VALUES ('".$am."', '".$pm."', '".$unit."')";
mysql_query($sql);
}
}
not showing any error.
Hi, I could see some inconsistencies in your code. Do you get the json output properly? Even then, if the output contains single row like this,
Delete{
"New item" : {
"PM" : "false",
"AM" : "false"
},
"title" : " Unit 13"
}
then you should not use foreach loop to parse it. Use the loop only if json output contains multiple rows that looks something like this,
[{
"New item" : {
"PM" : "false",
"AM" : "false"
},
"title" : " Unit 13"
},
{
"New item" : {
"PM" : "false",
"AM" : "false"
},
"title" : " Unit 14"
}]
Also while retrieving data from json, you should use the exact key attribute like this,
$am = $row['New item']['AM'];
$pm = $row['New item']['PM'];
$unit = $row['title'];
(I could see you are using lower case and wrong key attributes here).
Note: If you don't get error print the values in browser and make sure you get the desired result.
Thank you so much sir
ReplyDeleteNice tutorial,
ReplyDeleteBut I want to store JSON data as a JSON into mysql, not the way you have done, then what are the steps to store and retrieve JSON from mysql using php?
Hey! you can store json data in mysql without breaking up into columns. Only proper escaping of json will protect the db from security risk.
DeleteBut it's not a proper way to do as it just stores a long length of string and will restrict mysql's ability to search, sort or index data and processing concurrent queries.
This thread discusses the topic in more detail http://stackoverflow.com/questions/20041835/putting-json-string-as-field-data-on-mysql
Hope this helps :)
How can a json from an api be saved into mySQL? For example I have this json coming from an api http://api.androidhive.info/json/movies.json. How can I save this data into the database?
ReplyDeleteHi,
DeleteIf your json o/p is from remote server like the one you have mentioned (http://api.androidhive.info/json/movies.json) then pass the complete url like this,
//read json file
$jsondata = file_get_contents('http://api.androidhive.info/json/movies.json');
This should work :)
Hi,
ReplyDeleteI can't send my json data to mysql!! I need help...
$json_data = '[{
"external_urls" : "https://open.spotify.com/artist/2QWIScpFDNxmS6ZEMIUvgm",
"followers" : {
"total" : 116986
},
"genres" : "latin alternative",
"id" : "2QWIScpFDNxmS6ZEMIUvgm",
"name" : "Julieta Venegas",
"popularity" : 72,
"type" : "artist",
}]';
//convert to stdclass object
$data = json_decode($json_data, true);
$href = $data['external_urls'];
$followers = $data['followers']['total'];
$genres = $data['genres'];
$id = $data['id'];
$name = $data['name'];
$popularity = $data['popularity'];
$type = $data['type'];
//insert values into mysql database
$sql="INSERT INTO `spotify`(`external_urls`, `followers`, `genres`, `empid`, `empname`, `popularity`, `type`)
VALUES ('$href', '$followers', '$genres', '$id', '$name', '$popularity', '$type')";
Hi, the json you have given is invalid with the comma (,) at the end of the last item ("type" : "artist",). It should be like,
Delete$json_data = '[{
...
"popularity" : 72,
"type" : "artist"
}]';
Also the square brackets [] around json data makes it an array. So you should iterate through it like this,
foreach ($data as $row) {
$href = $row['external_urls'];
$followers = $row['followers']['total'];
$genres = $row['genres'];
$id = $row['id'];
$name = $row['name'];
$popularity = $row['popularity'];
$type = $row['type'];
//insert values into mysql database
$sql="INSERT INTO `spotify`(`external_urls`, `followers`, `genres`, `empid`, `empname`, `popularity`, `type`)
VALUES ('$href', '$followers', '$genres', '$id', '$name', '$popularity', '$type')";
}
This should work :)
This work..!! :) Thank you so much Valli!!
DeleteGlad it works :)
DeleteThank you so much!
ReplyDeleteI'm new in web-programming and was feeling nervous with one of my first tasks. However with your help i did a daytask within an hour.
Great!
Glad! I could help you...Cheers!!!
DeleteHey valli, great work here, could you help me please?
DeleteI try to load a JSON named Business from this website http://www.yelp.com/dataset_challenge. But give me an error on business_id, can t load that.
my code is the following:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
}
catch(PDOException $e)
{
echo "Connection failed: " . $e->getMessage();
}
//read the json file contents
$jsondata = file_get_contents('c:\yelp_academic_dataset_business.json');
ini_set('memory_limit', '512M');
//convert json object to php associative array
$data = json_decode($jsondata, true);
//get the employee details
$idBusiness = $data['business_id'];
$name = $data['name'];
$neighborhoods = $data['neighborhoods'];
$full_address = $data['full_address'];
$city = $data['city'];
$state = $data['state'];
$latitude = $data['latitude'];
$longitude = $data['longitude'];
$stars = $data['stars'];
$review_count = $data['review_count'];
$open = $data['open'];
$procedure = $conn -> prepare("INSERT INTO business(business_id, name, neighborhoods, full_address, city, state, latitude, longitude, stars, review_count, open)
VALUES('$idBusiness', '$name', '$neighborhoods', '$full_address', '$city', '$state', '$latitude', '$longitude', '$stars', '$review_count', '$open')");
$procedure -> execute(); ?>
Hey valli, great work here, could you help me please?
DeleteI try to load a JSON named Business from this website http://www.yelp.com/dataset_challenge. But give me an error on business_id, can t load that.
my code is the following:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
}
catch(PDOException $e)
{
echo "Connection failed: " . $e->getMessage();
}
//read the json file contents
$jsondata = file_get_contents('c:\yelp_academic_dataset_business.json');
ini_set('memory_limit', '512M');
//convert json object to php associative array
$data = json_decode($jsondata, true);
//get the employee details
$idBusiness = $data['business_id'];
$name = $data['name'];
$neighborhoods = $data['neighborhoods'];
$full_address = $data['full_address'];
$city = $data['city'];
$state = $data['state'];
$latitude = $data['latitude'];
$longitude = $data['longitude'];
$stars = $data['stars'];
$review_count = $data['review_count'];
$open = $data['open'];
$procedure = $conn -> prepare("INSERT INTO business(business_id, name, neighborhoods, full_address, city, state, latitude, longitude, stars, review_count, open)
VALUES('$idBusiness', '$name', '$neighborhoods', '$full_address', '$city', '$state', '$latitude', '$longitude', '$stars', '$review_count', '$open')");
$procedure -> execute(); ?>
Could you help me? Then after make this work i need to load the field attributes to a table in my sql named attributes to the fields Designation and value, How could i do that, if there is so many attributes and i can t call them by your code, like garage, parking, etc. take a look in the Json named business please.
DeleteHere's a example of a line of the JSON.
{"business_id": "fNGIbpazjTRdXgwRY_NIXA", "full_address": "1201 Washington Ave\nCarnegie, PA 15106", "hours": {}, "open": true, "categories": ["Bars", "American (Traditional)", "Nightlife", "Lounges", "Restaurants"], "city": "Carnegie", "review_count": 5, "name": "Rocky's Lounge", "neighborhoods": [], "longitude": -80.084941599999993, "state": "PA", "stars": 4.0, "latitude": 40.396468800000001, "attributes": {"Alcohol": "full_bar", "Noise Level": "average", "Music": {"dj": false, "background_music": true, "karaoke": false, "live": false, "video": false, "jukebox": false}, "Attire": "casual", "Ambience": {"romantic": false, "intimate": false, "touristy": false, "hipster": false, "divey": false, "classy": false, "trendy": false, "upscale": false, "casual": false}, "Good for Kids": true, "Wheelchair Accessible": false, "Good For Dancing": false, "Delivery": false, "Coat Check": false, "Smoking": "no", "Accepts Credit Cards": true, "Take-out": false, "Price Range": 2, "Outdoor Seating": false, "Takes Reservations": false, "Waiter Service": true, "Caters": false, "Good For": {"dessert": false, "latenight": false, "lunch": false, "dinner": false, "brunch": false, "breakfast": false}, "Parking": {"garage": false, "street": false, "validated": false, "lot": false, "valet": false}, "Has TV": true, "Good For Groups": true}, "type": "business"}
Thank you :)
Hi, What error you get? From the json you have given below, the business id seems to be a string value. Did you set the mysql 'datatype' for the said id field as varchar or not? Please make sure you use the matching datatypes for the mysql field attributes.
DeleteFor the last query,
Your query is not clear and I hope this is what you want to ask.
The given json is a complex nested object and to get the value for the key 'garage' you should use like this,
$garage = $data['attributes']['parking']['garage'];
If you have queries apart from this, please make it clear.
Cheers.
Is it possible to exclude certain lines from going into the database? My json file has 1 array with about 20 items in it. Do I just not write in those specific lines in the php so it'll ignore them? I know the file is small, just doing this as a learning tool.
DeleteHi, you can do it by checking on the key value on json records like this,
Delete//convert json object to associative array
$data = json_decode($jsondata, true);
//loop through the array
foreach ($data as $row) {
if ($row['empid'] != '5121') { //replace this with your own filter
//get the employee details
$id = $row['empid'];
....
//insert into mysql table
$sql = "INSERT INTO tbl_emp(empid, empname, gender, age, streetaddress, city, state, postalcode, designation, department)
VALUES('$id', '$name', '$gender', '$age', '$streetaddress', '$city', '$state', '$postalcode', '$designation', '$department')";
...
}
}
Hope this helps.
Cheers.
Great post, thanks ;-)
ReplyDeleteGlad you liked it :-)
Deletei will try thanks
ReplyDeleteHi Vallie,
ReplyDeleteHmm what if that was a dynamic JSon result, instead of INSERT syntax to MySQL, possible to UPDATE syntax, or using PHP with if statement before INSERT-ing ?
Hi,
DeleteThe process is same for dynamic json too, for e.g., you can make an api call and store the result in a variable and proceed in the same way discussed above.
For your second question, yes you can use the json values with UPDATE query also. As for using if statement, say you want to insert the values only for 'SALES' department, then use it like
If ($department == "SALES") {
// Insert into DB
// or process the data as you wish
}
Hope that helps!
Cheers.
Hi,
ReplyDeleteI am trying to save the same json as mentioned above but not able to save data in mysql, the code is working but it is showing empty fields in db.
and I have tried to echo the file contents and it is successful ,but the problem is that if I try to echo the decoded data then it shows a blank page.
Hi! It seems like the json format you are trying is not valid. Please make sure you haven't missed out quotes or any thing else.
DeleteYou can check if you are using the right JSON format like this,
http://www.kodingmadesimple.com/2015/04/validate-json-string-php.html
Hope that helps.
Cheers.
Hello , how could I do with my code ?
ReplyDeleteI need to get the data :
Go dia_0 , dia_1 , dia_2 , dia_3 , dia_4 , dia_5 , dia_6 , dia_7 , DC , PMP and CR .
This is my code .
{ "type" : " FeatureCollection "
"features" : [
{ "Type" : " Feature "
"You properties" :
{
" Id" : "1 "
" dia_0 ": " 0 "
" dia_1 ": " 0 "
" dia_2 ": " 0 "
" dia_3 ": " 0 "
" dia_4 ": " 0 "
" dia_5 ": " 0 "
" dia_6 ": " 0 "
" dia_7 ": " 0 "
"CC" : "0 "
" PMP " , " 0 "
"CR ": " 0 "},
... please help
Assalam-o-Alikum !!
ReplyDeletei m trying to save data form json to sql by using following steps but i m getting error any help ??
//json file//
{
"error_code": "0",
"message": "success",
"food_items": [
{
"id": "1",
"food_name": "apple",
"food_fat": "10",
"food_bar_code": "25",
"food_carbs": "26",
"food_protein": "20",
"food_servings": "125",
"food_points": "2",
"food_fiber": "2"
}
]
}
// php code //
include 'config.php';
echo $jsondata = file_get_contents('document.json');
$data = json_decode($jsondata, true);
//get the employee details
$food_name = $data['food_name'];
$food_fat = $data['food_fat'];
$food_bar_code = $data['food_bar_code'];
$food_carbs = $data['food_carbs'];
$food_protein = $data['food_protein'];
$food_servings = $data['food_servings'];
$food_points = $data['points'];
$food_fiber = $data['food_fiber'];
$addFood = "INSERT INTO food_points (food_name, food_fat, food_bar_code, food_carbs, food_protein, food_servings, points, food_fiber)
VALUES
('$food_name', '$food_fat', '$food_bar_code', '$food_carbs', '$food_protein', '$food_servings', '$food_points', '$food_fiber')";
if ($conn->query($addFood)===TRUE)
{
echo "data is updated . . . . !!!";
}
else
{
echo "Error: " . $addFood . "
" . $conn->error;;
}
// getting this error //
Notice: Undefined index: food_name in E:\z\htdocs\ramis\test\index.php on line 24
Notice: Undefined index: food_fat in E:\z\htdocs\ramis\test\index.php on line 25
Notice: Undefined index: food_bar_code in E:\z\htdocs\ramis\test\index.php on line 26
Notice: Undefined index: food_carbs in E:\z\htdocs\ramis\test\index.php on line 27
Notice: Undefined index: food_protein in E:\z\htdocs\ramis\test\index.php on line 28
Notice: Undefined index: food_servings in E:\z\htdocs\ramis\test\index.php on line 29
Notice: Undefined index: points in E:\z\htdocs\ramis\test\index.php on line 30
Notice: Undefined index: food_fiber in E:\z\htdocs\ramis\test\index.php on line 31
data is updated . . . . !!!
Welcome! Accessing your json data like this won't work. The key "food_items": [ ] itself is representing an array. Note that the square brackets around [] is considered as array and should be parsed with loop.
DeleteWorking with json array is clearly described in another tutorial. Please check this url: http://www.kodingmadesimple.com/2015/10/insert-multiple-json-data-into-mysql-database-php.html
Let me know if you need any more help.
Cheers.
Hi!
ReplyDeleteThanx everybody for help, it's very useful!
How can I get and decode information from several json files in one php script? The files have the simmilar structure. Could you transform the script for me?
Hi!
ReplyDeleteThanx everybody for help, it's very useful!
How can I get and decode information from several json files in one php script? The files have the simmilar structure. Could you transform the script for me?
Hi! Say you have all the json files stored in a directory, you can read through the files one by one like this.
Delete$jdir = scandir($dirpath);
foreach($jdir as $file)
{
//read the json file contents
$jsondata = file_get_contents($file);
...
}
Cheers:)
My error is-
ReplyDeletePHP Notice: Undefined index: dishes in /Applications/MAMP/htdocs/ionicserver/start.php on line 26
I am not able to view the data in phpmyadmin database
ReplyDeleteits showing data succefully inserted
my code is looks like this
Thanks for share!
ReplyDeleteWelcome Ahmed:)
Deletehello,
ReplyDeleteI need help please: i have a php file which offer a jsonarray, i need to store this result into a json file so i can explore it later !
For example for the command file_get_contents !
Any response please :( ?!
Hi,
ReplyDeleteI need Help please !
I need to store the result provided by my php file (which is a jsonarray) into a json file, so i can explore it later for example to use the command file_get_contents !
Any response please ?
We have an article that discusses the topic in detail. Please check this below link...
Deletehttp://www.kodingmadesimple.com/2016/05/how-to-write-json-to-file-in-php.html
Cheers.
Its really good article for ajax in codeiginiter. Thanks a lot. http://www.phptutorials.club
ReplyDeleteHey I Want To display My Employee details "table" on my webpage only using php and json.... I have created already .json file but i don't know how to display in browser using only PHP.. help me..
ReplyDeletePlease check these links,
Deletehttp://www.kodingmadesimple.com/2016/02/get-json-from-url-in-php.html
http://www.kodingmadesimple.com/2016/05/how-to-pretty-print-json-in-php.html
Cheers.
Hi my error is
ReplyDeleteNotice: Undefined index: id in C:\xampp\htdocs\demo_json\demo.php on line 21
Notice: Undefined index: department in C:\xampp\htdocs\demo_json\demo.php on line 22
Notice: Undefined index: institution in C:\xampp\htdocs\demo_json\demo.php on line 23
Notice: Undefined index: yearGuarantee in C:\xampp\htdocs\demo_json\demo.php on line 24
Notice: Undefined index: yearStart in C:\xampp\htdocs\demo_json\demo.php on line 25
Notice: Undefined index: yearStop in C:\xampp\htdocs\demo_json\demo.php on line 26
------------------------------------------------------------------------------------------------------------
My Code
Great article for reference
ReplyDeleteI have written the same thing Link:http://www.psychocodes.in/Insert-JSON-data-into-MySQL-using-php.html
Can you have an IF statement for this at all? like:
ReplyDeleteJSON
"dataset":[
{"field1":509,"field2":5443,"id":1},
{"field1":32,"field2":4211,"id":2},
{"field1":112,"field2":1221,"id":3},
]
PHP
$entry1 = $data['dataset']['field1'] ONLY IF ID =1;
$entry2 = $data['dataset']['field1'] ONLY IF ID =2;
Any help be good :) thanks
ReplyDeleteHi
Can you help me import this json
{
"lljuree33321.16": {
"lat": 57.532696,
"lng": 9.949331
},
"ae22564266.16": {
"lat": 55.675633,
"lng": 12.505909,
"name": "The Atom",
"test": false
},
"2203467336.16": {
"lat": 55.675571,
"lng": 12.505199,
"name": "Johannes Bjerg 1918",
"test": false
}
}
Hi this article helps me a lot to understand the use of json.By the way, I am also a developing a web application but Im stuck of a particular function. What I want to do is select multiple data/rows from mysql table then change it into json so that I could still add values to it from a php variables before inserting into a new table. Is this possible? I hope you could help me on how to go about that. Thanks in advance.
ReplyDelete"results":
ReplyDelete[{"numberOfAdditionalListings":1,
"primaryContacts":
[
{"type":"PHONE","value":"(02) 6557 8330"},
{"type":"EMAIL","value":"ahpearse@bigpond.com.au"},
{"type":"URL","value":"http://www.allanpearsefunerals.com.au/2-home"}
],
"primaryAddress":{"state":"NSW","type":"PHYSICAL","postcode":"2430","suburb":"Taree","longitude":"152.477357","latitude":"-31.903368","geoCodeGranularity":"PROPERTY","addressLine":"17 Oxley St","mappable":true},
"detailsLink":"http://www.yellowpages.com.au/nsw/taree/allan-pearse-funerals-pty-ltd-15399420-listing.html?referredBy=TAPI-W6i9iPhJQTtRNrM_YMwnllPJ61rH16v7",
"imageGallery":
[
{
"thumbnailUrl":"http://s0.yellowpages.com.au/e2134f7e-1945-4eb5-a402-25ec6f8efee7/allan-pearse-funerals-pty-ltd-taree-2430-thumbnail.jpg",
"largeUrl":"http://s0.yellowpages.com.au/b6cd2d7c-3078-468b-8ec3-e154803ca44d/allan-pearse-funerals-pty-ltd-taree-2430-image.jpg"
},
{
"thumbnailUrl":"http://s0.yellowpages.com.au/0e99853b-95bf-4e8f-80a7-ffd3c0ea460f/allan-pearse-funerals-pty-ltd-taree-2430-thumbnail.jpg",
"largeUrl":"http://s0.yellowpages.com.au/b78a208c-180c-48ca-89ac-3feb8c4776a2/allan-pearse-funerals-pty-ltd-taree-2430-image.jpg"},
{"thumbnailUrl":"http://s0.yellowpages.com.au/d690e122-f178-4801-9872-dcf5f1d158c2/allan-pearse-funerals-pty-ltd-taree-2430-thumbnail.jpg",
"largeUrl":"http://s0.yellowpages.com.au/8282c451-a8a4-4bd7-83a6-96ddd5d1aecd/allan-pearse-funerals-pty-ltd-taree-2430-image.jpg"}],
"externalLinks":[{"url":"http://www.allanpearsefunerals.com.au/3-home","displayValue":"About Us","label":"Other","type":"DEEP_LINK"},
{"url":"http://www.allanpearsefunerals.com.au/3-home","displayValue":"Testimonials","label":"Other","type":"DEEP_LINK"},
{"url":"http://www.allanpearsefunerals.com.au/3-home","displayValue":"FAQ","label":"Other","type":"DEEP_LINK"},
{"url":"http://www.allanpearsefunerals.com.au/3-services","displayValue":"Services","label":"Other","type":"DEEP_LINK"},
{"url":"http://www.allanpearsefunerals.com.au/3-contact","displayValue":"Contact us","label":"Contact Us","type":"DEEP_LINK"},
],
}]
how save imageGallery data in database
Article usefull
ReplyDeletewhere to store the .json file ?
ReplyDeleteAnywhere you like, though you don't need to store the file to read it and insert into database.
Deleteotherwise...
// define your folder where you want to save file
$filefolder = 'absolute_path/file_folder_name/';
// Get the file from its source
$file = file_get_contents('https://file.address.com');
// save file to your predefined folder
file_put_contents($filefolder .'/name_your_file_what_you_want.json', $file);
can any help to get data form json api to sql
ReplyDeleteHey! Only the db connectivity features will change, rest are the same. Maybe I can write tutorial on that in near future.
DeleteI'm using your method above, but I need some help with filtering out parts of an json file.
ReplyDeleteI get the json from https://redisq.zkillboard.com/listen.php and have no problem with printing out everything until I get to "attackers", I need to filter out a certain corporation and the attacker who got the "finalBlow"
With attackers, there can be as few as 1 or as many as 200, but I don't seem to be able to loop through the attackers to get the corporation > id or final blow.
Hey it's difficult to help without seeing json. But as for filtering json rows, you can simply loop through them, check for the specific key value and perform transactions as per your choice. Check this link, it deals with importing multiple json records into mysql. http://www.kodingmadesimple.com/2015/10/insert-multiple-json-data-into-mysql-database-php.html
DeleteHi Valli, I have a program polling a temperature sensor and would like to store that into mysql.
ReplyDeleteCleanest output is the json string:
{"time" : "2017-08-17 15:53:20", "model" : "Acurite Sensor", "id" : 101, "battery" : "OK", "temperature_F" : 121.460}
It is a continued flow of data that comes about every 50 seconds. Have been googling the last few days and so far your explanation is the most understandable for a beginning hobbyist.
With your explanation I would be able without a problem to fill the database from a file. But my challenge is to grasp the output flow and put it without storing to file into the database.
Maybe It is too much to describe but if you would be able to point me in a direction where to look would be a great help.