Hi, we'll see how to upload multiple files using jQuery, AJAX and PHP in this tutorial. To upload multiple file in php we are going to use an awesome jquery file upload plug-in from hayageek.com. It is a drag and drop file upload plug-in that supports multiple file uploads at once along with progress bars. In HTML 5 drag and drop is part of the standard and it comes with inbuilt drag and drop api to support drag and drop feature in web projects.
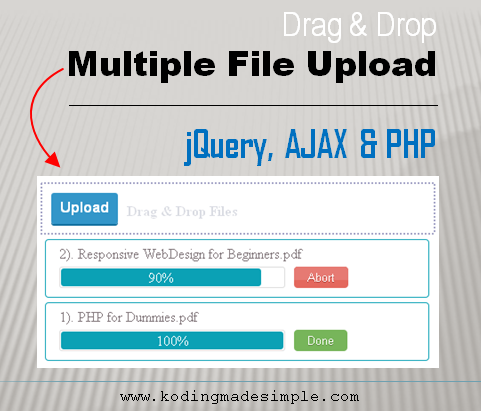
This file upload plug-in creates an ajax form on go to upload files and comes with various options for us to customize the way it behaves like showing progress bar, cancelling upload in the middle, auto form submission, restrict the number of files and types of files to upload etc. It depends on Ajax Form Plug-in to automatically generate ajax form for uploading files without page refresh.
You can either drag and drop the files in the form or select them with the upload button.
Implementing jQuery Multiple File Upload with Progress Bar
First download the file upload plug-in and extract its contents to your working folder. Then create a folder named 'uploads' to hold all the uploaded files.
Next create a HTML file 'index.html' which serves as an interface to upload files.
1. Load the required css and javascript files. Since it is built over jquery library we have to include jquery before the other js file.
<link href="/path/to/uploadfile.min.css" rel="stylesheet"> <script src="/path/to/jquery-1.10.2.js"></script> <script src="/path/to/jquery.uploadfile.min.js"></script>
2. Next create a <div>
block with an id 'multiple_file_uploader' which acts as a placeholder for the ajax form.
<div id="multiple_file_uploader">Upload</div>
3. Next add the java script code to initialize the plug in and create ajax form in the place of <div>
block to upload files.
$(document).ready(function() { $("#multiple_file_uploader").uploadFile({ fileName : "myfile", url : "upload.php", multiple : true, maxFileCount : 5, allowedTypes : "jpg,png,gif", showProgress : true }); });
When you open the html file in the browser you will get a file upload form with upload button like this.
![]() |
File Upload AJAX Form |
The uploadFile()
API creates ajax form and uploads the files to server. This method takes up various options as parameters to customize its behavior.
Upload File Options
- fileName - Name of the file input.
- url - PHP file name that should be executed when the form is submitted.
- multiple - Set as true for multiple file uploading.
- maxFileCount - The maximum number of files allowed to be uploaded at a time.
- showProgress - Set as true to show the ongoing progress bar.
- allowedTypes - Comma (,) separated file extensions that are allowed to be uploaded. For example if you want to allow only text or pdf files use it like "txt,pdf".
Here is the complete code for the 'index.html' file.
index.html
<!DOCTYPE html> <html> <head> <title>Drag and Drop Multiple File Upload | PHP Ajax and jQuery</title> <link href="/path/to/uploadfile.min.css" rel="stylesheet"> <script src="/path/to/jquery-1.10.2.js"></script> <script src="/path/to/jquery.uploadfile.min.js"></script> </head> <body> <div id="multiple_file_uploader">Upload</div> <script> $(document).ready(function() { $("#multiple_file_uploader").uploadFile({ fileName : "myfile", url : "upload.php", multiple : true, maxFileCount : 5, allowedTypes : "jpg,png,gif", showProgress : true }); }); </script> </body> </html>
PHP Script to Upload Multiple Files
Next we have to create the php file 'upload.php' which we have passed using the 'url' parameter. Once the file uploading ajax form is submitted, this php script will be executed where the actual file uploading process takes place.
upload.php
<?php // set folder to upload files $output_dir = "uploads/"; if(isset($_FILES["myfile"])) { $ret = array(); $error = $_FILES["myfile"]["error"]; // upload single file if(!is_array($_FILES["myfile"]["name"])) //single file { $fileName = $_FILES["myfile"]["name"]; move_uploaded_file($_FILES["myfile"]["tmp_name"],$output_dir.$fileName); $ret[]= $fileName; } else { // Handle Multiple files $fileCount = count($_FILES["myfile"]["name"]); for($i=0; $i $fileCount; $i++) { $fileName = $_FILES["myfile"]["name"][$i]; move_uploaded_file($_FILES["myfile"]["tmp_name"][$i],$output_dir.$fileName); $ret[]= $fileName; } } // output file names as comma seperated strings to display status echo json_encode($ret); } ?>
The $_FILES["myfile"]["error"] will return error messages if there is any and displayed below the file upload form like this.

Read this article to learn how file upload process works in php.
All the selected files will be uploaded to the 'uploads' directory. And progress bars will be shown at the bottom of the forms for each file along with an 'Abort' button. Once the uploading process is completed it shows 'Done' button at the end of the progress bar. This is a very user friendly feature available in this plug-in.
![]() |
File Upload Progress Bar with Abort and Done Buttons |
If you don't want the abort and done buttons to show in the progress bar, then set them to false like this,
showAbort : false showDone : falseRead:
- How to AutoComplete Textbox from MySQL Database using PHP & jQuery
- How to Add Slider Input control with Textbox in HTML Form using jQuery
That was all about drag and drop multiple file uploading process using jquery, ajax and php. I hope you like this tutorial. Please don't forget to share it on your social circle.
Hi,
ReplyDeletehow to insert the photos into the database with mysql.Please give me ideas.
Thankyou
It's simple. After the <?php open you mysql connection using:
ReplyDelete$conn = mysql_connect('localhost','root','') or die("Error connecting to host".mysql_error()); // mysql connection
After the move_uploaded_file() function add the code below
mysql_select_db("your_db_name",$conn); // connecting to db
$sql = "INSERT INTO `image` (name) VALUES ('$fileName')" // mysql insert statement
mysql_query($sql); // mysql query execution
It will be sent to the database and stored on the table call image. Hope this helps.
This plugin can send only max 2MB files. I dont get any errors, it's just doesn't work. How change this?
ReplyDeleteThis plugin can send only max 2MB files. How to change it? I've no errors.
ReplyDelete