Hi! In this post, let's see how to use PHPMailer to send email from PHP. PHPMailer is an amazing php library to send emails quickly. We all know the generic PHP's mail() function helps you send emails. But on the downside, it requires local mail server to send them. And also the setup and configuration is not that easy. But that's not the case with PHPMailer. The library supports SMTP protocol and allows easy authentication over SSL and TSL. It allows you to display error messages in more than 40 languages in case there is an error when sending emails.
PHPMailer uses the real valid email address to send mails. Hence you have to setup one if you don't have it. Here, let's see how to use Gmail SMTP to send email via PHPMailer.
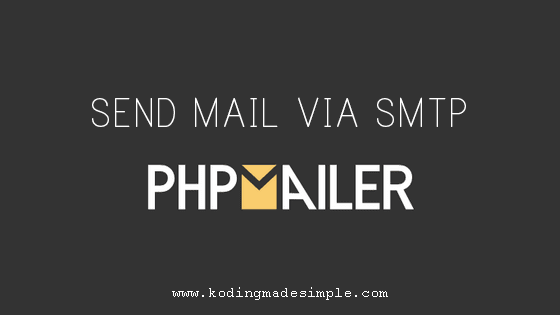
Sending Email via Gmail SMTP using PHPMailer:
SMTP is basically a mail protocol that sends a request to the mail server and after verification sends it to the mail server of the recipient.
To send an email via smtp, you need the authentication from the other host. So if you want to use gmail, you must provide the following details for authentication.
- Host - gmail smtp server
- Port - smtp port number
- Username - gmail address
- Password - gmail a/c password
First you need to download and extract the 'PHPMailer' folder to your application root. If you use composer (which is recommended), simply including the 'vendor/autoload.php' file in your php script will do. Otherwise, you must load all the required class files manually as I do in the following example.
PHPMailer Script to Send Mail:
<?php use PHPMailer\PHPMailer\PHPMailer; use PHPMailer\PHPMailer\Exception; require 'PHPMailer/src/Exception.php'; require 'PHPMailer/src/PHPMailer.php'; require 'PHPMailer/src/SMTP.php'; $mail = new PHPMailer; // smtp settings $mail->isSMTP(); $mail->SMTPDebug = 2; $mail->SMTPAuth = true; $mail->Host = 'smtp.gmail.com'; $mail->Port = 587; $mail->SMTPSecure = 'tsl'; $mail->Username = 'myemailaddress@gmail.com'; // change this to yours $mail->Password = '********'; // change this to yours // set from & to email address $mail->setFrom('myemailaddress@gmail.com', 'KMS'); // change this to yours $mail->addReplyTo('myemailaddress@gmail.com', 'KMS'); // change this to yours $mail->addAddress('john.doe@gmail.com'); // mail content $mail->isHTML(true); $mail->Subject = 'This is the subject'; $mail->Body = '<h1>Test Mail!</h1><p>This is the body of the message.</p>'; // send email if($mail->send()){ echo 'Message has been sent successfully!'; } else { echo 'Message could not be sent. Mailer Error: ' . $mail->ErrorInfo; } ?>
This php script send a simple html email to the recipient's email address. Note that we have used isHTML(true)
to set the mail format to html.
The first two lines of the script are used to import PHPMailer classes into the global namespace. Make sure they remain at the very top of your script and not within a function.
Sending Mail to Mulitiple Email-IDs:
To send an email to multiple email addresses, add the recipients one by one with the addAddress()
function.
$mail->addAddress('user1@example.com', 'user 1'); $mail->addAddress('user2@example.com', 'user 2'); $mail->addAddress('user3@example.com', 'user 3');
Including CC and BCC:
To send 'CC' and 'BCC', use addCC()
and addBCC()
functions.
$mail->addCC('cc_address@example.com'); $mail->addBCC('bcc_address@example.com');
Sending Mail with Attachments:
Use the addAttachment()
function to add attachments to the mails.
// attachment $mail->addAttachment('files/file1.tar.gz'); // add optional name to the attachment file $mail->addAttachment('images/image1.png', 'newimage.png');
Some may face problems while sending emails with gmail smtp. This is due to Google blocking the authentication from apps.
Just log in to your Google account, go to 'Sign-in & security' > 'Connected apps & sites' and turn on 'Allow less secure apps' option.
You may also face problem if you have enabled two-step verification on your account. Either disable the feature or create a unique password for signing in through apps.
Read Also:- Simple Contact Form to Send Email with PHP & Bootstrap
- How to Auto Complete Textbox in PHP, MySQL and jQuery
Following the above procedure, you can easily send emails through smtp with the phpmailer class. The same applies to any other third-party mail servers. You have to change the smtp host, username and password accordingly. I hope you find this post useful. Please share it on social media if you like it.
No comments:
Post a Comment