Bootstrap Login Form Tutorial: This post explains how to create simple bootstrap login form with validation using PHP & MySQL. Signup and Login pages are two core modules required for any member-ship based websites and with bootstrap you can create responsive login page effortlessly. Here first we'll design the login form with bootstrap, css & html. Then use php script to complete the background process involved in user login.
Simple Bootstrap Login Form Example:
Here goes our simple bootstrap login system. Since bootstrap is one of the front-end tools, we can design a nice-looking login form with it, but need some sort of server scripting to make the form workable. For this tutorial I'm going to use PHP for server-side script and MySQL for database.
Create Database:
Run these below sql commands in MySQL to create the database required for our bootstrap login demo.
CREATE DATABASE IF NOT EXISTS `bt_demo`; USE `bt_demo`; CREATE TABLE IF NOT EXISTS `users` ( `id` int(9) NOT NULL AUTO_INCREMENT, `name` varchar(30) NOT NULL, `email` varchar(60) NOT NULL, `password` varchar(40) NOT NULL, PRIMARY KEY (`id`), UNIQUE KEY `email` (`email`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=2 ; INSERT INTO `users` (`id`, `name`, `email`, `password`) VALUES ('1', 'admin', 'admin@mydomain.com', '21232f297a57a5a743894a0e4a801fc3');
Next we need to build user interface for login. Come, let's create an html login form using bootstrap css.
index.php
<!DOCTYPE html> <html> <head> <title>Bootstrap Login Form Example using PHP & MySQL</title> <meta content="width=device-width, initial-scale=1.0" name="viewport" > <link rel="stylesheet" href="bootstrap.css" type="text/css" /> <style type="text/css"> #login-form .input-group, #login-form .form-group { margin-top: 30px; } #login-form .btn-default { background-color: #EEE; } .brand { color: #CCC; } </style> </head> <body> <div class="container" style="margin-top: 30px;"> <div class="col-sm-6 col-sm-offset-3"> <div class="panel panel-default"> <div class="panel-body"> <form id="login-form" method="post" action="login.php" role="form"> <legend>Bootstrap Login</legend> <?php if (isset($_GET['err'])) { ?> <div class="alert alert-danger text-center"><?php echo "Login failed! Invalid email-id or password!"; ?></div> <?php } ?> <div class="input-group"> <span class="input-group-addon"><i class="glyphicon glyphicon-envelope"></i></span> <input type="text" name="email" placeholder="Enter your email-id" required class="form-control" /> </div> <div class="input-group"> <span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span> <input type="password" name="password" placeholder="Password" required class="form-control" /> </div> <div class="form-group"> <input type="submit" name="submit" value="Login" class="btn btn-primary btn-block" /> </div> <div class="form-group"> <hr/> <div class="col-sm-6" style="padding: 0;">New user? <a href="#">Sign Up here</a></div> <div class="col-sm-6 text-right brand" style="padding: 0;">kodingmadesimple.com</div> </div> </form> </div> </div> </div> </div> </body> </html>
In the above file, I have tweaked & customized bootstrap css a little to make the login form look like this.

Also have used some php code <?php if (isset($_GET['err'])) { ?> ... <?php } ?>
to display error message if user authentication fails.
Next we'll need a php script where the actual authentication process takes place. The script should receive user login details, check them against 'users' table from database and authenticate user if there is a match for the provided email and password else display error message in the login form. Here's the php file for user authentication.
login.php
<?php // connect to mysql database $con = mysqli_connect("localhost", "root", "", "bt_demo") or die("Error " . mysqli_error($con)); // check if form is submitted if (isset($_POST['submit'])) { $uemail = mysqli_real_escape_string($con, $_POST['email']); $upwd = mysqli_real_escape_string($con, $_POST['password']); $result = mysqli_query($con, "SELECT * FROM users WHERE email = '" . $uemail. "' and password = '" . md5($upwd) . "'"); if (mysqli_num_rows($result) > 0) { // login successful - start user session, store data in session & redirect user to index or dashboard page as per your need $row = mysqli_fetch_array($result); session_start(); $_SESSION['user_id'] = $row['id']; $_SESSION['user_name'] = $row['name']; header("Location: profile.php"); //change this } else { // login failed header("Location: index.php?err=true"); } } ?>
If the user enters valid email & password, then the script start session, stores user id & name as session data for future reference and redirect user to his/her profile page. On the other hand if login fails the script redirects to index.php and display invalid login message to the user.
Note: The scope of this article is just about login process and creating profile.php is not dealt here. If you want to read about complete user login and signup system then check here.
Now we have all the required files in place. Run index.php and the bootstrap login form prompts user to enter email & password field. As for validating form fields we have included simple HTML5 form validation here.
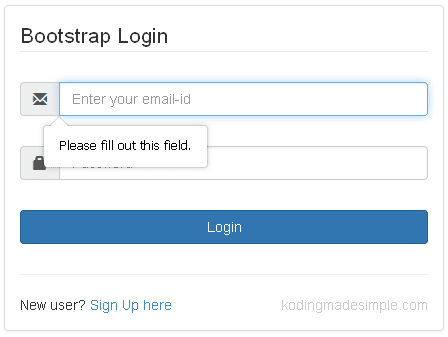
To test the login process, enter 'admin@mydomain.com' for email and 'admin' for password and click login. These are the valid login credentials present in the 'users' table. Also make sure to create profile.php file or redirect to some other page that exists for the script to work properly.
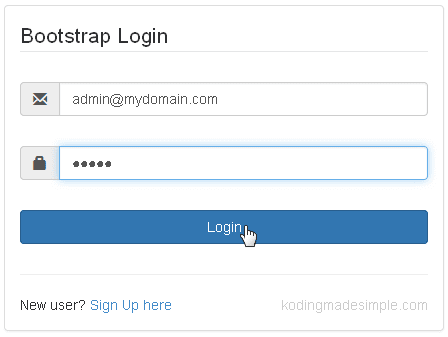
As for invalid login, enter some wrong data and click login button and an error message will be shown above the form like this,

Related: Workable Bootstrap Contact us Form Example
So that explains about creating login page in bootstrap & php. I hope you enjoyed this simple bootstrap login form tutorial. I intend to share a post about bootstrap signup form soon. Like us on Facebook and stay tuned:)
Thank you, very useful code
ReplyDelete